Driver for CC3000 Wi-Fi module
Dependencies: NVIC_set_all_priorities
Dependents: CC3000_Simple_Socket Wi-Go_IOT_Demo
wlan.h
00001 /***************************************************************************** 00002 * 00003 * wlan.h - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 #ifndef __WLAN_H__ 00036 #define __WLAN_H__ 00037 00038 #include "security.h" 00039 00040 //***************************************************************************** 00041 // 00042 //! \addtogroup wlan 00043 //! @{ 00044 // 00045 //***************************************************************************** 00046 00047 /** CC3000 Host driver - WLAN 00048 * 00049 */ 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 #define SMART_CONFIG_PROFILE_SIZE 67 // 67 = 32 (max ssid) + 32 (max key) + 1 (SSID length) + 1 (security type) + 1 (key length) 00056 00057 /* patches type */ 00058 #define PATCHES_HOST_TYPE_WLAN_DRIVER 0x01 00059 #define PATCHES_HOST_TYPE_WLAN_FW 0x02 00060 #define PATCHES_HOST_TYPE_BOOTLOADER 0x03 00061 00062 #define SL_SET_SCAN_PARAMS_INTERVAL_LIST_SIZE (16) 00063 #define SL_SIMPLE_CONFIG_PREFIX_LENGTH (3) 00064 #define ETH_ALEN (6) 00065 #define MAXIMAL_SSID_LENGTH (32) 00066 00067 #define SL_PATCHES_REQUEST_DEFAULT (0) 00068 #define SL_PATCHES_REQUEST_FORCE_HOST (1) 00069 #define SL_PATCHES_REQUEST_FORCE_NONE (2) 00070 00071 00072 #define WLAN_SEC_UNSEC (0) 00073 #define WLAN_SEC_WEP (1) 00074 #define WLAN_SEC_WPA (2) 00075 #define WLAN_SEC_WPA2 (3) 00076 00077 00078 #define WLAN_SL_INIT_START_PARAMS_LEN (1) 00079 #define WLAN_PATCH_PARAMS_LENGTH (8) 00080 #define WLAN_SET_CONNECTION_POLICY_PARAMS_LEN (12) 00081 #define WLAN_DEL_PROFILE_PARAMS_LEN (4) 00082 #define WLAN_SET_MASK_PARAMS_LEN (4) 00083 #define WLAN_SET_SCAN_PARAMS_LEN (100) 00084 #define WLAN_GET_SCAN_RESULTS_PARAMS_LEN (4) 00085 #define WLAN_ADD_PROFILE_NOSEC_PARAM_LEN (24) 00086 #define WLAN_ADD_PROFILE_WEP_PARAM_LEN (36) 00087 #define WLAN_ADD_PROFILE_WPA_PARAM_LEN (44) 00088 #define WLAN_CONNECT_PARAM_LEN (29) 00089 #define WLAN_SMART_CONFIG_START_PARAMS_LEN (4) 00090 00091 00092 /** 00093 * Send HCI_CMND_SIMPLE_LINK_START to CC3000 00094 * @param usPatchesAvailableAtHost flag to indicate if patches available\n 00095 * from host or from EEPROM. Due to the \n 00096 * fact the patches are burn to the EEPROM\n 00097 * using the patch programmer utility, the \n 00098 * patches will be available from the EEPROM\n 00099 * and not from the host.\n 00100 * @return none 00101 */ 00102 static void SimpleLink_Init_Start(unsigned short usPatchesAvailableAtHost); 00103 00104 /** 00105 * Initialize wlan driver 00106 * @param sWlanCB Asynchronous events callback. \n 00107 * 0 no event call back.\n 00108 * -call back parameters:\n 00109 * 1) event_type: HCI_EVNT_WLAN_UNSOL_CONNECT connect event,\n 00110 * HCI_EVNT_WLAN_UNSOL_DISCONNECT disconnect event,\n 00111 * HCI_EVNT_WLAN_ASYNC_SIMPLE_CONFIG_DONE config done,\n 00112 * HCI_EVNT_WLAN_UNSOL_DHCP dhcp report, \n 00113 * HCI_EVNT_WLAN_ASYNC_PING_REPORT ping report OR \n 00114 * HCI_EVNT_WLAN_KEEPALIVE keepalive.\n 00115 * 2) data: pointer to extra data that received by the event\n 00116 * (NULL no data).\n 00117 * 3) length: data length.\n 00118 * -Events with extra data:\n 00119 * HCI_EVNT_WLAN_UNSOL_DHCP: 4 bytes IP, 4 bytes Mask,\n 00120 * 4 bytes default gateway, 4 bytes DHCP server and 4 bytes\n 00121 * for DNS server.\n 00122 * HCI_EVNT_WLAN_ASYNC_PING_REPORT: 4 bytes Packets sent,\n 00123 * 4 bytes Packets received, 4 bytes Min round time, \n 00124 * 4 bytes Max round time and 4 bytes for Avg round time.