
Trying to make Modbus code into a lib
Embed:
(wiki syntax)
Show/hide line numbers
portevent.cpp
00001 /* 00002 * FreeModbus Libary: BARE Port 00003 * Copyright (C) 2006 Christian Walter <wolti@sil.at> 00004 * 00005 * This library is free software; you can redistribute it and/or 00006 * modify it under the terms of the GNU Lesser General Public 00007 * License as published by the Free Software Foundation; either 00008 * version 2.1 of the License, or (at your option) any later version. 00009 * 00010 * This library is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00013 * Lesser General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU Lesser General Public 00016 * License along with this library; if not, write to the Free Software 00017 * Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00018 * 00019 * File: $Id: portevent.c,v 1.1 2006/08/22 21:35:13 wolti Exp $ 00020 */ 00021 00022 /* ----------------------- Modbus includes ----------------------------------*/ 00023 #include "mb.h" 00024 #include "mbport.h" 00025 00026 /* ----------------------- Variables ----------------------------------------*/ 00027 static eMBEventType eQueuedEvent; 00028 static BOOL xEventInQueue; 00029 00030 /* ----------------------- Start implementation -----------------------------*/ 00031 BOOL 00032 xMBPortEventInit( void ) 00033 { 00034 xEventInQueue = FALSE; 00035 return TRUE; 00036 } 00037 00038 BOOL 00039 xMBPortEventPost( eMBEventType eEvent ) 00040 { 00041 xEventInQueue = TRUE; 00042 eQueuedEvent = eEvent; 00043 return TRUE; 00044 } 00045 00046 BOOL 00047 xMBPortEventGet( eMBEventType * eEvent ) 00048 { 00049 BOOL xEventHappened = FALSE; 00050 00051 if( xEventInQueue ) 00052 { 00053 *eEvent = eQueuedEvent; 00054 xEventInQueue = FALSE; 00055 xEventHappened = TRUE; 00056 } 00057 return xEventHappened; 00058 }
Generated on Tue Jul 12 2022 19:17:06 by
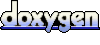