
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_mcpwm.c
00001 /** 00002 * @file : lpc17xx_mcpwm.c 00003 * @brief : Contains all functions support for Motor Control PWM firmware 00004 * library on LPC17xx 00005 * @version : 1.0 00006 * @date : 26. May. 2009 00007 * @author : HieuNguyen 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **********************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @addtogroup MCPWM 00023 * @{ 00024 */ 00025 00026 /* Includes ------------------------------------------------------------------- */ 00027 #include "lpc17xx_mcpwm.h" 00028 #include "lpc17xx_clkpwr.h" 00029 00030 /* If this source file built with example, the LPC17xx FW library configuration 00031 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00032 * otherwise the default FW library configuration file must be included instead 00033 */ 00034 #ifdef __BUILD_WITH_EXAMPLE__ 00035 #include "lpc17xx_libcfg.h" 00036 #else 00037 #include "lpc17xx_libcfg_default.h" 00038 #endif /* __BUILD_WITH_EXAMPLE__ */ 00039 00040 00041 #ifdef _MCPWM 00042 00043 /* Public Functions ----------------------------------------------------------- */ 00044 /** @addtogroup MCPWM_Public_Functions 00045 * @{ 00046 */ 00047 00048 /*********************************************************************//** 00049 * @brief Initializes the MCPWM peripheral 00050 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00051 * @return None 00052 **********************************************************************/ 00053 void MCPWM_Init(LPC_MCPWM_TypeDef *MCPWMx) 00054 { 00055 00056 /* Turn On MCPWM PCLK */ 00057 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCMC, ENABLE); 00058 /* As default, peripheral clock for MCPWM module 00059 * is set to FCCLK / 2 */ 00060 // CLKPWR_SetPCLKDiv(CLKPWR_PCLKSEL_MC, CLKPWR_PCLKSEL_CCLK_DIV_2); 00061 00062 MCPWMx->MCCAP_CLR = MCPWM_CAPCLR_CAP(0) | MCPWM_CAPCLR_CAP(1) | MCPWM_CAPCLR_CAP(2); 00063 MCPWMx->MCINTFLAG_CLR = MCPWM_INT_ILIM(0) | MCPWM_INT_ILIM(1) | MCPWM_INT_ILIM(2) \ 00064 | MCPWM_INT_IMAT(0) | MCPWM_INT_IMAT(1) | MCPWM_INT_IMAT(2) \ 00065 | MCPWM_INT_ICAP(0) | MCPWM_INT_ICAP(1) | MCPWM_INT_ICAP(2); 00066 MCPWMx->MCINTEN_CLR = MCPWM_INT_ILIM(0) | MCPWM_INT_ILIM(1) | MCPWM_INT_ILIM(2) \ 00067 | MCPWM_INT_IMAT(0) | MCPWM_INT_IMAT(1) | MCPWM_INT_IMAT(2) \ 00068 | MCPWM_INT_ICAP(0) | MCPWM_INT_ICAP(1) | MCPWM_INT_ICAP(2); 00069 } 00070 00071 00072 /*********************************************************************//** 00073 * @brief Configures each channel in MCPWM peripheral according to the 00074 * specified parameters in the MCPWM_CHANNEL_CFG_Type. 00075 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00076 * @param[in] channelNum Channel number, should be in range from 0 to 2. 00077 * @param[in] channelSetup Pointer to a MCPWM_CHANNEL_CFG_Type structure 00078 * that contains the configuration information for the 00079 * specified MCPWM channel. 00080 * @return None 00081 **********************************************************************/ 00082 void MCPWM_ConfigChannel(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00083 MCPWM_CHANNEL_CFG_Type * channelSetup) 00084 { 00085 if (channelNum <= 2) { 00086 if (channelNum == 0) { 00087 MCPWMx->MCTIM0 = channelSetup->channelTimercounterValue; 00088 MCPWMx->MCPER0 = channelSetup->channelPeriodValue; 00089 MCPWMx->MCPW0 = channelSetup->channelPulsewidthValue; 00090 } else if (channelNum == 1) { 00091 MCPWMx->MCTIM1 = channelSetup->channelTimercounterValue; 00092 MCPWMx->MCPER1 = channelSetup->channelPeriodValue; 00093 MCPWMx->MCPW1 = channelSetup->channelPulsewidthValue; 00094 } else if (channelNum == 2) { 00095 MCPWMx->MCTIM2 = channelSetup->channelTimercounterValue; 00096 MCPWMx->MCPER2 = channelSetup->channelPeriodValue; 00097 MCPWMx->MCPW2 = channelSetup->channelPulsewidthValue; 00098 } else { 00099 return; 00100 } 00101 00102 if (channelSetup->channelType == MCPWM_CHANNEL_CENTER_MODE){ 00103 MCPWMx->MCCON_SET = MCPWM_CON_CENTER(channelNum); 00104 } else { 00105 MCPWMx->MCCON_CLR = MCPWM_CON_CENTER(channelNum); 00106 } 00107 00108 if (channelSetup->channelPolarity == MCPWM_CHANNEL_PASSIVE_HI){ 00109 MCPWMx->MCCON_SET = MCPWM_CON_POLAR(channelNum); 00110 } else { 00111 MCPWMx->MCCON_CLR = MCPWM_CON_POLAR(channelNum); 00112 } 00113 00114 if (channelSetup->channelDeadtimeEnable /* == ENABLE */){ 00115 MCPWMx->MCCON_SET = MCPWM_CON_DTE(channelNum); 00116 MCPWMx->MCDEADTIME &= ~(MCPWM_DT(channelNum, 0x3FF)); 00117 MCPWMx->MCDEADTIME |= MCPWM_DT(channelNum, channelSetup->channelDeadtimeValue); 00118 } else { 00119 MCPWMx->MCCON_CLR = MCPWM_CON_DTE(channelNum); 00120 } 00121 00122 if (channelSetup->channelUpdateEnable /* == ENABLE */){ 00123 MCPWMx->MCCON_CLR = MCPWM_CON_DISUP(channelNum); 00124 } else { 00125 MCPWMx->MCCON_SET = MCPWM_CON_DISUP(channelNum); 00126 } 00127 } 00128 } 00129 00130 00131 /*********************************************************************//** 00132 * @brief Write to MCPWM shadow registers - Update the value for period 00133 * and pulse width in MCPWM peripheral. 00134 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00135 * @param[in] channelNum Channel Number, should be in range from 0 to 2. 00136 * @param[in] channelSetup Pointer to a MCPWM_CHANNEL_CFG_Type structure 00137 * that contains the configuration information for the 00138 * specified MCPWM channel. 00139 * @return None 00140 **********************************************************************/ 00141 void MCPWM_WriteToShadow(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00142 MCPWM_CHANNEL_CFG_Type *channelSetup) 00143 { 00144 if (channelNum == 0){ 00145 MCPWMx->MCPER0 = channelSetup->channelPeriodValue; 00146 MCPWMx->MCPW0 = channelSetup->channelPulsewidthValue; 00147 } else if (channelNum == 1) { 00148 MCPWMx->MCPER1 = channelSetup->channelPeriodValue; 00149 MCPWMx->MCPW1 = channelSetup->channelPulsewidthValue; 00150 } else if (channelNum == 2) { 00151 MCPWMx->MCPER2 = channelSetup->channelPeriodValue; 00152 MCPWMx->MCPW2 = channelSetup->channelPulsewidthValue; 00153 } 00154 } 00155 00156 00157 00158 /*********************************************************************//** 00159 * @brief Configures capture function in MCPWM peripheral 00160 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00161 * @param[in] channelNum MCI (Motor Control Input pin) number, should be in range from 0 to 2. 00162 * @param[in] captureConfig Pointer to a MCPWM_CAPTURE_CFG_Type structure 00163 * that contains the configuration information for the 00164 * specified MCPWM capture. 