
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_timer.h
00001 /** 00002 * @file : lpc17xx_timer.h 00003 * @brief : Contains all functions support for Timer firmware library on LPC17xx 00004 * @version : 1.0 00005 * @date : 14. April. 2009 00006 * @author : HieuNguyen 00007 ************************************************************************** 00008 * Software that is described herein is for illustrative purposes only 00009 * which provides customers with programming information regarding the 00010 * products. This software is supplied "AS IS" without any warranties. 00011 * NXP Semiconductors assumes no responsibility or liability for the 00012 * use of the software, conveys no license or title under any patent, 00013 * copyright, or mask work right to the product. NXP Semiconductors 00014 * reserves the right to make changes in the software without 00015 * notification. NXP Semiconductors also make no representation or 00016 * warranty that such application will be suitable for the specified 00017 * use without further testing or modification. 00018 **********************************************************************/ 00019 00020 /* Peripheral group ----------------------------------------------------------- */ 00021 /** @defgroup TIM 00022 * @ingroup LPC1700CMSIS_FwLib_Drivers 00023 * @{ 00024 */ 00025 00026 #ifndef __LPC17XX_TIMER_H_ 00027 #define __LPC17XX_TIMER_H_ 00028 00029 /* Includes ------------------------------------------------------------------- */ 00030 #include "cmsis.h" 00031 #include "lpc_types.h" 00032 00033 00034 /* Private Macros ------------------------------------------------------------- */ 00035 /** @defgroup TIM_Private_Macros 00036 * @{ 00037 */ 00038 00039 /************************** TIMER/COUNTER Control **************************/ 00040 /** @defgroup TIM_REGISTER_BIT_DEFINITION 00041 * @{ 00042 */ 00043 00044 /********************************************************************** 00045 ** Interrupt information 00046 **********************************************************************/ 00047 /** Macro to clean interrupt pending */ 00048 #define TIM_IR_CLR(n) _BIT(n) 00049 00050 /********************************************************************** 00051 ** Timer interrupt register definitions 00052 **********************************************************************/ 00053 /** Macro for getting a timer match interrupt bit */ 00054 #define TIM_MATCH_INT(n) (_BIT(n & 0x0F)) 00055 /** Macro for getting a capture event interrupt bit */ 00056 #define TIM_CAP_INT(n) (_BIT(((n & 0x0F) + 4))) 00057 00058 /********************************************************************** 00059 * Timer control register definitions 00060 **********************************************************************/ 00061 /** Timer/counter enable bit */ 00062 #define TIM_ENABLE ((uint32_t)(1<<0)) 00063 /** Timer/counter reset bit */ 00064 #define TIM_RESET ((uint32_t)(1<<1)) 00065 /** Timer control bit mask */ 00066 #define TIM_TCR_MASKBIT ((uint32_t)(3)) 00067 00068 /********************************************************************** 00069 * Timer match control register definitions 00070 **********************************************************************/ 00071 /** Bit location for interrupt on MRx match, n = 0 to 3 */ 00072 #define TIM_INT_ON_MATCH(n) (_BIT((n * 3))) 00073 /** Bit location for reset on MRx match, n = 0 to 3 */ 00074 #define TIM_RESET_ON_MATCH(n) (_BIT(((n * 3) + 1))) 00075 /** Bit location for stop on MRx match, n = 0 to 3 */ 00076 #define TIM_STOP_ON_MATCH(n) (_BIT(((n * 3) + 2))) 00077 /** Timer Match control bit mask */ 00078 #define TIM_MCR_MASKBIT ((uint32_t)(0x0FFF)) 00079 /** Timer Match control bit mask for specific channel*/ 00080 #define TIM_MCR_CHANNEL_MASKBIT(n) ((uint32_t)(7<<n)) 00081 /********************************************************************** 00082 * Timer capture control register definitions 00083 **********************************************************************/ 00084 /** Bit location for CAP.n on CRx rising edge, n = 0 to 3 */ 00085 #define TIM_CAP_RISING(n) (_BIT((n * 3))) 00086 /** Bit location for CAP.n on CRx falling edge, n = 0 to 3 */ 00087 #define TIM_CAP_FALLING(n) (_BIT(((n * 3) + 1))) 00088 /** Bit location for CAP.n on CRx interrupt enable, n = 0 to 3 */ 00089 #define TIM_INT_ON_CAP(n) (_BIT(((n * 3) + 2))) 00090 /** Mask bit for rising and falling edge bit */ 00091 #define TIM_EDGE_MASK(n) (_SBF((n * 3), 0x03)) 00092 /** Timer capture control bit mask */ 00093 #define TIM_CCR_MASKBIT ((uint32_t)(0x3F)) 00094 /** Timer Capture control bit mask for specific channel*/ 00095 #define TIM_CCR_CHANNEL_MASKBIT(n) ((uint32_t)(7<<n)) 00096 00097 /********************************************************************** 00098 * Timer external match register definitions 00099 **********************************************************************/ 00100 /** Bit location for output state change of MAT.n when external match 00101 happens, n = 0 to 3 */ 00102 #define TIM_EM(n) _BIT(n) 00103 /** Output state change of MAT.n when external match happens: no change */ 00104 #define TIM_EM_NOTHING ((uint8_t)(0x0)) 00105 /** Output state change of MAT.n when external match happens: low */ 00106 #define TIM_EM_LOW ((uint8_t)(0x1)) 00107 /** Output state change of MAT.n when external match happens: high */ 00108 #define TIM_EM_HIGH ((uint8_t)(0x2)) 00109 /** Output state change of MAT.n when external match happens: toggle */ 00110 #define TIM_EM_TOGGLE ((uint8_t)(0x3)) 00111 /** Macro for setting for the MAT.n change state bits */ 00112 #define TIM_EM_SET(n,s) (_SBF(((n << 1) + 4), (s & 0x03))) 00113 /** Mask for the MAT.n change state bits */ 00114 #define TIM_EM_MASK(n) (_SBF(((n << 1) + 4), 0x03)) 00115 /** Timer external match bit mask */ 00116 #define TIM_EMR_MASKBIT 0x0FFF 00117 /********************************************************************** 00118 * Timer Count Control Register definitions 00119 **********************************************************************/ 00120 /** Mask to get the Counter/timer mode bits */ 00121 #define TIM_CTCR_MODE_MASK 0x3 00122 /** Mask to get the count input select bits */ 00123 #define TIM_CTCR_INPUT_MASK 0xC 00124 /** Timer Count control bit mask */ 00125 #define TIM_CTCR_MASKBIT 0xF 00126 #define TIM_COUNTER_MODE ((uint8_t)(1)) 00127 00128 /** 00129 * @} 00130 */ 00131 00132 /** 00133 * @} 00134 */ 00135 00136 00137 /* Public Types --------------------------------------------------------------- */ 00138 /** @defgroup TIM_Public_Types 00139 * @{ 00140 */ 00141 00142 /*********************************************************************** 00143 * Timer device enumeration 00144 **********************************************************************/ 00145 /** @brief interrupt type */ 00146 typedef enum 00147 { 00148 TIM_MR0_INT =0, /*!< interrupt for Match channel 0*/ 00149 TIM_MR1_INT =1, /*!< interrupt for Match channel 1*/ 00150 TIM_MR2_INT =2, /*!< interrupt for Match channel 2*/ 00151 TIM_MR3_INT =3, /*!