
Trying to make Modbus code into a lib
Embed:
(wiki syntax)
Show/hide line numbers
portserial.cpp
00001 /* 00002 * FreeModbus Libary: BARE Port 00003 * Copyright (C) 2006 Christian Walter <wolti@sil.at> 00004 * 00005 * This library is free software; you can redistribute it and/or 00006 * modify it under the terms of the GNU Lesser General Public 00007 * License as published by the Free Software Foundation; either 00008 * version 2.1 of the License, or (at your option) any later version. 00009 * 00010 * This library is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00013 * Lesser General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU Lesser General Public 00016 * License along with this library; if not, write to the Free Software 00017 * Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00018 * 00019 * File: $Id: portserial.c,v 1.1 2006/08/22 21:35:13 wolti Exp $ 00020 */ 00021 00022 /* ----------------------- System includes ----------------------------------*/ 00023 #include "mbed.h" // Cam 00024 00025 /* ----------------------- Platform includes --------------------------------*/ 00026 #include "port.h" 00027 00028 /* ----------------------- Modbus includes ----------------------------------*/ 00029 #include "mb.h" 00030 #include "mbport.h" 00031 00032 00033 /* ----------------------- static functions ---------------------------------*/ 00034 static void prvvUARTTxReadyISR( void ); 00035 static void prvvUARTRxISR( void ); 00036 static void prvvUARTISR( void ); 00037 00038 /* ----------------------- System Variables ---------------------------------*/ 00039 Serial pc(USBTX, USBRX); // Cam - mbed USB serial port 00040 00041 Ticker simISR; // Cam - mbed ticker 00042 // we don't have the TX buff empty interrupt, so 00043 // we just interrupt every 1 mSec and read RX & TX 00044 // status to simulate the proper ISRs. 00045 00046 static BOOL RxEnable, TxEnable; // Cam - keep a static copy of the RxEnable and TxEnable 00047 // status for the simulated ISR (ticker) 00048 00049 00050 /* ----------------------- Start implementation -----------------------------*/ 00051 // Cam - This is called every 1mS to simulate Rx character received ISR and 00052 // Tx buffer empty ISR. 00053 static void 00054 prvvUARTISR( void ) 00055 { 00056 if (TxEnable) 00057 if(pc.writeable()) 00058 prvvUARTTxReadyISR(); 00059 00060 if (RxEnable) 00061 if(pc.readable()) 00062 prvvUARTRxISR(); 00063 } 00064 00065 void 00066 vMBPortSerialEnable( BOOL xRxEnable, BOOL xTxEnable ) 00067 { 00068 /* If xRXEnable enable serial receive interrupts. If xTxENable enable 00069 * transmitter empty interrupts. 00070 */ 00071 RxEnable = xRxEnable; 00072 TxEnable = xTxEnable; 00073 } 00074 00075 BOOL 00076 xMBPortSerialInit( UCHAR ucPORT, ULONG ulBaudRate, UCHAR ucDataBits, eMBParity eParity ) 00077 { 00078 simISR.attach_us(&prvvUARTISR,1000); // Cam - attach prvvUARTISR to a 1mS ticker to simulate serial interrupt behaviour 00079 // 1mS is just short of a character time at 9600 bps, so quick enough to pick 00080 // up status on a character by character basis. 00081 return TRUE; 00082 } 00083 00084 BOOL 00085 xMBPortSerialPutByte( CHAR ucByte ) 00086 { 00087 /* Put a byte in the UARTs transmit buffer. This function is called 00088 * by the protocol stack if pxMBFrameCBTransmitterEmpty( ) has been 00089 * called. */ 00090 pc.putc( ucByte); 00091 return TRUE; 00092 } 00093 00094 BOOL 00095 xMBPortSerialGetByte( CHAR * pucByte ) 00096 { 00097 /* Return the byte in the UARTs receive buffer. This function is called 00098 * by the protocol stack after pxMBFrameCBByteReceived( ) has been called. 00099 */ 00100 * pucByte = pc.getc(); 00101 return TRUE; 00102 } 00103 00104 /* Create an interrupt handler for the transmit buffer empty interrupt 00105 * (or an equivalent) for your target processor. This function should then 00106 * call pxMBFrameCBTransmitterEmpty( ) which tells the protocol stack that 00107 * a new character can be sent. The protocol stack will then call 00108 * xMBPortSerialPutByte( ) to send the character. 00109 */ 00110 static void prvvUARTTxReadyISR( void ) 00111 { 00112 pxMBFrameCBTransmitterEmpty( ); 00113 } 00114 00115 /* Create an interrupt handler for the receive interrupt for your target 00116 * processor. This function should then call pxMBFrameCBByteReceived( ). The 00117 * protocol stack will then call xMBPortSerialGetByte( ) to retrieve the 00118 * character. 00119 */ 00120 static void prvvUARTRxISR( void ) 00121 { 00122 pxMBFrameCBByteReceived( ); 00123 } 00124 00125
Generated on Tue Jul 12 2022 19:17:06 by
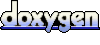