\n 00125 * @param sFWPatches 0 no patch or pointer to FW patches 00126 * @param sDriverPatches 0 no patch or pointer to driver patches 00127 * @param sBootLoaderPatches 0 no patch or pointer to bootloader patches 00128 * @param sReadWlanInterruptPin init callback. the callback read wlan interrupt status. 00129 * @param sWlanInterruptEnable init callback. the callback enable wlan interrupt. 00130 * @param sWlanInterruptDisable init callback. the callback disable wlan interrupt. 00131 * @param sWriteWlanPin init callback. the callback write value to device pin. 00132 * @return none 00133 * @sa wlan_set_event_mask , wlan_start , wlan_stop 00134 * @warning This function must be called before ANY other wlan driver function 00135 */ 00136 void wlan_init(tWlanCB sWlanCB, 00137 tFWPatches sFWPatches, 00138 tDriverPatches sDriverPatches, 00139 tBootLoaderPatches sBootLoaderPatches, 00140 tWlanReadInteruptPin sReadWlanInterruptPin, 00141 tWlanInterruptEnable sWlanInterruptEnable, 00142 tWlanInterruptDisable sWlanInterruptDisable, 00143 tWriteWlanPin sWriteWlanPin); 00144 00145 /** 00146 * Trigger Received event/data processing - called from the SPI library to receive the data 00147 * @param pvBuffer - pointer to the received data buffer\n 00148 * The function triggers Received event/data processing\n 00149 * @param Pointer to the received data 00150 * @return none 00151 */ 00152 void SpiReceiveHandler(void *pvBuffer); 00153 00154 00155 /** 00156 * Start WLAN device. 00157 * This function asserts the enable pin of \n 00158 * the device (WLAN_EN), starting the HW initialization process.\n 00159 * The function blocked until device Initialization is completed.\n 00160 * Function also configure patches (FW, driver or bootloader) \n 00161 * and calls appropriate device callbacks.\n 00162 * @param usPatchesAvailableAtHost - flag to indicate if patches available\n 00163 * from host or from EEPROM. Due to the\n 00164 * fact the patches are burn to the EEPROM\n 00165 * using the patch programmer utility, the \n 00166 * patches will be available from the EEPROM\n 00167 * and not from the host.\n 00168 * @return none 00169 * @Note Prior calling the function wlan_init shall be called. 00170 * @Warning This function must be called after wlan_init and before any other wlan API 00171 * @sa wlan_init , wlan_stop 00172 */ 00173 extern void wlan_start(unsigned short usPatchesAvailableAtHost); 00174 00175 /** 00176 * Stop WLAN device by putting it into reset state. 00177 * @param none 00178 * @return none 00179 * @sa wlan_start 00180 */ 00181 extern void wlan_stop(void); 00182 00183 /** 00184 * Connect to AP 00185 * @param sec_type security options:\n 00186 * WLAN_SEC_UNSEC, \n 00187 * WLAN_SEC_WEP (ASCII support only),\n 00188 * WLAN_SEC_WPA or WLAN_SEC_WPA2\n 00189 * @param ssid up to 32 bytes and is ASCII SSID of the AP 00190 * @param ssid_len length of the SSID 00191 * @param bssid 6 bytes specified the AP bssid 00192 * @param key up to 16 bytes specified the AP security key 00193 * @param key_len key length 00194 * @return On success, zero is returned. On error, negative is returned.\n 00195 * Note that even though a zero is returned on success to trigger\n 00196 * connection operation, it does not mean that CCC3000 is already\n 00197 * connected. An asynchronous "Connected" event is generated when \n 00198 * actual association process finishes and CC3000 is connected to\n 00199 * the AP. If DHCP is set, An asynchronous "DHCP" event is \n 00200 * generated when DHCP process is finish.\n 00201 * @warning Please Note that when connection to AP configured with security\n 00202 * type WEP, please confirm that the key is set as ASCII and not as HEX.