00165 * @return 00166 **********************************************************************/ 00167 void MCPWM_ConfigCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00168 MCPWM_CAPTURE_CFG_Type *captureConfig) 00169 { 00170 if (channelNum <= 2) { 00171 00172 if (captureConfig->captureFalling /* == ENABLE */) { 00173 MCPWMx->MCCAPCON_SET = MCPWM_CAPCON_CAPMCI_FE(captureConfig->captureChannel, channelNum); 00174 } else { 00175 MCPWMx->MCCAPCON_CLR = MCPWM_CAPCON_CAPMCI_FE(captureConfig->captureChannel, channelNum); 00176 } 00177 00178 if (captureConfig->captureRising /* == ENABLE */) { 00179 MCPWMx->MCCAPCON_SET = MCPWM_CAPCON_CAPMCI_RE(captureConfig->captureChannel, channelNum); 00180 } else { 00181 MCPWMx->MCCAPCON_CLR = MCPWM_CAPCON_CAPMCI_RE(captureConfig->captureChannel, channelNum); 00182 } 00183 00184 if (captureConfig->timerReset /* == ENABLE */){ 00185 MCPWMx->MCCAPCON_SET = MCPWM_CAPCON_RT(captureConfig->captureChannel); 00186 } else { 00187 MCPWMx->MCCAPCON_CLR = MCPWM_CAPCON_RT(captureConfig->captureChannel); 00188 } 00189 00190 if (captureConfig->hnfEnable /* == ENABLE */){ 00191 MCPWMx->MCCAPCON_SET = MCPWM_CAPCON_HNFCAP(channelNum); 00192 } else { 00193 MCPWMx->MCCAPCON_CLR = MCPWM_CAPCON_HNFCAP(channelNum); 00194 } 00195 } 00196 } 00197 00198 00199 /*********************************************************************//** 00200 * @brief Clears current captured value in specified capture channel 00201 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00202 * @param[in] captureChannel Capture channel number, should be in range from 0 to 2 00203 * @return None 00204 **********************************************************************/ 00205 void MCPWM_ClearCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t captureChannel) 00206 { 00207 MCPWMx->MCCAP_CLR = MCPWM_CAPCLR_CAP(captureChannel); 00208 } 00209 00210 /*********************************************************************//** 00211 * @brief Get current captured value in specified capture channel 00212 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00213 * @param[in] captureChannel Capture channel number, should be in range from 0 to 2 00214 * @return None 00215 **********************************************************************/ 00216 uint32_t MCPWM_GetCapture(LPC_MCPWM_TypeDef *MCPWMx, uint32_t captureChannel) 00217 { 00218 if (captureChannel == 0){ 00219 return (MCPWMx->MCCR0); 00220 } else if (captureChannel == 1) { 00221 return (MCPWMx->MCCR1); 00222 } else if (captureChannel == 2) { 00223 return (MCPWMx->MCCR2); 00224 } 00225 return (0); 00226 } 00227 00228 00229 /*********************************************************************//** 00230 * @brief Configures Count control in MCPWM peripheral 00231 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00232 * @param[in] channelNum Channel number, should be in range from 0 to 2 00233 * @param[in] countMode Count mode, should be: 00234 * - ENABLE: Enables count mode. 00235 * - DISABLE: Disable count mode, the channel is in timer mode. 00236 * @param[in] countConfig Pointer to a MCPWM_COUNT_CFG_Type structure 00237 * that contains the configuration information for the 00238 * specified MCPWM count control. 00239 * @return None 00240 **********************************************************************/ 00241 void MCPWM_CountConfig(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channelNum, 00242 uint32_t countMode, MCPWM_COUNT_CFG_Type *countConfig) 00243 { 00244 if ((channelNum <= 2)) { 00245 if (countMode /* == ENABLE */){ 00246 MCPWMx->MCCNTCON_SET = MCPWM_CNTCON_CNTR(channelNum); 00247 if (countConfig->countFalling /* == ENABLE */) { 00248 MCPWMx->MCCNTCON_SET = MCPWM_CNTCON_TCMCI_FE(countConfig->counterChannel,channelNum); 00249 } else { 00250 MCPWMx->MCCNTCON_CLR = MCPWM_CNTCON_TCMCI_FE(countConfig->counterChannel,channelNum); 00251 } 