
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_timer.c
00001 /** 00002 * @file : lpc17xx_timer.c 00003 * @brief : Contains all functions support for Timer firmware library on LPC17xx 00004 * @version : 1.0 00005 * @date : 14. April. 2009 00006 * @author : HieuNguyen 00007 ************************************************************************** 00008 * Software that is described herein is for illustrative purposes only 00009 * which provides customers with programming information regarding the 00010 * products. This software is supplied "AS IS" without any warranties. 00011 * NXP Semiconductors assumes no responsibility or liability for the 00012 * use of the software, conveys no license or title under any patent, 00013 * copyright, or mask work right to the product. NXP Semiconductors 00014 * reserves the right to make changes in the software without 00015 * notification. NXP Semiconductors also make no representation or 00016 * warranty that such application will be suitable for the specified 00017 * use without further testing or modification. 00018 **********************************************************************/ 00019 00020 /* Peripheral group ----------------------------------------------------------- */ 00021 /** @addtogroup TIM 00022 * @{ 00023 */ 00024 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_timer.h" 00027 #include "lpc17xx_clkpwr.h" 00028 #include "lpc17xx_pinsel.h" 00029 00030 /* If this source file built with example, the LPC17xx FW library configuration 00031 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00032 * otherwise the default FW library configuration file must be included instead 00033 */ 00034 #ifdef __BUILD_WITH_EXAMPLE__ 00035 #include "lpc17xx_libcfg.h" 00036 #else 00037 #include "lpc17xx_libcfg_default.h" 00038 #endif /* __BUILD_WITH_EXAMPLE__ */ 00039 00040 #ifdef _TIM 00041 00042 /* Private Functions ---------------------------------------------------------- */ 00043 /** @defgroup TIM_Private_Functions 00044 * @{ 00045 */ 00046 00047 uint32_t TIM_GetPClock (uint32_t timernum); 00048 uint32_t TIM_ConverUSecToVal (uint32_t timernum, uint32_t usec); 00049 uint32_t TIM_ConverPtrToTimeNum (LPC_TIM_TypeDef *TIMx); 00050 00051 00052 /*********************************************************************//** 00053 * @brief Get peripheral clock of each timer controller 00054 * @param[in] timernum Timer number 00055 * @return Peripheral clock of timer 00056 **********************************************************************/ 00057 00058 uint32_t TIM_GetPClock (uint32_t timernum) 00059 { 00060 uint32_t clkdlycnt=0; 00061 switch (timernum) 00062 { 00063 case 0: 00064 clkdlycnt = CLKPWR_GetPCLK (CLKPWR_PCLKSEL_TIMER0); 00065 break; 00066 00067 case 1: 00068 clkdlycnt = CLKPWR_GetPCLK (CLKPWR_PCLKSEL_TIMER1); 00069 break; 00070 00071 case 2: 00072 clkdlycnt = CLKPWR_GetPCLK (CLKPWR_PCLKSEL_TIMER2); 00073 break; 00074 00075 case 3: 00076 clkdlycnt = CLKPWR_GetPCLK (CLKPWR_PCLKSEL_TIMER3); 00077 break; 00078 } 00079 return clkdlycnt; 00080 } 00081 00082 00083 /*********************************************************************//** 00084 * @brief Convert a time to a timer count value 00085 * @param[in] timernum Timer number 00086 * @param[in] usec Time in microseconds 00087 * @return The number of required clock ticks to give the time delay 00088 **********************************************************************/ 00089 uint32_t TIM_ConverUSecToVal (uint32_t timernum, uint32_t