< interrupt for Match channel 3*/ 00152 TIM_CR0_INT =4, /*!< interrupt for Capture channel 0*/ 00153 TIM_CR1_INT =5, /*!< interrupt for Capture channel 1*/ 00154 }TIM_INT_TYPE; 00155 #define PARAM_TIM_INT_TYPE(TYPE) ((TYPE ==TIM_MR0_INT)||(TYPE ==TIM_MR1_INT)\ 00156 ||(TYPE ==TIM_MR2_INT)||(TYPE ==TIM_MR3_INT)\ 00157 ||(TYPE ==TIM_CR0_INT)||(TYPE ==TIM_CR1_INT)) 00158 00159 /** @brief Timer/counter operating mode */ 00160 typedef enum 00161 { 00162 TIM_TIMER_MODE = 0, /*!< Timer mode */ 00163 TIM_COUNTER_RISING_MODE , /*!< Counter rising mode */ 00164 TIM_COUNTER_FALLING_MODE , /*!< Counter falling mode */ 00165 TIM_COUNTER_ANY_MODE /*!< Counter on both edges */ 00166 } TIM_MODE_OPT; 00167 #define PARAM_TIM_MODE_OPT(MODE) ((MODE == TIM_TIMER_MODE)||(MODE == TIM_COUNTER_RISING_MODE)\ 00168 || (MODE == TIM_COUNTER_RISING_MODE)||(MODE == TIM_COUNTER_RISING_MODE)) 00169 /** @brief Timer/Counter prescale option */ 00170 typedef enum 00171 { 00172 TIM_PRESCALE_TICKVAL = 0, /*!< Prescale in absolute value */ 00173 TIM_PRESCALE_USVAL /*!< Prescale in microsecond value */ 00174 } TIM_PRESCALE_OPT; 00175 #define PARAM_TIM_PRESCALE_OPT(OPT) ((OPT == TIM_PRESCALE_TICKVAL)||(OPT == TIM_PRESCALE_USVAL)) 00176 /** @brief Counter input option */ 00177 typedef enum 00178 { 00179 TIM_COUNTER_INCAP0 = 0, /*!< CAPn.0 input pin for TIMERn */ 00180 TIM_COUNTER_INCAP1 , /*!< CAPn.1 input pin for TIMERn */ 00181 } TIM_COUNTER_INPUT_OPT; 00182 #define PARAM_TIM_COUNTER_INPUT_OPT(OPT) ((OPT == TIM_COUNTER_INCAP0)||(OPT == TIM_COUNTER_INCAP1)) 00183 00184 /** @brief Timer/Counter external match option */ 00185 typedef enum 00186 { 00187 TIM_EXTMATCH_NOTHING = 0, /*!< Do nothing for external output pin if match */ 00188 TIM_EXTMATCH_LOW , /*!< Force external output pin to low if match */ 00189 TIM_EXTMATCH_HIGH , /*!< Force external output pin to high if match */ 00190 TIM_EXTMATCH_TOGGLE /*!< Toggle external output pin if match */ 00191 }TIM_EXTMATCH_OPT; 00192 #define PARAM_TIM_EXTMATCH_OPT(OPT) ((OPT == TIM_EXTMATCH_NOTHING)||(OPT == TIM_EXTMATCH_LOW)\ 00193 ||(OPT == TIM_EXTMATCH_HIGH)||(OPT == TIM_EXTMATCH_TOGGLE)) 00194 00195 /** @brief Timer/counter capture mode options */ 00196 typedef enum { 00197 TIM_CAPTURE_NONE = 0, /*!< No Capture */ 00198 TIM_CAPTURE_RISING , /*!< Rising capture mode */ 00199 TIM_CAPTURE_FALLING , /*!< Falling capture mode */ 00200 TIM_CAPTURE_ANY /*!< On both edges */ 00201 } TIM_CAP_MODE_OPT; 00202 00203 #define PARAM_TIM_CAP_MODE_OPT(OPT) ((OPT == TIM_CAPTURE_NONE)||(OPT == TIM_CAPTURE_RISING) \ 00204 ||(OPT == TIM_CAPTURE_FALLING)||(OPT == TIM_CAPTURE_ANY)) 00205 00206 /** @brief Configuration structure in TIMER mode */ 00207 typedef struct 00208 { 00209 00210 uint8_t PrescaleOption; /**< Timer Prescale option, should be: 00211 - TIM_PRESCALE_TICKVAL: Prescale in absolute value 00212 - TIM_PRESCALE_USVAL: Prescale in microsecond value 00213 */ 00214 uint8_t Reserved[3]; /**< Reserved */ 00215 uint32_t PrescaleValue; /**< Prescale value */ 00216 } TIM_TIMERCFG_Type; 00217 00218 /** @brief Configuration structure in COUNTER mode */ 00219 typedef struct { 00220 00221 uint8_t CounterOption; /**< Counter Option, should be: 00222 - TIM_COUNTER_INCAP0: CAPn.0 input pin for TIMERn 00223 - TIM_COUNTER_INCAP1: CAPn.1 input pin for TIMERn 00224 */ 00225 uint8_t CountInputSelect; 00226 uint8_t Reserved[2]; 00227 } TIM_COUNTERCFG_Type; 00228 00229 /** @brief Match channel configuration structure */ 00230 typedef struct { 00231 uint8_t MatchChannel; /**< Match channel, should be in range 00232 from 0..3 */ 00233 uint8_t IntOnMatch; /**< Interrupt On match, should be: 00234 - ENABLE: Enable this function. 