
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_pinsel.h
00001 /***********************************************************************//** 00002 * @file : lpc17xx_pinsel.h 00003 * @brief : Contains all macro definitions and function prototypes 00004 * support for Pin connect block firmware library on LPC17xx 00005 * @version : 1.0 00006 * @date : 25. Feb. 2009 00007 * @author : HoanTran 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup PINSEL 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_PINSEL_H_ 00028 #define LPC17XX_PINSEL_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "cmsis.h" 00032 #include "lpc_types.h" 00033 00034 #ifdef __cplusplus 00035 extern "C" 00036 { 00037 #endif 00038 00039 00040 /* Private Macros ------------------------------------------------------------- */ 00041 /** @defgroup PINSEL_Private_Macros 00042 * @{ 00043 */ 00044 00045 /** @defgroup PINSEL_REGISTER_BIT_DEFINITIONS 00046 * @{ 00047 */ 00048 00049 /* Pin selection define */ 00050 /* I2C Pin Configuration register bit description */ 00051 #define PINSEL_I2CPADCFG_SDADRV0 _BIT(0) /**< Drive mode control for the SDA0 pin, P0.27 */ 00052 #define PINSEL_I2CPADCFG_SDAI2C0 _BIT(1) /**< I2C mode control for the SDA0 pin, P0.27 */ 00053 #define PINSEL_I2CPADCFG_SCLDRV0 _BIT(2) /**< Drive mode control for the SCL0 pin, P0.28 */ 00054 #define PINSEL_I2CPADCFG_SCLI2C0 _BIT(3) /**< I2C mode control for the SCL0 pin, P0.28 */ 00055 00056 /** 00057 * @} 00058 */ 00059 00060 /** 00061 * @} 00062 */ 00063 00064 00065 /* Public Macros -------------------------------------------------------------- */ 00066 /** @defgroup PINSEL_Public_Macros 00067 * @{ 00068 */ 00069 00070 /*********************************************************************//** 00071 *!< Macros define for PORT Selection 00072 ***********************************************************************/ 00073 #define PINSEL_PORT_0 ((0)) /**< PORT 0*/ 00074 #define PINSEL_PORT_1 ((1)) /**< PORT 1*/ 00075 #define PINSEL_PORT_2 ((2)) /**< PORT 2*/ 00076 #define PINSEL_PORT_3 ((3)) /**< PORT 3*/ 00077 #define PINSEL_PORT_4 ((4)) /**< PORT 4*/ 00078 00079 00080 /*********************************************************************** 00081 * Macros define for Pin Function selection 00082 **********************************************************************/ 00083 #define PINSEL_FUNC_0 ((0)) /**< default function*/ 00084 #define PINSEL_FUNC_1 ((1)) /**< first alternate function*/ 00085 #define PINSEL_FUNC_2 ((2)) /**< second alternate function*/ 00086 #define PINSEL_FUNC_3 ((3)) /**< third or reserved alternate function*/ 00087 00088 00089 00090 /*********************************************************************** 00091 * Macros define for Pin Number of Port 00092 **********************************************************************/ 00093 #define PINSEL_PIN_0 ((0)) /**< Pin 0 */ 00094 #define PINSEL_PIN_1 ((1)) /**< Pin 1 */ 00095 #define PINSEL_PIN_2 ((2)) /**< Pin 2 */ 00096 #define PINSEL_PIN_3 ((3)) /**< Pin 3 */ 00097 #define PINSEL_PIN_4 ((4)) /**< Pin 4 */ 00098 #define PINSEL_PIN_5 ((5)) /**< Pin 5 */ 00099 #define PINSEL_PIN_6 ((6)) /**< Pin 6 */ 00100 #define PINSEL_PIN_7 ((7)) /**< Pin 7 */ 00101 #define PINSEL_PIN_8 ((8)) /**< Pin 8 */ 00102 #define PINSEL_PIN_9 ((9)) /**< Pin 9 */ 00103 #define PINSEL_PIN_10 ((10)) /**< Pin 10 */ 00104 #define PINSEL_PIN_11 ((11)) /**< Pin 11 */ 00105 #define PINSEL_PIN_12 ((12)) /**< Pin 12 */ 00106 #define PINSEL_PIN_13 ((13)) /**< Pin 13 */ 00107 #define PINSEL_PIN_14 ((14)) /**< Pin 14 */ 00108 #define PINSEL_PIN_15 ((15)) /**< Pin 15 */ 00109 #define PINSEL_PIN_16 ((16)) /**< Pin 16 */ 00110 #define PINSEL_PIN_17 ((17)) /**< Pin 17 */ 00111 #define PINSEL_PIN_18 ((18)) /**< Pin 18 */ 00112 #define PINSEL_PIN_19 ((19)) /**< Pin 19 */ 00113 #define PINSEL_PIN_20 ((20)) /**< Pin 20 */ 00114 #define PINSEL_PIN_21 ((21)) /**< Pin 21 */ 00115 #define PINSEL_PIN_22 ((22)) /**< Pin 22 */ 00116 #define PINSEL_PIN_23 ((23)) /**< Pin 23 */ 00117 #define PINSEL_PIN_24 ((24)) /**< Pin 24 */ 00118 #define PINSEL_PIN_25 ((25)) /**< Pin 25 */ 00119 #define PINSEL_PIN_26 ((26)) /**< Pin 26 */ 00120 #define PINSEL_PIN_27 ((27)) /**< Pin 27 */ 00121 #define PINSEL_PIN_28 ((28)) /**< Pin 28 */ 00122 #define PINSEL_PIN_29 ((29)) /**< Pin 29 */ 00123 #define PINSEL_PIN_30 ((30)) /**< Pin 30 */ 00124 #define PINSEL_PIN_31 ((31)) /**< Pin 31 */ 00125 00126 00127 /*********************************************************************** 00128 * Macros define for Pin mode 00129 **********************************************************************/ 00130 #define PINSEL_PINMODE_PULLUP ((0)) /**< Internal pull-up resistor*/ 00131 #define PINSEL_PINMODE_TRISTATE ((2)) /**< Tri-state */ 00132 #define PINSEL_PINMODE_PULLDOWN ((3)) /**< Internal pull-down resistor */ 00133 00134 00135 /*********************************************************************** 00136 * Macros define for Pin mode (normal/open drain) 00137 **********************************************************************/ 00138 #define PINSEL_PINMODE_NORMAL ((0)) /**< Pin is in the normal (not open drain) mode.*/ 00139 #define PINSEL_PINMODE_OPENDRAIN ((1)) /**< Pin is in the open drain mode */ 00140 00141 00142 /*********************************************************************** 00143 * Macros define for I2C mode 00144 ***********************************************************************/ 00145 #define PINSEL_I2C_Normal_Mode ((0)) /**< The standard drive mode */ 00146 #define PINSEL_I2C_Fast_Mode ((1)) /**< Fast Mode Plus drive mode */ 00147 00148 00149 /** 00150 * @} 00151 */ 00152 00153 00154 /* Public Types --------------------------------------------------------------- */ 00155 /** @defgroup PINSEL_Public_Types 00156 * @{ 00157 */ 00158 00159 /** @brief Pin configuration structure */ 00160 typedef struct 00161 { 00162 uint8_t Portnum; /**< Port Number, should be PINSEL_PORT_x, 00163 where x should be in range from 0 to 4 */ 00164 uint8_t Pinnum; /**< Pin Number, should be PINSEL_PIN_x, 00165 where x should be in range from 0 to 31 */ 00166 uint8_t Funcnum; /**< Function Number, should be PINSEL_FUNC_x, 00167 where x should be in range from 0 to 3 */ 00168 uint8_t Pinmode; /**< Pin Mode, should be: 00169 - PINSEL_PINMODE_PULLUP: Internal pull-up resistor 00170 - PINSEL_PINMODE_TRISTATE: Tri-state 00171 - PINSEL_PINMODE_PULLDOWN: Internal pull-down resistor */ 00172 uint8_t OpenDrain; /**< OpenDrain mode, should be: 00173 - PINSEL_PINMODE_NORMAL: Pin is in the normal (not open drain) mode 00174 - PINSEL_PINMODE_OPENDRAIN: Pin is in the open drain mode */ 00175 } PINSEL_CFG_Type; 00176 00177 /** 00178 * @} 00179 */ 00180 00181 00182 /* Public Functions ----------------------------------------------------------- */ 00183 /** @defgroup PINSEL_Public_Functions 00184 * @{ 00185 */ 00186 00187 void PINSEL_SetPinFunc ( uint8_t portnum, uint8_t pinnum, uint8_t funcnum); 00188 void PINSEL_ConfigTraceFunc (FunctionalState NewState); 00189 void PINSEL_SetResistorMode ( uint8_t portnum, uint8_t pinnum, uint8_t modenum); 00190 void PINSEL_SetOpenDrainMode( uint8_t portnum, uint8_t pinnum, uint8_t modenum); 00191 void PINSEL_SetI2C0Pins(uint8_t i2cPinMode, FunctionalState filterSlewRateEnable); 00192 void PINSEL_ConfigPin(PINSEL_CFG_Type *PinCfg); 00193 00194 /** 00195 * @} 00196 */ 00197 00198 00199 #ifdef __cplusplus 00200 } 00201 #endif 00202 00203 #endif /* LPC17XX_PINSEL_H_ */ 00204 00205 /** 00206 * @} 00207 */ 00208 00209 /* --------------------------------- End Of File ------------------------------ */ 00210
Generated on Tue Jul 12 2022 17:06:02 by
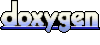