
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
raw.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_RAW_H__ 00033 #define __LWIP_RAW_H__ 00034 00035 #include "lwip/opt.h" 00036 00037 #if LWIP_RAW /* don't build if not configured for use in lwipopts.h */ 00038 00039 #include "lwip/pbuf.h" 00040 #include "lwip/def.h" 00041 #include "lwip/ip.h" 00042 #include "lwip/ip_addr.h" 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 struct raw_pcb; 00049 00050 /** Function prototype for raw pcb receive callback functions. 00051 * @param arg user supplied argument (raw_pcb.recv_arg) 00052 * @param pcb the raw_pcb which received data 00053 * @param p the packet buffer that was received 00054 * @param addr the remote IP address from which the packet was received 00055 * @return 1 if the packet was 'eaten' (aka. deleted), 00056 * 0 if the packet lives on 00057 * If returning 1, the callback is responsible for freeing the pbuf 00058 * if it's not used any more. 00059 */ 00060 typedef u8_t (*raw_recv_fn)(void *arg, struct raw_pcb *pcb, struct pbuf *p, 00061 ip_addr_t *addr); 00062 00063 struct raw_pcb { 00064 /* Common members of all PCB types */ 00065 IP_PCB; 00066 00067 struct raw_pcb *next; 00068 00069 u8_t protocol; 00070 00071 /** receive callback function */ 00072 raw_recv_fn recv; 00073 /* user-supplied argument for the recv callback */ 00074 void *recv_arg; 00075 }; 00076 00077 /* The following functions is the application layer interface to the 00078 RAW code. */ 00079 struct raw_pcb * raw_new (u8_t proto); 00080 void raw_remove (struct raw_pcb *pcb); 00081 err_t raw_bind (struct raw_pcb *pcb, ip_addr_t *ipaddr); 00082 err_t raw_connect (struct raw_pcb *pcb, ip_addr_t *ipaddr); 00083 00084 void raw_recv (struct raw_pcb *pcb, raw_recv_fn recv, void *recv_arg); 00085 err_t raw_sendto (struct raw_pcb *pcb, struct pbuf *p, ip_addr_t *ipaddr); 00086 err_t raw_send (struct raw_pcb *pcb, struct pbuf *p); 00087 00088 /* The following functions are the lower layer interface to RAW. */ 00089 u8_t raw_input (struct pbuf *p, struct netif *inp); 00090 #define raw_init() /* Compatibility define, not init needed. */ 00091 00092 #ifdef __cplusplus 00093 } 00094 #endif 00095 00096 #endif /* LWIP_RAW */ 00097 00098 #endif /* __LWIP_RAW_H__ */
Generated on Tue Jul 12 2022 21:10:26 by
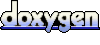