
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
autoip.h
00001 /** 00002 * @file 00003 * 00004 * AutoIP Automatic LinkLocal IP Configuration 00005 */ 00006 00007 /* 00008 * 00009 * Copyright (c) 2007 Dominik Spies <kontakt@dspies.de> 00010 * All rights reserved. 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. The name of the author may not be used to endorse or promote products 00021 * derived from this software without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00024 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00025 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00026 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00027 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00028 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00029 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00030 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00031 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00032 * OF SUCH DAMAGE. 00033 * 00034 * Author: Dominik Spies <kontakt@dspies.de> 00035 * 00036 * This is a AutoIP implementation for the lwIP TCP/IP stack. It aims to conform 00037 * with RFC 3927. 00038 * 00039 * 00040 * Please coordinate changes and requests with Dominik Spies 00041 * <kontakt@dspies.de> 00042 */ 00043 00044 #ifndef __LWIP_AUTOIP_H__ 00045 #define __LWIP_AUTOIP_H__ 00046 00047 #include "lwip/opt.h" 00048 00049 #if LWIP_AUTOIP /* don't build if not configured for use in lwipopts.h */ 00050 00051 #include "lwip/netif.h" 00052 #include "lwip/udp.h" 00053 #include "netif/etharp.h" 00054 00055 #ifdef __cplusplus 00056 extern "C" { 00057 #endif 00058 00059 /* AutoIP Timing */ 00060 #define AUTOIP_TMR_INTERVAL 100 00061 #define AUTOIP_TICKS_PER_SECOND (1000 / AUTOIP_TMR_INTERVAL) 00062 00063 /* RFC 3927 Constants */ 00064 #define PROBE_WAIT 1 /* second (initial random delay) */ 00065 #define PROBE_MIN 1 /* second (minimum delay till repeated probe) */ 00066 #define PROBE_MAX 2 /* seconds (maximum delay till repeated probe) */ 00067 #define PROBE_NUM 3 /* (number of probe packets) */ 00068 #define ANNOUNCE_NUM 2 /* (number of announcement packets) */ 00069 #define ANNOUNCE_INTERVAL 2 /* seconds (time between announcement packets) */ 00070 #define ANNOUNCE_WAIT 2 /* seconds (delay before announcing) */ 00071 #define MAX_CONFLICTS 10 /* (max conflicts before rate limiting) */ 00072 #define RATE_LIMIT_INTERVAL 60 /* seconds (delay between successive attempts) */ 00073 #define DEFEND_INTERVAL 10 /* seconds (min. wait between defensive ARPs) */ 00074 00075 /* AutoIP client states */ 00076 #define AUTOIP_STATE_OFF 0 00077 #define AUTOIP_STATE_PROBING 1 00078 #define AUTOIP_STATE_ANNOUNCING 2 00079 #define AUTOIP_STATE_BOUND 3 00080 00081 struct autoip 00082 { 00083 ip_addr_t llipaddr; /* the currently selected, probed, announced or used LL IP-Address */ 00084 u8_t state; /* current AutoIP state machine state */ 00085 u8_t sent_num; /* sent number of probes or announces, dependent on state */ 00086 u16_t ttw; /* ticks to wait, tick is AUTOIP_TMR_INTERVAL long */ 00087 u8_t lastconflict; /* ticks until a conflict can be solved by defending */ 00088 u8_t tried_llipaddr; /* total number of probed/used Link Local IP-Addresses */ 00089 }; 00090 00091 00092 /** Init srand, has to be called before entering mainloop */ 00093 void autoip_init(void); 00094 00095 /** Set a struct autoip allocated by the application to work with */ 00096 void autoip_set_struct(struct netif *netif, struct autoip *autoip); 00097 00098 /** Start AutoIP client */ 00099 err_t autoip_start(struct netif *netif); 00100 00101 /** Stop AutoIP client */ 00102 err_t autoip_stop(struct netif *netif); 00103 00104 /** Handles every incoming ARP Packet, called by etharp_arp_input */ 00105 void autoip_arp_reply(struct netif *netif, struct etharp_hdr *hdr); 00106 00107 /** Has to be called in loop every AUTOIP_TMR_INTERVAL milliseconds */ 00108 void autoip_tmr(void); 00109 00110 /** Handle a possible change in the network configuration */ 00111 void autoip_network_changed(struct netif *netif); 00112 00113 #ifdef __cplusplus 00114 } 00115 #endif 00116 00117 #endif /* LWIP_AUTOIP */ 00118 00119 #endif /* __LWIP_AUTOIP_H__ */
Generated on Tue Jul 12 2022 21:10:24 by
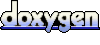