
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc17xx_dac.c
00001 /** 00002 * @file : lpc17xx_dac.c 00003 * @brief : Contains all functions support for DAC firmware library on LPC17xx 00004 * @version : 1.0 00005 * @date : 3. April. 2009 00006 * @author : HieuNguyen 00007 ************************************************************************** 00008 * Software that is described herein is for illustrative purposes only 00009 * which provides customers with programming information regarding the 00010 * products. This software is supplied "AS IS" without any warranties. 00011 * NXP Semiconductors assumes no responsibility or liability for the 00012 * use of the software, conveys no license or title under any patent, 00013 * copyright, or mask work right to the product. NXP Semiconductors 00014 * reserves the right to make changes in the software without 00015 * notification. NXP Semiconductors also make no representation or 00016 * warranty that such application will be suitable for the specified 00017 * use without further testing or modification. 00018 **********************************************************************/ 00019 00020 /* Peripheral group ----------------------------------------------------------- */ 00021 /** @addtogroup DAC 00022 * @{ 00023 */ 00024 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_dac.h" 00027 #include "lpc17xx_clkpwr.h" 00028 00029 /* If this source file built with example, the LPC17xx FW library configuration 00030 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00031 * otherwise the default FW library configuration file must be included instead 00032 */ 00033 #ifdef __BUILD_WITH_EXAMPLE__ 00034 #include "lpc17xx_libcfg.h" 00035 #else 00036 #include "lpc17xx_libcfg_default.h" 00037 #endif /* __BUILD_WITH_EXAMPLE__ */ 00038 00039 00040 #ifdef _DAC 00041 00042 /* Public Functions ----------------------------------------------------------- */ 00043 /** @addtogroup DAC_Public_Functions 00044 * @{ 00045 */ 00046 00047 /*********************************************************************//** 00048 * @brief Initial ADC configuration 00049 * - Maximum current is 700 uA 00050 * - Value to AOUT is 0 00051 * @param[in] DACx pointer to LPC_DAC_TypeDef 00052 * @return None 00053 ***********************************************************************/ 00054 void DAC_Init(LPC_DAC_TypeDef *DACx) 00055 { 00056 CHECK_PARAM(PARAM_DACx(DACx)); 00057 /* Set default clock divider for DAC */ 00058 // CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_DAC, CLKPWR_PCLKSEL_CCLK_DIV_4); 00059 //Set maximum current output 00060 DAC_SetBias(LPC_DAC,DAC_MAX_CURRENT_700uA ); 00061 00062 00063 } 00064 00065 /*********************************************************************//** 00066 * @brief Update value to DAC 00067 * @param[in] DACx pointer to LPC_DAC_TypeDef 00068 * @param[in] dac_value : value 10 bit to be converted to output 00069 * @return None 00070 ***********************************************************************/ 00071 void DAC_UpdateValue (LPC_DAC_TypeDef *DACx,uint32_t dac_value) 00072 { 00073 uint32_t tmp; 00074 CHECK_PARAM(PARAM_DACx(DACx)); 00075 tmp = DACx->DACR & DAC_BIAS_EN; 00076 tmp |= DAC_VALUE(dac_value); 00077 //DACx->DACR &= ~DAC_VALUE(0x3FF); 00078 //DACx->DACR |= DAC_VALUE(dac_value); 00079 // Update value 00080 DACx->DACR = tmp; 00081 } 00082 00083 /*********************************************************************//** 00084 * @brief Set Maximum current for DAC 00085 * @param[in] DACx pointer to LPC_DAC_TypeDef 00086 * @param[in] bias : 0 is 700 uA 00087 * 1 350 uA 00088 * @return None 00089 ***********************************************************************/ 00090 void DAC_SetBias (LPC_DAC_TypeDef *DACx,uint32_t bias) 00091 { 00092 CHECK_PARAM(PARAM_DAC_CURRENT_OPT(bias)); 00093 DACx->DACR &=~DAC_BIAS_EN; 00094 if (bias == DAC_MAX_CURRENT_350uA ) 00095 { 00096 DACx->DACR |= DAC_BIAS_EN; 00097 } 00098 } 00099 /*********************************************************************//** 00100 * @brief To enable the DMA operation and control DMA timer 00101 * @param[in] DACx pointer to LPC_DAC_TypeDef 00102 * @param[in] DAC_ConverterConfigStruct pointer to DAC_CONVERTER_CFG_Type 00103 * - DBLBUF_ENA : enable/disable DACR double buffering feature 00104 * - CNT_ENA : enable/disable timer out counter 00105 * - DMA_ENA : enable/disable DMA access 00106 * @return None 00107 ***********************************************************************/ 00108 void DAC_ConfigDAConverterControl (LPC_DAC_TypeDef *DACx,DAC_CONVERTER_CFG_Type *DAC_ConverterConfigStruct) 00109 { 00110 CHECK_PARAM(PARAM_DACx(DACx)); 00111 DACx->DACCTRL &= ~DAC_DACCTRL_MASK; 00112 if (DAC_ConverterConfigStruct->DBLBUF_ENA) 00113 DACx->DACCTRL |= DAC_DBLBUF_ENA; 00114 if (DAC_ConverterConfigStruct->CNT_ENA ) 00115 DACx->DACCTRL |= DAC_CNT_ENA; 00116 if (DAC_ConverterConfigStruct->DMA_ENA ) 00117 DACx->DACCTRL |= DAC_DMA_ENA; 00118 } 00119 /*********************************************************************//** 00120 * @brief Set reload value for interrupt/DMA counter 00121 * @param[in] DACx pointer to LPC_DAC_TypeDef 00122 * @param[in] time_out time out to reload for interrupt/DMA counter 00123 * @return None 00124 ***********************************************************************/ 00125 void DAC_SetDMATimeOut(LPC_DAC_TypeDef *DACx, uint32_t time_out) 00126 { 00127 CHECK_PARAM(PARAM_DACx(DACx)); 00128 DACx->DACCNTVAL = DAC_CCNT_VALUE(time_out); 00129 } 00130 00131 /** 00132 * @} 00133 */ 00134 00135 #endif /* _DAC */ 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
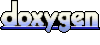