usec) 00090 { 00091 uint64_t clkdlycnt; 00092 00093 // Get Pclock of timer 00094 clkdlycnt = (uint64_t) TIM_GetPClock (timernum); 00095 00096 clkdlycnt = (clkdlycnt * usec) / 1000000; 00097 return (uint32_t) clkdlycnt; 00098 } 00099 00100 00101 /*********************************************************************//** 00102 * @brief Convert a timer register pointer to a timer number 00103 * @param[in] TIMx Pointer to a timer register set 00104 * @return The timer number (0 to 3) or -1 if register pointer is bad 00105 **********************************************************************/ 00106 uint32_t TIM_ConverPtrToTimeNum (LPC_TIM_TypeDef *TIMx) 00107 { 00108 uint32_t tnum = (uint32_t)-1; 00109 00110 if (TIMx == LPC_TIM0) 00111 { 00112 tnum = 0; 00113 } 00114 else if (TIMx == LPC_TIM1) 00115 { 00116 tnum = 1; 00117 } 00118 else if (TIMx == LPC_TIM2) 00119 { 00120 tnum = 2; 00121 } 00122 else if (TIMx == LPC_TIM3) 00123 { 00124 tnum = 3; 00125 } 00126 00127 return tnum; 00128 } 00129 00130 /** 00131 * @} 00132 */ 00133 00134 00135 /* Public Functions ----------------------------------------------------------- */ 00136 /** @addtogroup TIM_Public_Functions 00137 * @{ 00138 */ 00139 00140 /*********************************************************************//** 00141 * @brief Get Interrupt Status 00142 * @param[in] TIMx Timer selection, should be TIM0, TIM1, TIM2, TIM3 00143 * @param[in] IntFlag 00144 * @return FlagStatus 00145 * - SET : interrupt 00146 * - RESET : no interrupt 00147 **********************************************************************/ 00148 FlagStatus TIM_GetIntStatus(LPC_TIM_TypeDef *TIMx, uint8_t IntFlag) 00149 { 00150 CHECK_PARAM(PARAM_TIMx(TIMx)); 00151 CHECK_PARAM(PARAM_TIM_INT_TYPE(IntFlag)); 00152 uint8_t temp = (TIMx->IR)& TIM_IR_CLR(IntFlag); 00153 if (temp) 00154 return SET; 00155 00156 return RESET; 00157 00158 } 00159 /*********************************************************************//** 00160 * @brief Get Capture Interrupt Status 00161 * @param[in] TIMx Timer selection, should be TIM0, TIM1, TIM2, TIM3 00162 * @param[in] IntFlag 00163 * @return FlagStatus 00164 * - SET : interrupt 00165 * - RESET : no interrupt 00166 **********************************************************************/ 00167 FlagStatus TIM_GetIntCaptureStatus(LPC_TIM_TypeDef *TIMx, uint8_t IntFlag) 00168 { 00169 CHECK_PARAM(PARAM_TIMx(TIMx)); 00170 CHECK_PARAM(PARAM_TIM_INT_TYPE(IntFlag)); 00171 uint8_t temp = (TIMx->IR) & (1<<(4+IntFlag)); 00172 if(temp) 00173 return SET; 00174 return RESET; 00175 } 00176 /*********************************************************************//** 00177 * @brief Clear Interrupt pending 00178 * @param[in] TIMx Timer selection, should be TIM0, TIM1, TIM2, TIM3 00179 * @param[in] IntFlag should be in TIM_INT_TYPE enum 00180 * @return None 00181 **********************************************************************/ 00182 void TIM_ClearIntPending(LPC_TIM_TypeDef *TIMx, uint8_t IntFlag) 00183 { 00184 CHECK_PARAM(PARAM_TIMx(TIMx)); 00185 CHECK_PARAM(PARAM_TIM_INT_TYPE(IntFlag)); 00186 TIMx->IR |= TIM_IR_CLR(IntFlag); 00187 } 00188 00189 /*********************************************************************//** 00190 * @brief Clear Capture Interrupt pending 00191 * @param[in] TIMx Timer selection, should be TIM0, TIM1, TIM2, TIM3 00192 * @param[in] IntFlag should be in TIM_INT_TYPE enum 00193 * @return None 00194 **********************************************************************/ 00195 