
LAN(Wi-Fi) air controller through the Internet. Also you can use TANK. See:http://wizard.nestegg.jp/lanir.html
Dependencies: EthernetNetIf mbed HTTPServer
main.cpp
00001 ////////////////////////////////////////////////////////// 00002 //Nest Egg Inc. // 00003 //Wi-Fi TANK with Air conditioner // 00004 // // 00005 //SD card module must be Normal chip select mode. // 00006 //Do not use wwChipSelect.cpp & wwChipSelect.h // 00007 //White Wizard Chip Select setting must be // 00008 //invalidated. // 00009 ////////////////////////////////////////////////////////// 00010 00011 #include "mbed.h" 00012 #include "EthernetNetIf.h" 00013 #include "HTTPServer.h" 00014 #include "RPCFunction.h" 00015 00016 #include "string.h" 00017 #include "stdio.h" 00018 00019 //SD----------------------- 00020 #include "SDFileSystem.h" 00021 00022 //semaphore---------------- 00023 #include "Semaphore.h" 00024 00025 #define SDch 0x05 00026 #define MAXdatanum 500 00027 #define TimeLimit 3000 00028 #define pul38khz 26 00029 #define pul38k_ON 13 00030 #define pul38k_OFF 26 00031 00032 #define test 1 00033 00034 //semaphore---------------- 00035 Semaphore sem; 00036 volatile char shared_resource = 'a'; // This is a shared resource for example. 00037 #define LOCK() sem.try_enter() 00038 #define UNLOCK() sem.release() 00039 00040 //prototype 00041 void send_pulse(int, int); 00042 00043 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00044 //------------------------- 00045 00046 //IR----------------------- 00047 PwmOut Pulse_pin(p25); 00048 //------------------------- 00049 00050 DigitalOut led1(LED1); 00051 DigitalOut led2(LED2); 00052 DigitalOut led3(LED3); 00053 DigitalOut led4(LED4); 00054 00055 DigitalOut motor1(p21); 00056 DigitalOut motor2(p22); 00057 DigitalOut motor3(p23); 00058 DigitalOut motor4(p24); 00059 00060 AnalogIn ad19(p19); 00061 AnalogIn ad20(p20); 00062 00063 #if 1 00064 /* 00065 * Use DHCP 00066 */ 00067 EthernetNetIf ethif; 00068 #else 00069 /* 00070 * Use "static IP address" (Parameters:IP, Subnet mask, Gateway, DNS) 00071 */ 00072 EthernetNetIf ethif(IpAddr(xxx,xxx,xxx,xxx), IpAddr(xxx,xxx,xxx,xxx), IpAddr(xxx,xxx,xxx,xxx), IpAddr(xxx,xxx,xxx,xxx)); 00073 #endif 00074 00075 HTTPServer server; 00076 LocalFileSystem local("local"); 00077 void MotorSig(char *input,char *output); 00078 void ReadStatus(char *input,char *output); 00079 RPCFunction rpcFunc(&MotorSig, "MotorSig"); 00080 RPCFunction readrpcFunc(&ReadStatus, "ReadStatus"); 00081 00082 int main(void) { 00083 //Base::add_rpc_class<DigitalOut>(); 00084 //Base::add_rpc_class<AnalogIn>(); 00085 00086 if (ethif.setup()) { 00087 error("Ethernet setup failed."); 00088 return 1; 00089 } 00090 00091 IpAddr ethIp=ethif.getIp(); 00092 00093 led1=1; 00094 wait(1); 00095 server.addHandler<SimpleHandler>("/hello"); 00096 server.addHandler<RPCHandler>("/rpc"); 00097 FSHandler::mount("/local", "/"); 00098 server.addHandler<FSHandler>("/"); 00099 server.bind(80); 00100 while (1) { 00101 Net::poll(); 00102 } 00103 00104 } 00105 00106 00107 void MotorSig(char *input , char *output) 00108 { 00109 //for data----------------------- 00110 int IRdata[MAXdatanum]; 00111 int pulsetime[MAXdatanum]; 00112 int j = 0; 00113 int datanum = 0; 00114 int pHL = 0; 00115 int t = 0; 00116 // FILE *fp1; 00117 00118 //text--------------------------- 00119 int length = 0; 00120 char *str; 00121 char *str1; 00122 char str2[30] = {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}; 00123 char str3[30] = {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}; 00124 00125 //get input data length 00126 for (length = 0; input[length] != '\0'; length++); 00127 00128 //check data 00129 printf("length = %d : input = %s\n", length, input); 00130 00131 //check period of extension 00132 if((length > 4)&&(input[length-4] == '.')){ 00133 //data 00134 //printf("data mode start\n"); 00135 00136 //read file from micro SD card 00137 str1 = "/sd/"; 00138 //printf("%s\n",str1); 00139 for(j = 0; j < length; j++){ 00140 //printf("j=%d input[%d] = %c\n",j,j,input[j]); 00141 str2[j] = input[j]; 00142 } 00143 00144 // printf("str1 = %s\n",str1); 00145 //printf("str2 = %s\n",str2); 00146 00147 strcpy(str3, str1); 00148 //printf("strcpy = %s\n",str3); 00149 00150 strcat(str3, str2); 00151 //printf("strcat = %s\n",str3); 00152 00153 for (length = 0; (str3[length] != '\0'); length++); 00154 00155 FILE *fp1 = fopen(str3, "r"); 00156 //FILE *fp1 = fopen("/sd/off.csv", "r"); 00157 00158 if(fp1 == NULL){ 00159 printf("can not open data file!\n"); 00160 00161 }else{ 00162 printf("loading %s ...\n",str3); 00163 datanum = 0; 00164 00165 fscanf(fp1, "%d\n",&datanum); //read from SD 00166 printf("datanum, %d\n",datanum); 00167 00168 Pulse_pin.period_us(pul38khz); 00169 00170 j=0; 00171 for(j=0;j<datanum;++j){ 00172 fscanf(fp1, "%d,%d,\n", &pHL, &t); 00173 IRdata[j] = pHL; 00174 pulsetime[j] = t; 00175 } 00176 00177 00178 fclose(fp1); 00179 printf("close fp1\n"); 00180 00181 j=0; 00182 if (LOCK()) { 00183 for(j=0;j<datanum;++j){ 00184 send_pulse(IRdata[j],pulsetime[j]); 00185 } 00186 UNLOCK(); 00187 printf("WW sent IR data!\n"); 00188 } 00189 00190 Pulse_pin.pulsewidth(pul38k_OFF); 00191 } 00192 00193 }else{ 00194 //controller 00195 printf("controller mode start\n"); 00196 00197 str = "forward"; 00198 if (strcmp(input,str) == 0){ 00199 //printf("input OK!\n"); 00200 motor1 = 0; 00201 motor2 = 1; 00202 motor3 = 1; 00203 motor4 = 0; 00204 00205 led1 = 0; 00206 led2 = 1; 00207 led3 = 1; 00208 led4 = 0; 00209 } 00210 00211 str = "stop"; 00212 if (strcmp(input,str) == 0){ 00213 //printf("input OK!\n"); 00214 motor1 = 0; 00215 motor2 = 0; 00216 motor3 = 0; 00217 motor4 = 0; 00218 00219 led1 = 0; 00220 led2 = 0; 00221 led3 = 0; 00222 led4 = 0; 00223 } 00224 00225 str = "back"; 00226 if (strcmp(input,str) == 0){ 00227 //printf("input OK!\n"); 00228 motor1 = 1; 00229 motor2 = 0; 00230 motor3 = 0; 00231 motor4 = 1; 00232 00233 led1 = 1; 00234 led2 = 0; 00235 led3 = 0; 00236 led4 = 1; 00237 } 00238 00239 str = "right"; 00240 if (strcmp(input,str) == 0){ 00241 //printf("input OK!\n"); 00242 motor1 = 0; 00243 motor2 = 1; 00244 motor3 = 0; 00245 motor4 = 1; 00246 00247 led1 = 0; 00248 led2 = 1; 00249 led3 = 0; 00250 led4 = 1; 00251 } 00252 00253 str = "left"; 00254 if (strcmp(input,str) == 0){ 00255 //printf("input OK!\n"); 00256 motor1 = 1; 00257 motor2 = 0; 00258 motor3 = 1; 00259 motor4 = 0; 00260 00261 led1 = 1; 00262 led2 = 0; 00263 led3 = 1; 00264 led4 = 0; 00265 } 00266 } 00267 //------------------------------- 00268 } 00269 00270 void ReadStatus(char * input, char * output){ 00271 00272 float ADdata19,ADdata20,temp,humid; 00273 char str[30] = {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0}; 00274 00275 ADdata19 = ad19.read()*3.3; 00276 ADdata20 = ad20.read()*3.3; 00277 00278 temp = 100*(ADdata20-0.5); //temp 00279 humid = (80.0/1.3)*(ADdata19-0.6); //humid 00280 00281 sprintf( str, "T : %.1f <br> H : %.1f", temp, humid); 00282 sprintf(output, str); 00283 } 00284 00285 void send_pulse(int pHL, int t){ 00286 00287 int k = 0; 00288 00289 if(pHL == 0){ 00290 //38kHz pulse on 00291 Pulse_pin.pulsewidth_us(pul38k_ON); 00292 00293 }else if(pHL == 1){ 00294 //38kHz pulse off 00295 Pulse_pin.pulsewidth(pul38k_OFF); 00296 00297 }else{ 00298 Pulse_pin.pulsewidth(pul38k_OFF); 00299 printf("\n\n-----Data format is incorrect!!-----\n pHL = %d, t = %d \n", pHL, t); 00300 00301 } 00302 for(k = 0; k < t; ++k){ 00303 wait_us(50); 00304 } 00305 } 00306 00307
Generated on Tue Jul 19 2022 17:34:41 by
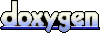