00252 if (countConfig->countRising /* == ENABLE */) { 00253 MCPWMx->MCCNTCON_SET = MCPWM_CNTCON_TCMCI_RE(countConfig->counterChannel,channelNum); 00254 } else { 00255 MCPWMx->MCCNTCON_CLR = MCPWM_CNTCON_TCMCI_RE(countConfig->counterChannel,channelNum); 00256 } 00257 } else { 00258 MCPWMx->MCCNTCON_CLR = MCPWM_CNTCON_CNTR(channelNum); 00259 } 00260 } 00261 } 00262 00263 00264 /*********************************************************************//** 00265 * @brief Start MCPWM activity for each MCPWM channel 00266 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00267 * @param[in] channel0 State of this command on channel 0: 00268 * - ENABLE: 'Start' command will effect on channel 0 00269 * - DISABLE: 'Start' command will not effect on channel 0 00270 * @param[in] channel1 State of this command on channel 1: 00271 * - ENABLE: 'Start' command will effect on channel 1 00272 * - DISABLE: 'Start' command will not effect on channel 1 00273 * @param[in] channel2 State of this command on channel 2: 00274 * - ENABLE: 'Start' command will effect on channel 2 00275 * - DISABLE: 'Start' command will not effect on channel 2 00276 * @return None 00277 **********************************************************************/ 00278 void MCPWM_Start(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channel0, 00279 uint32_t channel1, uint32_t channel2) 00280 { 00281 uint32_t regVal = 0; 00282 regVal = (channel0 ? MCPWM_CON_RUN(0) : 0) | (channel1 ? MCPWM_CON_RUN(1) : 0) \ 00283 | (channel2 ? MCPWM_CON_RUN(2) : 0); 00284 MCPWMx->MCCON_SET = regVal; 00285 } 00286 00287 00288 /*********************************************************************//** 00289 * @brief Stop MCPWM activity for each MCPWM channel 00290 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00291 * @param[in] channel0 State of this command on channel 0: 00292 * - ENABLE: 'Stop' command will effect on channel 0 00293 * - DISABLE: 'Stop' command will not effect on channel 0 00294 * @param[in] channel1 State of this command on channel 1: 00295 * - ENABLE: 'Stop' command will effect on channel 1 00296 * - DISABLE: 'Stop' command will not effect on channel 1 00297 * @param[in] channel2 State of this command on channel 2: 00298 * - ENABLE: 'Stop' command will effect on channel 2 00299 * - DISABLE: 'Stop' command will not effect on channel 2 00300 * @return None 00301 **********************************************************************/ 00302 void MCPWM_Stop(LPC_MCPWM_TypeDef *MCPWMx, uint32_t channel0, 00303 uint32_t channel1, uint32_t channel2) 00304 { 00305 uint32_t regVal = 0; 00306 regVal = (channel0 ? MCPWM_CON_RUN(0) : 0) | (channel1 ? MCPWM_CON_RUN(1) : 0) \ 00307 | (channel2 ? MCPWM_CON_RUN(2) : 0); 00308 MCPWMx->MCCON_CLR = regVal; 00309 } 00310 00311 00312 /*********************************************************************//** 00313 * @brief Enables/Disables 3-phase AC motor mode on MCPWM peripheral 00314 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00315 * @param[in] acMode State of this command, should be: 00316 * - ENABLE. 00317 * - DISABLE. 00318 * @return None 00319 **********************************************************************/ 00320 void MCPWM_ACMode(LPC_MCPWM_TypeDef *MCPWMx, uint32_t acMode) 00321 { 00322 if (acMode){ 00323 MCPWMx->MCCON_SET = MCPWM_CON_ACMODE; 00324 } else { 00325 MCPWMx->MCCON_CLR = MCPWM_CON_ACMODE; 00326 } 00327 } 00328 00329 00330 /*********************************************************************//** 00331 * @brief Enables/Disables 3-phase DC motor mode on MCPWM peripheral 00332 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00333 * @param[in] dcMode State of this command, should be: 00334 * - ENABLE. 