
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_wdt.h
00001 /***********************************************************************//** 00002 * @file : lpc17xx_wdt.h 00003 * @brief : Contains all macro definitions and function prototypes 00004 * support for WDT firmware library on LPC17xx 00005 * @version : 1.0 00006 * @date : 9. April. 2009 00007 * @author : HieuNguyen 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup WDT 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_WDT_H_ 00028 #define LPC17XX_WDT_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "cmsis.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 00041 /* Private Macros ------------------------------------------------------------- */ 00042 /** @defgroup WDT_Private_Macros 00043 * @{ 00044 */ 00045 00046 /** @defgroup WDT_REGISTER_BIT_DEFINITIONS 00047 * @{ 00048 */ 00049 /************************** WDT Control **************************/ 00050 /** WDT interrupt enable bit */ 00051 #define WDT_WDMOD_WDEN ((uint32_t)(1<<0)) 00052 /** WDT interrupt enable bit */ 00053 #define WDT_WDMOD_WDRESET ((uint32_t)(1<<1)) 00054 /** WDT time out flag bit */ 00055 #define WDT_WDMOD_WDTOF ((uint32_t)(1<<2)) 00056 /** WDT Time Out flag bit */ 00057 #define WDT_WDMOD_WDINT ((uint32_t)(1<<3)) 00058 /** WDT Mode */ 00059 #define WDT_WDMOD(n) ((uint32_t)(n<<1)) 00060 00061 /**************************** PRIVATE TYPES ***************************/ 00062 /** Define divider index for microsecond ( us ) */ 00063 #define WDT_US_INDEX ((uint32_t)(1000000)) 00064 /** WDT Time out minimum value */ 00065 #define WDT_TIMEOUT_MIN ((uint32_t)(0xFF)) 00066 /** WDT Time out maximum value */ 00067 #define WDT_TIMEOUT_MAX ((uint32_t)(0xFFFFFFFF)) 00068 00069 00070 /**************************** GLOBAL/PUBLIC TYPES ***************************/ 00071 /** Watchdog mode register mask */ 00072 #define WDT_WDMOD_MASK (uint8_t)(0x02) 00073 /** Watchdog timer constant register mask */ 00074 #define WDT_WDTC_MASK (uint8_t)(0xFFFFFFFF) 00075 /** Watchdog feed sequence register mask */ 00076 #define WDT_WDFEED_MASK (uint8_t)(0x000000FF) 00077 /** Watchdog timer value register mask */ 00078 #define WDT_WDCLKSEL_MASK (uint8_t)(0x03) 00079 /** Clock selected from internal RC */ 00080 #define WDT_WDCLKSEL_RC (uint8_t)(0x00) 00081 /** Clock selected from PCLK */ 00082 #define WDT_WDCLKSEL_PCLK (uint8_t)(0x01) 00083 /** Clock selected from external RTC */ 00084 #define WDT_WDCLKSEL_RTC (uint8_t)(0x02) 00085 00086 /** 00087 * @} 00088 */ 00089 00090 /** 00091 * @} 00092 */ 00093 00094 00095 /* Public Types --------------------------------------------------------------- */ 00096 /** @defgroup WDT_Public_Types 00097 * @{ 00098 */ 00099 00100 /** @brief Clock source option for WDT */ 00101 typedef enum { 00102 WDT_CLKSRC_IRC = 0, /*!< Clock source from Internal RC oscillator */ 00103 WDT_CLKSRC_PCLK = 1, /*!< Selects the APB peripheral clock (PCLK) */ 00104 WDT_CLKSRC_RTC = 2 /*!< Selects the RTC oscillator */ 00105 } WDT_CLK_OPT; 00106 #define PARAM_WDT_CLK_OPT(OPTION) ((OPTION ==WDT_CLKSRC_IRC)||(OPTION ==WDT_CLKSRC_PCLK)\ 00107 ||(OPTION ==WDT_CLKSRC_RTC)) 00108 /** @brief WDT operation mode */ 00109 typedef enum { 00110 WDT_MODE_INT_ONLY = 0, /*!< Use WDT to generate interrupt only */ 00111 WDT_MODE_RESET = 1 /*!< Use WDT to generate interrupt and reset MCU */ 00112 } WDT_MODE_OPT; 00113 #define PARAM_WDT_MODE_OPT(OPTION) ((OPTION ==WDT_MODE_INT_ONLY)||(OPTION ==WDT_MODE_RESET)) 00114 00115 /** 00116 * @} 00117 */ 00118 00119 00120 /* Public Functions ----------------------------------------------------------- */ 00121 /** @defgroup WDT_Public_Functions 00122 * @{ 00123 */ 00124 00125 void WDT_Init (uint32_t ClkSrc, uint32_t WDTMode); 00126 void WDT_Start(uint32_t TimeOut); 00127 void WDT_Feed (void); 00128 FlagStatus WDT_ReadTimeOutFlag (void); 00129 void WDT_ClrTimeOutFlag (void); 00130 void WDT_UpdateTimeOut ( uint32_t TimeOut); 00131 uint32_t WDT_GetCurrentCount(void); 00132 00133 /** 00134 * @} 00135 */ 00136 00137 #ifdef __cplusplus 00138 } 00139 #endif 00140 00141 #endif /* LPC17XX_WDT_H_ */ 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
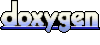