void TIM_ClearIntCapturePending(LPC_TIM_TypeDef *TIMx, uint8_t IntFlag) 00196 { 00197 CHECK_PARAM(PARAM_TIMx(TIMx)); 00198 CHECK_PARAM(PARAM_TIM_INT_TYPE(IntFlag)); 00199 TIMx->IR |= (1<<(4+IntFlag)); 00200 } 00201 00202 /*********************************************************************//** 00203 * @brief Configuration for Timer at initial time 00204 * @param[in] TimerCounterMode Should be : 00205 * - PrescaleOption = TC_PRESCALE_USVAL, 00206 * - PrescaleValue = 1 00207 * Counter mode with 00208 * - Caption channel 0 00209 * @param[in] TIM_ConfigStruct pointer to TIM_TIMERCFG_Type or 00210 * TIM_COUNTERCFG_Type 00211 * @return None 00212 **********************************************************************/ 00213 void TIM_ConfigStructInit(uint8_t TimerCounterMode, void *TIM_ConfigStruct) 00214 { 00215 if (TimerCounterMode == TIM_TIMER_MODE ) 00216 { 00217 TIM_TIMERCFG_Type * pTimeCfg = (TIM_TIMERCFG_Type *)TIM_ConfigStruct; 00218 pTimeCfg->PrescaleOption = TIM_PRESCALE_USVAL ; 00219 pTimeCfg->PrescaleValue = 1; 00220 } 00221 else 00222 { 00223 TIM_COUNTERCFG_Type * pCounterCfg = (TIM_COUNTERCFG_Type *)TIM_ConfigStruct; 00224 pCounterCfg->CountInputSelect = TIM_COUNTER_INCAP0 ; 00225 00226 } 00227 } 00228 00229 /*********************************************************************//** 00230 * @brief Initial Timer/Counter device 00231 * Set Clock frequency for Timer 00232 * Set initial configuration for Timer 00233 * @param[in] TIMx Timer selection, should be TIM0, TIM1, TIM2, TIM3 00234 * @param[in] TimerCounterMode TIM_MODE_OPT 00235 * @param[in] TIM_ConfigStruct pointer to TIM_TIMERCFG_Type 00236 * that contains the configuration information for the 00237 * specified Timer peripheral. 00238 * @return None 00239 **********************************************************************/ 00240 void TIM_Init(LPC_TIM_TypeDef *TIMx, uint8_t TimerCounterMode, void *TIM_ConfigStruct) 00241 { 00242 TIM_TIMERCFG_Type *pTimeCfg; 00243 TIM_COUNTERCFG_Type *pCounterCfg; 00244 //uint32_t timer; 00245 00246 CHECK_PARAM(PARAM_TIMx(TIMx)); 00247 CHECK_PARAM(PARAM_TIM_MODE_OPT(TimerCounterMode)); 00248 00249 //timer = TIM_ConverPtrToTimeNum(TIMx) ; 00250 //set power 00251 if (TIMx== LPC_TIM0) 00252 { 00253 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM0, ENABLE); 00254 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_TIMER0, CLKPWR_PCLKSEL_CCLK_DIV_4); 00255 } 00256 else if (TIMx== LPC_TIM1) 00257 { 00258 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM1, ENABLE); 00259 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_TIMER1, CLKPWR_PCLKSEL_CCLK_DIV_4); 00260 00261 } 00262 00263 else if (TIMx== LPC_TIM2) 00264 { 00265 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM2, ENABLE); 00266 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_TIMER2, CLKPWR_PCLKSEL_CCLK_DIV_4); 00267 } 00268 else if (TIMx== LPC_TIM3) 00269 { 00270 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM3, ENABLE); 00271 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_TIMER3, CLKPWR_PCLKSEL_CCLK_DIV_4); 00272 00273 } 00274 00275 TIMx->CCR &= ~TIM_CTCR_MODE_MASK; 00276 TIMx->CCR |= TIM_TIMER_MODE ; 00277 00278 TIMx->TC =0; 00279 TIMx->PC =0; 00280 TIMx->PR =0; 00281 if (TimerCounterMode == TIM_TIMER_MODE ) 00282 { 00283 pTimeCfg = (TIM_TIMERCFG_Type *)TIM_ConfigStruct; 00284 if (pTimeCfg->PrescaleOption == TIM_PRESCALE_TICKVAL ) 00285 { 00286 TIMx->PR = pTimeCfg->PrescaleValue -1 ; 00287 } 00288 else 00289 { 00290 TIMx->PR = TIM_ConverUSecToVal (TIM_ConverPtrToTimeNum(TIMx),pTimeCfg->PrescaleValue)-1; 00291 } 00292 } 00293 else 00294 { 00295 00296 pCounterCfg = (TIM_COUNTERCFG_Type *)TIM_ConfigStruct; 00297 TIMx->CCR &= ~TIM_CTCR_INPUT_MASK; 00298 if (pCounterCfg->CountInputSelect == TIM_COUNTER_INCAP1 ) 00299 TIMx->CCR |= _BIT(2); 00300 } 00301 00302 // Clear interrupt pending 00303 TIMx->IR = 0xFFFFFFFF; 00304 00305 } 00306 00307 /*********************************************************************//** 00308 * @brief Close Timer/Counter device 00309 * @param[in] TIMx Pointer to timer device 00310 * @return None 00311 **********************************************************************/ 00312 void TIM_DeInit (LPC_TIM_TypeDef *TIMx) 00313 { 00314 CHECK_PARAM(PARAM_TIMx(TIMx)); 00315 // Disable timer/counter 00316 TIMx->TCR = 0x00; 00317 00318 // Disable power 00319 if (TIMx== LPC_TIM0) 00320 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM0, DISABLE); 00321 00322 else if (TIMx== LPC_TIM1) 00323 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM1, DISABLE); 00324 00325 else if (TIMx== LPC_TIM2) 00326 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM2, DISABLE); 00327 00328 else if (TIMx== LPC_TIM3) 00329 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM2, DISABLE); 00330 00331 } 00332 00333 /*********************************************************************//** 00334 * @brief Start/Stop Timer/Counter device 00335 * @param[in] TIMx Pointer to timer device 00336 * @param[in] NewState 00337 * - ENABLE : set timer enable 00338 * - DISABLE : disable timer 00339 * @return None 00340 **********************************************************************/ 00341 void TIM_Cmd(LPC_TIM_TypeDef *TIMx, FunctionalState NewState) 00342 { 00343 CHECK_PARAM(PARAM_TIMx(TIMx)); 00344 if (NewState == ENABLE) 00345 { 00346 TIMx->TCR |= TIM_ENABLE; 00347 } 00348 else 00349 { 00350 TIMx->TCR &= ~TIM_ENABLE; 00351 } 00352 } 00353 00354 /*********************************************************************//** 00355 * @brief Reset Timer/Counter device, 00356 * Make TC and PC are synchronously reset on the next 00357 * positive edge of PCLK 00358 * @param[in] TIMx Pointer to timer device 00359 * @return None 00360 **********************************************************************/ 00361 void TIM_ResetCounter(LPC_TIM_TypeDef *TIMx) 00362 { 00363 CHECK_PARAM(PARAM_TIMx(TIMx)); 00364 TIMx->TCR |= TIM_RESET; 00365 TIMx->TCR &= ~TIM_RESET; 00366 } 00367 00368 /*********************************************************************//** 00369 * @brief Configuration for Match register 00370 * @param[in] TIMx Pointer to timer device 00371 * @param[in] TIM_MatchConfigStruct Pointer to TIM_MATCHCFG_Type 00372 * - MatchChannel : choose channel 0 or 1 00373 * - IntOnMatch : if SET, interrupt will be generated when MRxx match 00374 * the value in TC 00375 * - StopOnMatch : if SET, TC and PC will be stopped whenM Rxx match 00376 * the value in TC 00377 * - ResetOnMatch : if SET, Reset on MR0 when MRxx match 00378 * the value in TC 00379 * -ExtMatchOutputType: Select output for external match 00380 * + 0: Do nothing for external output pin if match 00381 * + 1: Force external output pin to low if match 00382 * + 2: Force external output pin to high if match 00383 * + 3: Toggle external output pin if match 00384 * MatchValue: Set the value to be compared with TC value 00385 * @return None 00386 **********************************************************************/ 