\n 00203 * @sa wlan_disconnect 00204 */ 00205 #ifndef CC3000_TINY_DRIVER 00206 extern long wlan_connect(unsigned long ulSecType, 00207 char *ssid, 00208 long ssid_len, 00209 unsigned char *bssid, 00210 unsigned char *key, 00211 long key_len); 00212 #else 00213 extern long wlan_connect(char *ssid, long ssid_len); 00214 00215 #endif 00216 00217 /** 00218 * Disconnect connection from AP. 00219 * @return 0 disconnected done, other CC3000 already disconnected 00220 * @sa wlan_connect 00221 */ 00222 extern long wlan_disconnect(void); 00223 00224 /** 00225 * Add profile. 00226 * When auto start is enabled, the device connects to\n 00227 * station from the profiles table. Up to 7 profiles are supported. \n 00228 * If several profiles configured the device choose the highest \n 00229 * priority profile, within each priority group, device will choose \n 00230 * profile based on security policy, signal strength, etc \n 00231 * parameters. All the profiles are stored in CC3000 NVMEM.\n 00232 * 00233 * @param ulSecType WLAN_SEC_UNSEC,WLAN_SEC_WEP,WLAN_SEC_WPA,WLAN_SEC_WPA2 00234 * @param ucSsid ssid SSID up to 32 bytes 00235 * @param ulSsidLen ssid length 00236 * @param ucBssid bssid 6 bytes 00237 * @param ulPriority ulPriority profile priority. Lowest priority:0. 00238 * @param ulPairwiseCipher_Or_TxKeyLen key length for WEP security 00239 * @param ulGroupCipher_TxKeyIndex key index 00240 * @param ulKeyMgmt KEY management 00241 * @param ucPf_OrKey security key 00242 * @param ulPassPhraseLen security key length for WPA\WPA2 00243 * @return On success, zero is returned. On error, -1 is returned 00244 * @sa wlan_ioctl_del_profile 00245 */ 00246 extern long wlan_add_profile(unsigned long ulSecType, 00247 unsigned char* ucSsid, 00248 unsigned long ulSsidLen, 00249 unsigned char *ucBssid, 00250 unsigned long ulPriority, 00251 unsigned long ulPairwiseCipher_Or_Key, 00252 unsigned long ulGroupCipher_TxKeyLen, 00253 unsigned long ulKeyMgmt, 00254 unsigned char* ucPf_OrKey, 00255 unsigned long ulPassPhraseLen); 00256 00257 /** 00258 * Delete WLAN profile. 00259 * @param index number of profile to delete 00260 * @return On success, zero is returned. On error, -1 is returned 00261 * @Note In order to delete all stored profile, set index to 255. 00262 * @sa wlan_add_profile 00263 */ 00264 extern long wlan_ioctl_del_profile(unsigned long ulIndex); 00265 00266 /** 00267 * Mask event according to bit mask. 00268 * In case that event is masked (1), the device will not send the masked event to host. 00269 * @param mask mask option:\n 00270 * HCI_EVNT_WLAN_UNSOL_CONNECT connect event\n 00271 * HCI_EVNT_WLAN_UNSOL_DISCONNECT disconnect event\n 00272 * HCI_EVNT_WLAN_ASYNC_SIMPLE_CONFIG_DONE smart config done\n 00273 * HCI_EVNT_WLAN_UNSOL_INIT init done\n 00274 * HCI_EVNT_WLAN_UNSOL_DHCP dhcp event report\n 00275 * HCI_EVNT_WLAN_ASYNC_PING_REPORT ping report\n 00276 * HCI_EVNT_WLAN_KEEPALIVE keepalive\n 00277 * HCI_EVNT_WLAN_TX_COMPLETE - disable information on end of transmission\n 00278 * Saved: no. 00279 * @return On success, zero is returned. On error, -1 is returned 00280 */ 00281 extern long wlan_set_event_mask(unsigned long ulMask); 00282 00283 /** 00284 * Get wlan status: disconnected, scanning, connecting or connected 00285 * @param none 00286 * @return WLAN_STATUS_DISCONNECTED, WLAN_STATUS_SCANING,\n 00287 * STATUS_CONNECTING or WLAN_STATUS_CONNECTED \n 00288 */ 00289 extern long wlan_ioctl_statusget(void); 00290 00291 00292 /** 00293 * Set connection policy. 