
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
dhcp.h
00001 /** @file 00002 */ 00003 00004 #ifndef __LWIP_DHCP_H__ 00005 #define __LWIP_DHCP_H__ 00006 00007 #include "lwip/opt.h" 00008 00009 #if LWIP_DHCP /* don't build if not configured for use in lwipopts.h */ 00010 00011 #include "lwip/netif.h" 00012 #include "lwip/udp.h" 00013 00014 #ifdef __cplusplus 00015 extern "C" { 00016 #endif 00017 00018 /** period (in seconds) of the application calling dhcp_coarse_tmr() */ 00019 #define DHCP_COARSE_TIMER_SECS 60 00020 /** period (in milliseconds) of the application calling dhcp_coarse_tmr() */ 00021 #define DHCP_COARSE_TIMER_MSECS (DHCP_COARSE_TIMER_SECS * 1000UL) 00022 /** period (in milliseconds) of the application calling dhcp_fine_tmr() */ 00023 #define DHCP_FINE_TIMER_MSECS 500 00024 00025 #define DHCP_CHADDR_LEN 16U 00026 #define DHCP_SNAME_LEN 64U 00027 #define DHCP_FILE_LEN 128U 00028 00029 struct dhcp 00030 { 00031 /** transaction identifier of last sent request */ 00032 u32_t xid; 00033 /** our connection to the DHCP server */ 00034 struct udp_pcb *pcb; 00035 /** incoming msg */ 00036 struct dhcp_msg *msg_in; 00037 /** current DHCP state machine state */ 00038 u8_t state; 00039 /** retries of current request */ 00040 u8_t tries; 00041 #if LWIP_DHCP_AUTOIP_COOP 00042 u8_t autoip_coop_state; 00043 #endif 00044 u8_t subnet_mask_given; 00045 00046 struct pbuf *p_out; /* pbuf of outcoming msg */ 00047 struct dhcp_msg *msg_out; /* outgoing msg */ 00048 u16_t options_out_len; /* outgoing msg options length */ 00049 u16_t request_timeout; /* #ticks with period DHCP_FINE_TIMER_SECS for request timeout */ 00050 u16_t t1_timeout; /* #ticks with period DHCP_COARSE_TIMER_SECS for renewal time */ 00051 u16_t t2_timeout; /* #ticks with period DHCP_COARSE_TIMER_SECS for rebind time */ 00052 ip_addr_t server_ip_addr; /* dhcp server address that offered this lease */ 00053 ip_addr_t offered_ip_addr; 00054 ip_addr_t offered_sn_mask; 00055 ip_addr_t offered_gw_addr; 00056 00057 u32_t offered_t0_lease; /* lease period (in seconds) */ 00058 u32_t offered_t1_renew; /* recommended renew time (usually 50% of lease period) */ 00059 u32_t offered_t2_rebind; /* recommended rebind time (usually 66% of lease period) */ 00060 /* @todo: LWIP_DHCP_BOOTP_FILE configuration option? 00061 integrate with possible TFTP-client for booting? */ 00062 #if LWIP_DHCP_BOOTP_FILE 00063 ip_addr_t offered_si_addr; 00064 char boot_file_name[DHCP_FILE_LEN]; 00065 #endif /* LWIP_DHCP_BOOTPFILE */ 00066 }; 00067 00068 /* MUST be compiled with "pack structs" or equivalent! */ 00069 #ifdef PACK_STRUCT_USE_INCLUDES 00070 # include "arch/bpstruct.h" 00071 #endif 00072 PACK_STRUCT_BEGIN 00073 /** minimum set of fields of any DHCP message */ 00074 struct dhcp_msg 00075 { 00076 PACK_STRUCT_FIELD(u8_t op); 00077 PACK_STRUCT_FIELD(u8_t htype); 00078 PACK_STRUCT_FIELD(u8_t hlen); 00079 PACK_STRUCT_FIELD(u8_t hops); 00080 PACK_STRUCT_FIELD(u32_t xid); 