00335 * - DISABLE. 00336 * @param[in] outputInvered Polarity of the MCOB outputs for all 3 channels, 00337 * should be: 00338 * - ENABLE: The MCOB outputs have opposite polarity 00339 * from the MCOA outputs. 00340 * - DISABLE: The MCOB outputs have the same basic 00341 * polarity as the MCOA outputs. 00342 * @param[in] outputPattern A value contains bits that enables/disables the specified 00343 * output pins route to the internal MCOA0 signal, should be: 00344 - MCPWM_PATENT_A0: MCOA0 tracks internal MCOA0 00345 - MCPWM_PATENT_B0: MCOB0 tracks internal MCOA0 00346 - MCPWM_PATENT_A1: MCOA1 tracks internal MCOA0 00347 - MCPWM_PATENT_B1: MCOB1 tracks internal MCOA0 00348 - MCPWM_PATENT_A2: MCOA2 tracks internal MCOA0 00349 - MCPWM_PATENT_B2: MCOB2 tracks internal MCOA0 00350 * @return None 00351 * 00352 * Note: all these outputPatent values above can be ORed together for using as input parameter. 00353 **********************************************************************/ 00354 void MCPWM_DCMode(LPC_MCPWM_TypeDef *MCPWMx, uint32_t dcMode, 00355 uint32_t outputInvered, uint32_t outputPattern) 00356 { 00357 if (dcMode){ 00358 MCPWMx->MCCON_SET = MCPWM_CON_DCMODE; 00359 } else { 00360 MCPWMx->MCCON_CLR = MCPWM_CON_DCMODE; 00361 } 00362 00363 if (outputInvered) { 00364 MCPWMx->MCCON_SET = MCPWM_CON_INVBDC; 00365 } else { 00366 MCPWMx->MCCON_CLR = MCPWM_CON_INVBDC; 00367 } 00368 00369 MCPWMx->MCCCP = outputPattern; 00370 } 00371 00372 00373 /*********************************************************************//** 00374 * @brief Configures the specified interrupt in MCPWM peripheral 00375 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00376 * @param[in] ulIntType Interrupt type, should be: 00377 * - MCPWM_INTFLAG_LIM0: Limit interrupt for channel (0) 00378 * - MCPWM_INTFLAG_MAT0: Match interrupt for channel (0) 00379 * - MCPWM_INTFLAG_CAP0: Capture interrupt for channel (0) 00380 * - MCPWM_INTFLAG_LIM1: Limit interrupt for channel (1) 00381 * - MCPWM_INTFLAG_MAT1: Match interrupt for channel (1) 00382 * - MCPWM_INTFLAG_CAP1: Capture interrupt for channel (1) 00383 * - MCPWM_INTFLAG_LIM2: Limit interrupt for channel (2) 00384 * - MCPWM_INTFLAG_MAT2: Match interrupt for channel (2) 00385 * - MCPWM_INTFLAG_CAP2: Capture interrupt for channel (2) 00386 * - MCPWM_INTFLAG_ABORT: Fast abort interrupt 00387 * @param[in] NewState New State of this command, should be: 00388 * - ENABLE. 00389 * - DISABLE. 00390 * @return None 00391 * 00392 * Note: all these ulIntType values above can be ORed together for using as input parameter. 00393 **********************************************************************/ 00394 void MCPWM_IntConfig(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType, FunctionalState NewState) 00395 { 00396 if (NewState) { 00397 MCPWMx->MCINTEN_SET = ulIntType; 00398 } else { 00399 MCPWMx->MCINTEN_CLR = ulIntType; 00400 } 00401 } 00402 00403 00404 /*********************************************************************//** 00405 * @brief Sets/Forces the specified interrupt for MCPWM peripheral 00406 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00407 * @param[in] ulIntType Interrupt type, should be: 00408 * - MCPWM_INTFLAG_LIM0: Limit interrupt for channel (0) 00409 * - MCPWM_INTFLAG_MAT0: Match