Mbed port of the Simple Plain Xml parser. See http://code.google.com/p/spxml/ for more details. This library uses less memory and is much better suited to streaming data than TinyXML (doesn\'t use as much C++ features, and especially works without streams). See http://mbed.org/users/hlipka/notebook/xml-parsing/ for usage examples.
Dependents: spxmltest_weather VFD_fontx2_weather weather_LCD_display News_LCD_display ... more
spxmlutils.hpp
00001 /* 00002 * Copyright 2007 Stephen Liu 00003 * LGPL, see http://code.google.com/p/spxml/ 00004 * For license terms, see the file COPYING along with this library. 00005 */ 00006 00007 #ifndef __spxmlutils_hpp__ 00008 #define __spxmlutils_hpp__ 00009 00010 #include <stdio.h> 00011 00012 typedef struct tagSP_XmlArrayListNode SP_XmlArrayListNode_t; 00013 00014 class SP_XmlArrayList { 00015 public: 00016 static const int LAST_INDEX; 00017 00018 SP_XmlArrayList( int initCount = 2 ); 00019 virtual ~SP_XmlArrayList(); 00020 00021 int getCount() const; 00022 int append( void * value ); 00023 const void * getItem( int index ) const; 00024 void * takeItem( int index ); 00025 void sort( int ( * cmpFunc )( const void *, const void * ) ); 00026 00027 private: 00028 SP_XmlArrayList( SP_XmlArrayList & ); 00029 SP_XmlArrayList & operator=( SP_XmlArrayList & ); 00030 00031 int mMaxCount; 00032 int mCount; 00033 void ** mFirst; 00034 }; 00035 00036 class SP_XmlQueue { 00037 public: 00038 SP_XmlQueue(); 00039 virtual ~SP_XmlQueue(); 00040 00041 void push( void * item ); 00042 void * pop(); 00043 void * top(); 00044 00045 private: 00046 void ** mEntries; 00047 unsigned int mHead; 00048 unsigned int mTail; 00049 unsigned int mCount; 00050 unsigned int mMaxCount; 00051 }; 00052 00053 class SP_XmlStringBuffer { 00054 public: 00055 SP_XmlStringBuffer(); 00056 virtual ~SP_XmlStringBuffer(); 00057 int append( char c ); 00058 int append( const char * value, int size = 0 ); 00059 int getSize() const; 00060 const char * getBuffer() const; 00061 char * takeBuffer(); 00062 void clean(); 00063 00064 private: 00065 SP_XmlStringBuffer( SP_XmlStringBuffer & ); 00066 SP_XmlStringBuffer & operator=( SP_XmlStringBuffer & ); 00067 00068 void init(); 00069 00070 char * mBuffer; 00071 int mMaxSize; 00072 int mSize; 00073 }; 00074 00075 #ifdef WIN32 00076 00077 #define snprintf _snprintf 00078 #define strncasecmp strnicmp 00079 #define strcasecmp stricmp 00080 #endif 00081 00082 #endif 00083
Generated on Sun Jul 17 2022 09:10:11 by
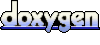