00235 - DISABLE: Disable this function. 00236 */ 00237 uint8_t StopOnMatch; /**< Stop On match, should be: 00238 - ENABLE: Enable this function. 00239 - DISABLE: Disable this function. 00240 */ 00241 uint8_t ResetOnMatch; /**< Reset On match, should be: 00242 - ENABLE: Enable this function. 00243 - DISABLE: Disable this function. 00244 */ 00245 00246 uint8_t ExtMatchOutputType; /**< External Match Output type, should be: 00247 - 0: Do nothing for external output pin if match 00248 - 1: Force external output pin to low if match 00249 - 2: Force external output pin to high if match 00250 - 3: Toggle external output pin if match. 00251 */ 00252 uint8_t Reserved[3]; /** Reserved */ 00253 uint32_t MatchValue; /** Match value */ 00254 } TIM_MATCHCFG_Type; 00255 00256 00257 /** @brief Capture Input configuration structure */ 00258 typedef struct { 00259 uint8_t CaptureChannel; /**< Capture channel, should be in range 00260 from 0..1 */ 00261 uint8_t RisingEdge; /**< caption rising edge, should be: 00262 - ENABLE: Enable rising edge. 00263 - DISABLE: Disable this function. 00264 */ 00265 uint8_t FallingEdge; /**< caption falling edge, should be: 00266 - ENABLE: Enable falling edge. 00267 - DISABLE: Disable this function. 00268 */ 00269 uint8_t IntOnCaption; /**< Interrupt On caption, should be: 00270 - ENABLE: Enable interrupt function. 00271 - DISABLE: Disable this function. 00272 */ 00273 00274 } TIM_CAPTURECFG_Type; 00275 00276 /** 00277 * @} 00278 */ 00279 00280 00281 /* Public Macros -------------------------------------------------------------- */ 00282 /** @defgroup TIM_Public_Macros 00283 * @{ 00284 */ 00285 00286 /** Macro to determine if it is valid TIMER peripheral */ 00287 #define PARAM_TIMx(n) ((((uint32_t *)n)==((uint32_t *)LPC_TIM0)) || (((uint32_t *)n)==((uint32_t *)LPC_TIM1)) \ 00288 || (((uint32_t *)n)==((uint32_t *)LPC_TIM2)) || (((uint32_t *)n)==((uint32_t *)LPC_TIM3))) 00289 00290 /** 00291 * @} 00292 */ 00293 00294 00295 /* Public Functions ----------------------------------------------------------- */ 00296 /** @defgroup TIM_Public_Functions 00297 * @{ 00298 */ 00299 00300 FlagStatus TIM_GetIntStatus(LPC_TIM_TypeDef *TIMx, uint8_t IntFlag); 00301 FlagStatus TIM_GetIntCaptureStatus(LPC_TIM_TypeDef *TIMx, uint8_t IntFlag); 00302 void TIM_ClearIntPending(LPC_TIM_TypeDef *TIMx, uint8_t IntFlag); 00303 void TIM_ClearIntCapturePending(LPC_TIM_TypeDef *TIMx, uint8_t IntFlag); 00304 void TIM_Cmd(LPC_TIM_TypeDef *TIMx, FunctionalState NewState); 00305 void TIM_ResetCounter(LPC_TIM_TypeDef *TIMx); 00306 void TIM_Init(LPC_TIM_TypeDef *TIMx, uint8_t TimerCounterMode, void *TIM_ConfigStruct); 00307 void TIM_DeInit(LPC_TIM_TypeDef *TIMx); 00308 void TIM_ConfigStructInit(uint8_t TimerCounterMode, void *TIM_ConfigStruct); 00309 void TIM_ConfigMatch(LPC_TIM_TypeDef *TIMx, TIM_MATCHCFG_Type *TIM_MatchConfigStruct); 00310 void TIM_SetMatchExt(LPC_TIM_TypeDef *TIMx,TIM_EXTMATCH_OPT ext_match ); 00311 void TIM_ConfigCapture(LPC_TIM_TypeDef *TIMx, TIM_CAPTURECFG_Type *TIM_CaptureConfigStruct); 00312 uint32_t TIM_GetCaptureValue(LPC_TIM_TypeDef *TIMx, uint8_t CaptureChannel); 00313 00314 /** 00315 * @} 00316 */ 00317 00318 #endif /* __LPC17XX_TIMER_H_ */ 00319 00320 /** 00321 * @} 00322 */ 00323 00324 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
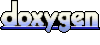