00294 * When auto is enabled, the device tries to connect according the following policy:\n 00295 * 1) If fast connect is enabled and last connection is valid, \n 00296 * the device will try to connect to it without the scanning \n 00297 * procedure (fast). The last connection will be marked as\n 00298 * invalid, due to adding/removing profile. \n 00299 * 2) If profile exists, the device will try to connect it \n 00300 * (Up to seven profiles).\n 00301 * 3) If fast and profiles are not found, and open mode is\n 00302 * enabled, the device will try to connect to any AP.\n 00303 * Note that the policy settings are stored in the CC3000 NVMEM.\n 00304 * @param should_connect_to_open_ap enable(1), disable(0) connect to any \n 00305 * available AP. This parameter corresponds to the configuration of \n 00306 * item # 3 in the brief description.\n 00307 * @param should_use_fast_connect enable(1), disable(0). if enabled, tries \n 00308 * to connect to the last connected AP. This parameter corresponds \n 00309 * to the configuration of item # 1 in the brief description.\n 00310 * @param auto_start enable(1), disable(0) auto connect \n 00311 * after reset and periodically reconnect if needed. This \n 00312 * configuration configures option 2 in the above description.\n 00313 * @return On success, zero is returned.\n 00314 * On error, -1 is returned \n 00315 * @sa wlan_add_profile , wlan_ioctl_del_profile 00316 */ 00317 extern long wlan_ioctl_set_connection_policy(unsigned long should_connect_to_open_ap, 00318 unsigned long should_use_fast_connect, 00319 unsigned long ulUseProfiles); 00320 00321 /** 00322 * Get entry from scan result table. 00323 * The scan results are returned one by one, and each entry represents a single AP found in the area.\n 00324 * The following is a format of the scan result: \n 00325 * - 4 Bytes: number of networks found\n 00326 * - 4 Bytes: The status of the scan: 0 - aged results, 1 - results valid, 2 - no results\n 00327 * - 42 bytes: Result entry, where the bytes are arranged as follows:\n 00328 * - 1 bit isValid - is result valid or not\n 00329 * - 7 bits rssi - RSSI value \n 00330 * - 2 bits securityMode - security mode of the AP: 0 - Open, 1 - WEP, 2 WPA, 3 WPA2\n 00331 * - 6 bits SSID name length\n 00332 * - 2 bytes the time at which the entry has entered into scans result table\n 00333 * - 32 bytes SSID name\n 00334 * - 6 bytes BSSID \n 00335 * @param[in] scan_timeout parameter not supported 00336 * @param[out] ucResults scan result (_wlan_full_scan_results_args_t) 00337 * @return On success, zero is returned. On error, -1 is returned 00338 * @Note scan_timeout, is not supported on this version. 00339 * @sa wlan_ioctl_set_scan_params 00340 */ 00341 extern long wlan_ioctl_get_scan_results(unsigned long ulScanTimeout, 00342 unsigned char *ucResults); 00343 00344 /** 00345 * start and stop scan procedure. Set scan parameters. 00346 * @param uiEnable start/stop application scan: \n 00347 * 1 = start scan with default interval value of 10 min. \n 00348 * To set a different scan interval value, apply the value in milliseconds.\n 00349 * minimum = 1 second. 0=stop.\n 00350 * Wlan reset (wlan_stop() wlan_start()) is needed when changing scan interval value.\n 00351 * Saved: No\n 00352 * @param uiMinDwellTime minimum dwell time value to be used for each channel, in milliseconds.\n 00353 * Saved: yes\n 00354 * Recommended Value: 100\n 00355 * Default: 20\n 00356 * @param uiMaxDwellTime maximum dwell time value to be used for each channel, in milliseconds.\n 00357 * Saved: yes\n 00358 * Recommended Value: 100\n 00359 * Default: 30\n 00360 * @param uiNumOfProbeRequests max probe request between dwell time. \n 00361 * Saved: yes.