00081 PACK_STRUCT_FIELD(u16_t secs); 00082 PACK_STRUCT_FIELD(u16_t flags); 00083 PACK_STRUCT_FIELD(ip_addr_t ciaddr); 00084 PACK_STRUCT_FIELD(ip_addr_t yiaddr); 00085 PACK_STRUCT_FIELD(ip_addr_t siaddr); 00086 PACK_STRUCT_FIELD(ip_addr_t giaddr); 00087 PACK_STRUCT_FIELD(u8_t chaddr[DHCP_CHADDR_LEN]); 00088 PACK_STRUCT_FIELD(u8_t sname[DHCP_SNAME_LEN]); 00089 PACK_STRUCT_FIELD(u8_t file[DHCP_FILE_LEN]); 00090 PACK_STRUCT_FIELD(u32_t cookie); 00091 #define DHCP_MIN_OPTIONS_LEN 68U 00092 /** make sure user does not configure this too small */ 00093 #if ((defined(DHCP_OPTIONS_LEN)) && (DHCP_OPTIONS_LEN < DHCP_MIN_OPTIONS_LEN)) 00094 # undef DHCP_OPTIONS_LEN 00095 #endif 00096 /** allow this to be configured in lwipopts.h, but not too small */ 00097 #if (!defined(DHCP_OPTIONS_LEN)) 00098 /** set this to be sufficient for your options in outgoing DHCP msgs */ 00099 # define DHCP_OPTIONS_LEN DHCP_MIN_OPTIONS_LEN 00100 #endif 00101 PACK_STRUCT_FIELD(u8_t options[DHCP_OPTIONS_LEN]); 00102 } PACK_STRUCT_STRUCT; 00103 PACK_STRUCT_END 00104 #ifdef PACK_STRUCT_USE_INCLUDES 00105 # include "arch/epstruct.h" 00106 #endif 00107 00108 void dhcp_set_struct(struct netif *netif, struct dhcp *dhcp); 00109 /** start DHCP configuration */ 00110 err_t dhcp_start(struct netif *netif); 00111 /** enforce early lease renewal (not needed normally)*/ 00112 err_t dhcp_renew(struct netif *netif); 00113 /** release the DHCP lease, usually called before dhcp_stop()*/ 00114 err_t dhcp_release(struct netif *netif); 00115 /** stop DHCP configuration */ 00116 void dhcp_stop(struct netif *netif); 00117 /** inform server of our manual IP address */ 00118 void dhcp_inform(struct netif *netif); 00119 /** Handle a possible change in the network configuration */ 00120 void dhcp_network_changed(struct netif *netif); 00121 00122 /** if enabled, check whether the offered IP address is not in use, using ARP */ 00123 #if DHCP_DOES_ARP_CHECK 00124 void dhcp_arp_reply(struct netif *netif, ip_addr_t *addr); 00125 #endif 00126 00127 /** to be called every minute */ 00128 void dhcp_coarse_tmr(void); 00129 /** to be called every half second */ 00130 void dhcp_fine_tmr(void); 00131 00132 /** DHCP message item offsets and length */ 00133 #define DHCP_OP_OFS 0 00134 #define DHCP_HTYPE_OFS 1 00135 #define DHCP_HLEN_OFS 2 00136 #define DHCP_HOPS_OFS 3 00137 #define DHCP_XID_OFS 4 00138 #define DHCP_SECS_OFS 8 00139 #define DHCP_FLAGS_OFS 10 00140 #define DHCP_CIADDR_OFS 12 00141 #define DHCP_YIADDR_OFS 16 00142 #define DHCP_SIADDR_OFS 20 00143 #define DHCP_GIADDR_OFS 24 00144 #define DHCP_CHADDR_OFS 28 00145 #define DHCP_SNAME_OFS 44 00146 #define DHCP_FILE_OFS 108 00147 #define DHCP_MSG_LEN 236 00148 00149 #define DHCP_COOKIE_OFS DHCP_MSG_LEN 00150 #define DHCP_OPTIONS_OFS (DHCP_MSG_LEN + 4) 00151 00152 #define DHCP_CLIENT_PORT 68 00153 #define DHCP_SERVER_PORT 67 00154 00155 /** DHCP client states */ 00156 #define DHCP_OFF 0 00157 #define DHCP_REQUESTING 1 00158 #define DHCP_INIT 2 00159 #define DHCP_REBOOTING 3 00160 #define DHCP_REBINDING 4 00161 #define DHCP_RENEWING 5 00162 #define DHCP_SELECTING 6 00163 #define DHCP_INFORMING 7 00164 #define DHCP_CHECKING 8 00165 #define DHCP_PERMANENT 9 00166 #define DHCP_BOUND 10 00167 /** not yet implemented #define DHCP_RELEASING 11 */ 00168 #define DHCP_BACKING_OFF 12 00169 00170 /** AUTOIP cooperatation flags */ 00171 #define DHCP_AUTOIP_COOP_STATE_OFF 0 00172 #define DHCP_AUTOIP_COOP_STATE_ON 1 00173 00174 #define DHCP_BOOTREQUEST 1 00175 #define DHCP_BOOTREPLY 2 00176 00177 /** DHCP message types */ 00178 #define DHCP_DISCOVER 1 00179 #define DHCP_OFFER 2 00180 #define DHCP_REQUEST 3 00181 #define DHCP_DECLINE 4 00182 #define DHCP_ACK 5 00183 #define DHCP_NAK 6 00184 #define DHCP_RELEASE 7 00185 #define DHCP_INFORM 8 00186 00187 /** DHCP hardware type, currently only ethernet is supported */ 00188 #define DHCP_HTYPE_ETH 1 00189 00190 #define DHCP_MAGIC_COOKIE 0x63825363UL 00191 00192 /* This is a list of options for BOOTP and DHCP, see RFC 2132 for descriptions */ 00193 00194 /** BootP options */ 00195 #define DHCP_OPTION_PAD 0 00196 #define DHCP_OPTION_SUBNET_MASK 1 /* RFC 2132 3.3 */ 00197 #define DHCP_OPTION_ROUTER 3 00198 #define DHCP_OPTION_DNS_SERVER 6 00199 #define DHCP_OPTION_HOSTNAME 12 00200 #define DHCP_OPTION_IP_TTL 23 00201 #define DHCP_OPTION_MTU 26 00202 #define DHCP_OPTION_BROADCAST 28 00203 #define DHCP_OPTION_TCP_TTL 37 00204 #define DHCP_OPTION_END 255 00205 00206 /** DHCP options */ 00207 #define DHCP_OPTION_REQUESTED_IP 50 /* RFC 2132 9.1, requested IP address */ 00208 #define DHCP_OPTION_LEASE_TIME 51 /* RFC 2132 9.2, time in seconds, in 4 bytes */ 00209 #define DHCP_OPTION_OVERLOAD 52 /* RFC2132 9.3, use file and/or sname field for options */ 00210 00211 #define DHCP_OPTION_MESSAGE_TYPE 53 /* RFC 2132 9.6, important for DHCP */ 00212 #define DHCP_OPTION_MESSAGE_TYPE_LEN 1 00213 00214 #define DHCP_OPTION_SERVER_ID 54 /* RFC 2132 9.7, server IP address */ 00215 #define DHCP_OPTION_PARAMETER_REQUEST_LIST 55 /* RFC 2132 9.8, requested option types */ 00216 00217 #define DHCP_OPTION_MAX_MSG_SIZE 57 /* RFC 2132 9.10, message size accepted >= 576 */ 00218 #define DHCP_OPTION_MAX_MSG_SIZE_LEN 2 00219 00220 #define DHCP_OPTION_T1 58 /* T1 renewal time */ 00221 #define DHCP_OPTION_T2 59 /* T2 rebinding time */ 00222 #define DHCP_OPTION_US 60 00223 #define DHCP_OPTION_CLIENT_ID 61 00224 #define DHCP_OPTION_TFTP_SERVERNAME 66 00225 #define DHCP_OPTION_BOOTFILE 67 00226 00227 /** possible combinations of overloading the file and sname fields with options */ 00228 #define DHCP_OVERLOAD_NONE 0 00229 #define DHCP_OVERLOAD_FILE 1 00230 #define DHCP_OVERLOAD_SNAME 2 00231 #define DHCP_OVERLOAD_SNAME_FILE 3 00232 00233 #ifdef __cplusplus 00234 } 00235 #endif 00236 00237 #endif /* LWIP_DHCP */ 00238 00239 #endif /*__LWIP_DHCP_H__*/
Generated on Tue Jul 12 2022 21:10:25 by
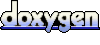