interrupt for channel (0) 00410 * - MCPWM_INTFLAG_CAP0: Capture interrupt for channel (0) 00411 * - MCPWM_INTFLAG_LIM1: Limit interrupt for channel (1) 00412 * - MCPWM_INTFLAG_MAT1: Match interrupt for channel (1) 00413 * - MCPWM_INTFLAG_CAP1: Capture interrupt for channel (1) 00414 * - MCPWM_INTFLAG_LIM2: Limit interrupt for channel (2) 00415 * - MCPWM_INTFLAG_MAT2: Match interrupt for channel (2) 00416 * - MCPWM_INTFLAG_CAP2: Capture interrupt for channel (2) 00417 * - MCPWM_INTFLAG_ABORT: Fast abort interrupt 00418 * @return None 00419 * Note: all these ulIntType values above can be ORed together for using as input parameter. 00420 **********************************************************************/ 00421 void MCPWM_IntSet(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) 00422 { 00423 MCPWMx->MCINTFLAG_SET = ulIntType; 00424 } 00425 00426 00427 /*********************************************************************//** 00428 * @brief Clear the specified interrupt pending for MCPWM peripheral 00429 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00430 * @param[in] ulIntType Interrupt type, should be: 00431 * - MCPWM_INTFLAG_LIM0: Limit interrupt for channel (0) 00432 * - MCPWM_INTFLAG_MAT0: Match interrupt for channel (0) 00433 * - MCPWM_INTFLAG_CAP0: Capture interrupt for channel (0) 00434 * - MCPWM_INTFLAG_LIM1: Limit interrupt for channel (1) 00435 * - MCPWM_INTFLAG_MAT1: Match interrupt for channel (1) 00436 * - MCPWM_INTFLAG_CAP1: Capture interrupt for channel (1) 00437 * - MCPWM_INTFLAG_LIM2: Limit interrupt for channel (2) 00438 * - MCPWM_INTFLAG_MAT2: Match interrupt for channel (2) 00439 * - MCPWM_INTFLAG_CAP2: Capture interrupt for channel (2) 00440 * - MCPWM_INTFLAG_ABORT: Fast abort interrupt 00441 * @return None 00442 * Note: all these ulIntType values above can be ORed together for using as input parameter. 00443 **********************************************************************/ 00444 void MCPWM_IntClear(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) 00445 { 00446 MCPWMx->MCINTFLAG_CLR = ulIntType; 00447 } 00448 00449 00450 /*********************************************************************//** 00451 * @brief Check whether if the specified interrupt in MCPWM is set or not 00452 * @param[in] MCPWMx Motor Control PWM peripheral selected, should be MCPWM 00453 * @param[in] ulIntType Interrupt type, should be: 00454 * - MCPWM_INTFLAG_LIM0: Limit interrupt for channel (0) 00455 * - MCPWM_INTFLAG_MAT0: Match interrupt for channel (0) 00456 * - MCPWM_INTFLAG_CAP0: Capture interrupt for channel (0) 00457 * - MCPWM_INTFLAG_LIM1: Limit interrupt for channel (1) 00458 * - MCPWM_INTFLAG_MAT1: Match interrupt for channel (1) 00459 * - MCPWM_INTFLAG_CAP1: Capture interrupt for channel (1) 00460 * - MCPWM_INTFLAG_LIM2: Limit interrupt for channel (2) 00461 * - MCPWM_INTFLAG_MAT2: Match interrupt for channel (2) 00462 * - MCPWM_INTFLAG_CAP2: Capture interrupt for channel (2) 00463 * - MCPWM_INTFLAG_ABORT: Fast abort interrupt 00464 * @return None 00465 **********************************************************************/ 00466 FlagStatus MCPWM_GetIntStatus(LPC_MCPWM_TypeDef *MCPWMx, uint32_t ulIntType) 00467 { 00468 return ((MCPWMx->MCINTFLAG & ulIntType) ? SET : RESET); 00469 } 00470 00471 /** 00472 * @} 00473 */ 00474 00475 #endif /* _MCPWM */ 00476 00477 /** 00478 * @} 00479 */ 00480 00481 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
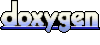