
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
memp_std.h
00001 /* 00002 * SETUP: Make sure we define everything we will need. 00003 * 00004 * We have create three types of pools: 00005 * 1) MEMPOOL - standard pools 00006 * 2) MALLOC_MEMPOOL - to be used by mem_malloc in mem.c 00007 * 3) PBUF_MEMPOOL - a mempool of pbuf's, so include space for the pbuf struct 00008 * 00009 * If the include'r doesn't require any special treatment of each of the types 00010 * above, then will declare #2 & #3 to be just standard mempools. 00011 */ 00012 #ifndef LWIP_MALLOC_MEMPOOL 00013 /* This treats "malloc pools" just like any other pool. 00014 The pools are a little bigger to provide 'size' as the amount of user data. */ 00015 #define LWIP_MALLOC_MEMPOOL(num, size) LWIP_MEMPOOL(POOL_##size, num, (size + sizeof(struct memp_malloc_helper)), "MALLOC_"#size) 00016 #define LWIP_MALLOC_MEMPOOL_START 00017 #define LWIP_MALLOC_MEMPOOL_END 00018 #endif /* LWIP_MALLOC_MEMPOOL */ 00019 00020 #ifndef LWIP_PBUF_MEMPOOL 00021 /* This treats "pbuf pools" just like any other pool. 00022 * Allocates buffers for a pbuf struct AND a payload size */ 00023 #define LWIP_PBUF_MEMPOOL(name, num, payload, desc) LWIP_MEMPOOL(name, num, (MEMP_ALIGN_SIZE(sizeof(struct pbuf)) + MEMP_ALIGN_SIZE(payload)), desc) 00024 #endif /* LWIP_PBUF_MEMPOOL */ 00025 00026 00027 /* 00028 * A list of internal pools used by LWIP. 00029 * 00030 * LWIP_MEMPOOL(pool_name, number_elements, element_size, pool_description) 00031 * creates a pool name MEMP_pool_name. description is used in stats.c 00032 */ 00033 #if LWIP_RAW 00034 LWIP_MEMPOOL(RAW_PCB, MEMP_NUM_RAW_PCB, sizeof(struct raw_pcb), "RAW_PCB") 00035 #endif /* LWIP_RAW */ 00036 00037 #if LWIP_UDP 00038 LWIP_MEMPOOL(UDP_PCB, MEMP_NUM_UDP_PCB, sizeof(struct udp_pcb), "UDP_PCB") 00039 #endif /* LWIP_UDP */ 00040 00041 #if LWIP_TCP 00042 LWIP_MEMPOOL(TCP_PCB, MEMP_NUM_TCP_PCB, sizeof(struct tcp_pcb), "TCP_PCB") 00043 LWIP_MEMPOOL(TCP_PCB_LISTEN, MEMP_NUM_TCP_PCB_LISTEN, sizeof(struct tcp_pcb_listen), "TCP_PCB_LISTEN") 00044 LWIP_MEMPOOL(TCP_SEG, MEMP_NUM_TCP_SEG, sizeof(struct tcp_seg), "TCP_SEG") 00045 #endif /* LWIP_TCP */ 00046 00047 #if IP_REASSEMBLY 00048 LWIP_MEMPOOL(REASSDATA, MEMP_NUM_REASSDATA, sizeof(struct ip_reassdata), "REASSDATA") 00049 #endif /* IP_REASSEMBLY */ 00050 00051 #if LWIP_NETCONN 00052 LWIP_MEMPOOL(NETBUF, MEMP_NUM_NETBUF, sizeof(struct netbuf), "NETBUF") 00053 LWIP_MEMPOOL(NETCONN, MEMP_NUM_NETCONN, sizeof(struct netconn), "NETCONN") 00054 #endif /* LWIP_NETCONN */ 00055 00056 #if NO_SYS==0 00057 LWIP_MEMPOOL(TCPIP_MSG_API, MEMP_NUM_TCPIP_MSG_API, sizeof(struct tcpip_msg), "TCPIP_MSG_API") 00058 LWIP_MEMPOOL(TCPIP_MSG_INPKT,MEMP_NUM_TCPIP_MSG_INPKT, sizeof(struct tcpip_msg), "TCPIP_MSG_INPKT") 00059 #endif /* NO_SYS==0 */ 00060 00061 #if ARP_QUEUEING 00062 LWIP_MEMPOOL(ARP_QUEUE, MEMP_NUM_ARP_QUEUE, sizeof(struct etharp_q_entry), "ARP_QUEUE") 00063 #endif /* ARP_QUEUEING */ 00064 00065 #if LWIP_IGMP 00066 LWIP_MEMPOOL(IGMP_GROUP, MEMP_NUM_IGMP_GROUP, sizeof(struct igmp_group), "IGMP_GROUP") 00067 #endif /* LWIP_IGMP */ 00068 00069 LWIP_MEMPOOL(SYS_TIMEOUT, MEMP_NUM_SYS_TIMEOUT, sizeof(struct sys_timeo), "SYS_TIMEOUT") 00070 00071 #if LWIP_SNMP 00072 LWIP_MEMPOOL(SNMP_ROOTNODE, MEMP_NUM_SNMP_ROOTNODE, sizeof(struct mib_list_rootnode), "SNMP_ROOTNODE") 00073 LWIP_MEMPOOL(SNMP_NODE, MEMP_NUM_SNMP_NODE, sizeof(struct mib_list_node), "SNMP_NODE") 00074 LWIP_MEMPOOL(SNMP_VARBIND, MEMP_NUM_SNMP_VARBIND, sizeof(struct snmp_varbind), "SNMP_VARBIND") 00075 LWIP_MEMPOOL(SNMP_VALUE, MEMP_NUM_SNMP_VALUE, SNMP_MAX_VALUE_SIZE, "SNMP_VALUE") 00076 #endif /* LWIP_SNMP */ 00077 #if LWIP_DNS && LWIP_SOCKET 00078 LWIP_MEMPOOL(NETDB, MEMP_NUM_NETDB, NETDB_ELEM_SIZE, "NETDB") 00079 #endif /* LWIP_DNS && LWIP_SOCKET */ 00080 00081 /* 00082 * A list of pools of pbuf's used by LWIP. 00083 * 00084 * LWIP_PBUF_MEMPOOL(pool_name, number_elements, pbuf_payload_size, pool_description) 00085 * creates a pool name MEMP_pool_name. description is used in stats.c 00086 * This allocates enough space for the pbuf struct and a payload. 00087 * (Example: pbuf_payload_size=0 allocates only size for the struct) 00088 */ 00089 LWIP_PBUF_MEMPOOL(PBUF, MEMP_NUM_PBUF, 0, "PBUF_REF/ROM") 00090 LWIP_PBUF_MEMPOOL(PBUF_POOL, PBUF_POOL_SIZE, PBUF_POOL_BUFSIZE, "PBUF_POOL") 00091 00092 00093 /* 00094 * Allow for user-defined pools; this must be explicitly set in lwipopts.h 00095 * since the default is to NOT look for lwippools.h 00096 */ 00097 #if MEMP_USE_CUSTOM_POOLS 00098 #include "lwippools.h" 00099 #endif /* MEMP_USE_CUSTOM_POOLS */ 00100 00101 /* 00102 * REQUIRED CLEANUP: Clear up so we don't get "multiply defined" error later 00103 * (#undef is ignored for something that is not defined) 00104 */ 00105 #undef LWIP_MEMPOOL 00106 #undef LWIP_MALLOC_MEMPOOL 00107 #undef LWIP_MALLOC_MEMPOOL_START 00108 #undef LWIP_MALLOC_MEMPOOL_END 00109 #undef LWIP_PBUF_MEMPOOL
Generated on Tue Jul 12 2022 21:10:25 by
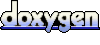