00387 void TIM_ConfigMatch(LPC_TIM_TypeDef *TIMx, TIM_MATCHCFG_Type *TIM_MatchConfigStruct) 00388 { 00389 //uint32_t timer; 00390 00391 CHECK_PARAM(PARAM_TIMx(TIMx)); 00392 CHECK_PARAM(PARAM_TIM_EXTMATCH_OPT(TIM_MatchConfigStruct->ExtMatchOutputType)); 00393 00394 //timer = TIM_ConverPtrToTimeNum(TIMx) ; 00395 switch(TIM_MatchConfigStruct->MatchChannel) 00396 { 00397 case 0: 00398 TIMx->MR0 = TIM_MatchConfigStruct->MatchValue; 00399 break; 00400 case 1: 00401 TIMx->MR1 = TIM_MatchConfigStruct->MatchValue; 00402 break; 00403 } 00404 //interrupt on MRn 00405 TIMx->MCR &=~TIM_MCR_CHANNEL_MASKBIT(TIM_MatchConfigStruct->MatchChannel); 00406 00407 if (TIM_MatchConfigStruct->IntOnMatch) 00408 TIMx->MCR |= TIM_INT_ON_MATCH(TIM_MatchConfigStruct->MatchChannel); 00409 00410 //reset on MRn 00411 if (TIM_MatchConfigStruct->ResetOnMatch) 00412 TIMx->MCR |= TIM_RESET_ON_MATCH(TIM_MatchConfigStruct->MatchChannel); 00413 00414 //stop on MRn 00415 if (TIM_MatchConfigStruct->StopOnMatch) 00416 TIMx->MCR |= TIM_STOP_ON_MATCH(TIM_MatchConfigStruct->MatchChannel); 00417 // TIMx->MCR = 0x02; 00418 00419 // match output type 00420 00421 TIMx->EMR &= ~TIM_EM_MASK(TIM_MatchConfigStruct->MatchChannel); 00422 TIMx->EMR = TIM_EM_SET(TIM_MatchConfigStruct->MatchChannel,TIM_MatchConfigStruct->ExtMatchOutputType); 00423 } 00424 /*********************************************************************//** 00425 * @brief Configuration for Capture register 00426 * @param[in] TIMx Pointer to timer device 00427 * - CaptureChannel: set the channel to capture data 00428 * - RisingEdge : if SET, Capture at rising edge 00429 * - FallingEdge : if SET, Capture at falling edge 00430 * - IntOnCaption : if SET, Capture generate interrupt 00431 * @param[in] TIM_CaptureConfigStruct Pointer to TIM_CAPTURECFG_Type 00432 * @return None 00433 **********************************************************************/ 00434 void TIM_ConfigCapture(LPC_TIM_TypeDef *TIMx, TIM_CAPTURECFG_Type *TIM_CaptureConfigStruct) 00435 { 00436 //uint32_t timer; 00437 00438 CHECK_PARAM(PARAM_TIMx(TIMx)); 00439 //timer = TIM_ConverPtrToTimeNum(TIMx) ; 00440 TIMx->CCR &= ~TIM_CCR_CHANNEL_MASKBIT(TIM_CaptureConfigStruct->CaptureChannel); 00441 00442 if (TIM_CaptureConfigStruct->RisingEdge) 00443 TIMx->CCR |= TIM_CAP_RISING(TIM_CaptureConfigStruct->CaptureChannel); 00444 00445 if (TIM_CaptureConfigStruct->FallingEdge) 00446 TIMx->CCR |= TIM_CAP_FALLING(TIM_CaptureConfigStruct->CaptureChannel); 00447 00448 if (TIM_CaptureConfigStruct->IntOnCaption) 00449 TIMx->CCR |= TIM_INT_ON_CAP(TIM_CaptureConfigStruct->CaptureChannel); 00450 } 00451 00452 /*********************************************************************//** 00453 * @brief Read value of capture register in timer/counter device 00454 * @param[in] TIMx Pointer to timer/counter device 00455 * @param[in] CaptureChannel: capture channel number 00456 * @return Value of capture register 00457 **********************************************************************/ 00458 uint32_t TIM_GetCaptureValue(LPC_TIM_TypeDef *TIMx, uint8_t CaptureChannel) 00459 { 00460 CHECK_PARAM(PARAM_TIMx(TIMx)); 00461 CHECK_PARAM(PARAM_TIM_COUNTER_INPUT_OPT(CaptureChannel)); 00462 00463 if(CaptureChannel==0) 00464 return TIMx->CR0; 00465 else 00466 return TIMx->CR1; 00467 } 00468 00469 /** 00470 * @} 00471 */ 00472 00473 #endif /* _TIMER */ 00474 00475 /** 00476 * @} 00477 */ 00478 00479 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
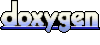