\n 00362 * Recommended Value: 5\n 00363 * Default:2\n 00364 * @param uiChannelMask bitwise, up to 13 channels (0x1fff). \n 00365 * Saved: yes.\n 00366 * Default: 0x7ff\n 00367 * @param uiRSSIThreshold RSSI threshold.\n 00368 * Saved: yes\n 00369 * Default: -80\n 00370 * @param uiSNRThreshold NSR threshold.\n 00371 * Saved: yes\n 00372 * Default: 0\n 00373 * @param uiDefaultTxPower probe Tx power.\n 00374 * Saved: yes\n 00375 * Default: 205\n 00376 * @param aiIntervalList pointer to array with 16 entries (16 channels) \n 00377 * Each entry (unsigned long) holds timeout between periodic scan \n 00378 * and connection scan - in milliseconds.\n 00379 * Saved: yes.\n 00380 * Default 2000ms.\n 00381 * @return On success, zero is returned. On error, -1 is returned 00382 * @Note uiDefaultTxPower, is not supported on this version. 00383 * @sa wlan_ioctl_get_scan_results 00384 */ 00385 extern long wlan_ioctl_set_scan_params(unsigned long uiEnable, 00386 unsigned long uiMinDwellTime, 00387 unsigned long uiMaxDwellTime, 00388 unsigned long uiNumOfProbeRequests, 00389 unsigned long uiChannelMask, 00390 long iRSSIThreshold, 00391 unsigned long uiSNRThreshold, 00392 unsigned long uiDefaultTxPower, 00393 unsigned long *aiIntervalList); 00394 00395 00396 /** 00397 * Start to acquire device profile. 00398 * The device will acquires its own profile if a profile message is found.\n 00399 * The acquired AP information is stored in CC3000 EEPROM only when AES128 encryption is used.\n 00400 * When AES128 encryption is not used, a profile is internally created by the device.\n 00401 * @param algoEncryptedFlag indicates whether the information is encrypted 00402 * @return On success, zero is returned. On error, -1 is returned 00403 * @Note An asynchronous event - Smart Config Done will be generated as soon\n 00404 * as the process finishes successfully.\n 00405 * @sa wlan_smart_config_set_prefix , wlan_smart_config_stop 00406 */ 00407 extern long wlan_smart_config_start(unsigned long algoEncryptedFlag); 00408 00409 /** 00410 * Stop the acquire profile procedure 00411 * @param algoEncryptedFlag indicates whether the information is encrypted 00412 * @return On success, zero is returned. On error, -1 is returned 00413 * @sa wlan_smart_config_start , wlan_smart_config_set_prefix 00414 */ 00415 extern long wlan_smart_config_stop(void); 00416 00417 /** 00418 * Configure station ssid prefix. 00419 * The prefix is used internally in CC3000. It should always be TTT.\n 00420 * @param newPrefix 3 bytes identify the SSID prefix for the Smart Config. 00421 * @return On success, zero is returned. On error, -1 is returned 00422 * @Note The prefix is stored in CC3000 NVMEM 00423 * @sa wlan_smart_config_start , wlan_smart_config_stop 00424 */ 00425 extern long wlan_smart_config_set_prefix(char* cNewPrefix); 00426 00427 /** 00428 * Process the acquired data and store it as a profile. 00429 * The acquired AP information is stored in CC3000 EEPROM encrypted. 00430 * The encrypted data is decrypted and stored as a profile.\n 00431 * behavior is as defined by connection policy.\n 00432 * @param none 00433 * @return On success, zero is returned. On error, -1 is returned 00434 */ 00435 extern long wlan_smart_config_process(void); 00436 00437 #ifdef __cplusplus 00438 } 00439 #endif // __cplusplus 00440 00441 //***************************************************************************** 00442 // 00443 // Close the Doxygen group. 00444 //! @} 00445 // 00446 //***************************************************************************** 00447 00448 #endif // __WLAN_H__
Generated on Wed Jul 13 2022 18:31:31 by
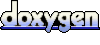