
Trying to make Modbus code into a lib
Embed:
(wiki syntax)
Show/hide line numbers
mbfuncother.cpp
00001 /* 00002 * FreeModbus Libary: A portable Modbus implementation for Modbus ASCII/RTU. 00003 * Copyright (c) 2006 Christian Walter <wolti@sil.at> 00004 * All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 1. Redistributions of source code must retain the above copyright 00010 * notice, this list of conditions and the following disclaimer. 00011 * 2. Redistributions in binary form must reproduce the above copyright 00012 * notice, this list of conditions and the following disclaimer in the 00013 * documentation and/or other materials provided with the distribution. 00014 * 3. The name of the author may not be used to endorse or promote products 00015 * derived from this software without specific prior written permission. 00016 * 00017 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR 00018 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES 00019 * OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00020 * IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, 00021 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 00022 * NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00023 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00024 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00025 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF 00026 * THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00027 * 00028 * File: $Id: mbfuncother.c,v 1.8 2006/12/07 22:10:34 wolti Exp $ 00029 */ 00030 00031 /* ----------------------- System includes ----------------------------------*/ 00032 #include "stdlib.h" 00033 #include "string.h" 00034 00035 /* ----------------------- Platform includes --------------------------------*/ 00036 #include "port.h" 00037 00038 /* ----------------------- Modbus includes ----------------------------------*/ 00039 #include "mb.h" 00040 #include "mbframe.h" 00041 #include "mbproto.h" 00042 #include "mbconfig.h" 00043 00044 #if MB_FUNC_OTHER_REP_SLAVEID_ENABLED > 0 00045 00046 /* ----------------------- Static variables ---------------------------------*/ 00047 static UCHAR ucMBSlaveID[MB_FUNC_OTHER_REP_SLAVEID_BUF]; 00048 static USHORT usMBSlaveIDLen; 00049 00050 /* ----------------------- Start implementation -----------------------------*/ 00051 00052 eMBErrorCode 00053 eMBSetSlaveID( UCHAR ucSlaveID, BOOL xIsRunning, 00054 UCHAR const *pucAdditional, USHORT usAdditionalLen ) 00055 { 00056 eMBErrorCode eStatus = MB_ENOERR ; 00057 00058 /* the first byte and second byte in the buffer is reserved for 00059 * the parameter ucSlaveID and the running flag. The rest of 00060 * the buffer is available for additional data. */ 00061 if( usAdditionalLen + 2 < MB_FUNC_OTHER_REP_SLAVEID_BUF ) 00062 { 00063 usMBSlaveIDLen = 0; 00064 ucMBSlaveID[usMBSlaveIDLen++] = ucSlaveID; 00065 ucMBSlaveID[usMBSlaveIDLen++] = ( UCHAR )( xIsRunning ? 0xFF : 0x00 ); 00066 if( usAdditionalLen > 0 ) 00067 { 00068 memcpy( &ucMBSlaveID[usMBSlaveIDLen], pucAdditional, 00069 ( size_t )usAdditionalLen ); 00070 usMBSlaveIDLen += usAdditionalLen; 00071 } 00072 } 00073 else 00074 { 00075 eStatus = MB_ENORES ; 00076 } 00077 return eStatus; 00078 } 00079 00080 eMBException 00081 eMBFuncReportSlaveID( UCHAR * pucFrame, USHORT * usLen ) 00082 { 00083 memcpy( &pucFrame[MB_PDU_DATA_OFF], &ucMBSlaveID[0], ( size_t )usMBSlaveIDLen ); 00084 *usLen = ( USHORT )( MB_PDU_DATA_OFF + usMBSlaveIDLen ); 00085 return MB_EX_NONE; 00086 } 00087 00088 #endif
Generated on Tue Jul 12 2022 19:17:06 by
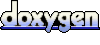