
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
cc.h
00001 /* 00002 * Author: Adam Dunkels <adam@sics.se> 00003 * 00004 */ 00005 #ifndef __LWIP_ARCH_CC_H__ 00006 #define __LWIP_ARCH_CC_H__ 00007 00008 #define LITTLE_ENDIAN 1234 00009 00010 #define BYTE_ORDER LITTLE_ENDIAN 00011 00012 typedef unsigned char u8_t; 00013 typedef signed char s8_t; 00014 typedef unsigned short u16_t; 00015 typedef signed short s16_t; 00016 typedef unsigned int u32_t; 00017 typedef signed int s32_t; 00018 typedef unsigned int mem_ptr_t; 00019 00020 #ifndef NULL 00021 #define NULL 0 00022 #endif 00023 00024 #ifndef TRUE 00025 #define TRUE 1 00026 #endif 00027 00028 #ifndef FALSE 00029 #define FALSE 0 00030 #endif 00031 00032 #ifndef DBG 00033 //#error 00034 #endif 00035 00036 #define LWIP_PLATFORM_DIAG(x) DBGFIXLF x 00037 #define LWIP_PLATFORM_ASSERT(x) DBG(x) 00038 00039 #define LWIP_PROVIDE_ERRNO 00040 00041 #define U16_F "hu" 00042 #define S16_F "hd" 00043 #define X16_F "hx" 00044 #define U32_F "lu" 00045 #define S32_F "ld" 00046 #define X32_F "lx" 00047 00048 #if 0 00049 /*Create compilation problems, and according to http://www.mail-archive.com/lwip-users@nongnu.org/msg06786.html, 00050 lwIP uses packed structures, so packing the field is not really a good idea ;) */ 00051 #define PACK_STRUCT_FIELD(x) __packed x 00052 #else 00053 #define PACK_STRUCT_FIELD(x) x 00054 #endif 00055 00056 #define PACK_STRUCT_STRUCT 00057 #define PACK_STRUCT_BEGIN __packed 00058 #define PACK_STRUCT_END 00059 00060 00061 #endif /* __LWIP_ARCH_CC_H__ */
Generated on Tue Jul 12 2022 21:10:24 by
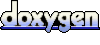