
NOT FINISHED YET!!! My first try to get a self built fully working Quadrocopter based on an mbed, a self built frame and some other more or less cheap parts.
LED.cpp
00001 #include "LED.h" 00002 #include "mbed.h" 00003 00004 LED::LED() : Led(LED1, LED2, LED3, LED4){ 00005 roller = 0; 00006 } 00007 00008 void LED::shownumber(int number) { 00009 Led = number; 00010 } 00011 00012 void LED::ride(int times = 1) { 00013 Led = 0; 00014 for (int j = 0; j < times; j++) { 00015 for(int i=0; i < 4; i++) { 00016 Led = 1 << i; 00017 wait(0.2); 00018 } 00019 } 00020 Led = 0; 00021 } 00022 00023 void LED::roll(int times = 1) { 00024 Led = 0; 00025 for (int j = 0; j < (times*2); j++) { 00026 for(int roller = 1; roller <= 4; roller++) { 00027 tilt(roller); 00028 wait(0.1); 00029 } 00030 } 00031 roller = 0; 00032 Led = 0; 00033 } 00034 00035 void LED::rollnext() { 00036 if (roller >= 4) 00037 roller = 0; 00038 roller++; 00039 tilt(roller); 00040 } 00041 00042 void LED::tilt(int index) { 00043 Led = Led ^ (1 << (index-1)); //XOR 00044 } 00045 00046 void LED::set(int index) { 00047 Led = Led | (1 << (index-1)); //OR 00048 } 00049 00050 void LED::reset(int index) { 00051 Led = Led & ~(1 << (index-1)); //OR 00052 } 00053 00054 int LED::check(int index) { 00055 return Led & (1 << (index-1)); 00056 } 00057 00058 void LED::operator=(int value) { 00059 Led = value; 00060 }
Generated on Tue Jul 12 2022 20:54:01 by
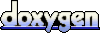