Driver for CC3000 Wi-Fi module
Dependencies: NVIC_set_all_priorities
Dependents: CC3000_Simple_Socket Wi-Go_IOT_Demo
nvmem.h
00001 /***************************************************************************** 00002 * 00003 * nvmem.h - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 #ifndef __NVRAM_H__ 00036 #define __NVRAM_H__ 00037 00038 #include "cc3000_spi_hci.h" 00039 00040 //***************************************************************************** 00041 // 00042 //! \addtogroup nvmem 00043 //! @{ 00044 // 00045 //***************************************************************************** 00046 00047 /** CC3000 Host driver - NVMEM 00048 * 00049 */ 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 //***************************************************************************** 00056 // 00057 // Prototypes for the structures for APIs. 00058 // 00059 //***************************************************************************** 00060 00061 #define NVMEM_READ_PARAMS_LEN (12) 00062 #define NVMEM_CREATE_PARAMS_LEN (8) 00063 #define NVMEM_WRITE_PARAMS_LEN (16) 00064 00065 00066 /**************************************************************************** 00067 ** 00068 ** Definitions for File IDs 00069 ** 00070 ****************************************************************************/ 00071 /* --------------------------------------------------------- EEPROM FAT table --------------------------------------------------------- 00072 00073 File ID Offset File Size Used Size Parameter 00074 # ID address (bytes) (bytes) 00075 -------------------------------------------------------------------------------------------------------------------------------------- 00076 0 NVMEM_NVS_FILEID 0x50 0x1A0 0x1A RF Calibration results table(generated automatically by TX Bip) 00077 1 NVMEM_NVS_SHADOW_FILEID 0x1F0 0x1A0 0x1A NVS Shadow 00078 2 NVMEM_WLAN_CONFIG_FILEID 0x390 0x1000 0x64 WLAN configuration 00079 3 NVMEM_WLAN_CONFIG_SHADOW_FILEID 0x1390 0x1000 0x64 WLAN configuration shadow 00080 4 NVMEM_WLAN_DRIVER_SP_FILEID 0x2390 0x2000 variable WLAN Driver ROM Patches 00081 5 NVMEM_WLAN_FW_SP_FILEID 0x4390 0x2000 variable WLAN FW Patches 00082 6 NVMEM_MAC_FILEID 0x6390 0x10 0x10 6 bytes of MAC address 00083 7 NVMEM_FRONTEND_VARS_FILEID 0x63A0 0x10 0x10 Frontend Vars 00084 8 NVMEM_IP_CONFIG_FILEID 0x63B0 0x40 0x40 IP configuration 00085 9 NVMEM_IP_CONFIG_SHADOW_FILEID 0x63F0 0x40 0x40 IP configuration shadow 00086 10 NVMEM_BOOTLOADER_SP_FILEID 0x6430 0x400 variable Bootloader Patches 00087 11 NVMEM_RM_FILEID 0x6830 0x200 0x7F Radio parameters 00088 12 NVMEM_AES128_KEY_FILEID 0x6A30 0x10 0x10 AES128 key file 00089 13 NVMEM_SHARED_MEM_FILEID 0x6A40 0x50 0x44 Host-CC3000 shared memory file 00090 14 NVMEM_USER_FILE_1_FILEID 0x6A90 variable variable 1st user file 00091 15 NVMEM_USER_FILE_2_FILEID variable variable variable 2nd user file 00092 */ 00093 /* NVMEM file ID - system files*/ 00094 #define NVMEM_NVS_FILEID (0) 00095 #define NVMEM_NVS_SHADOW_FILEID (1) 00096 #define NVMEM_WLAN_CONFIG_FILEID (2) 00097 #define NVMEM_WLAN_CONFIG_SHADOW_FILEID (3) 00098 #define NVMEM_WLAN_DRIVER_SP_FILEID (4) 00099 #define NVMEM_WLAN_FW_SP_FILEID (5) 00100 #define NVMEM_MAC_FILEID (6) 00101 #define NVMEM_FRONTEND_VARS_FILEID (7) 00102 #define NVMEM_IP_CONFIG_FILEID (8) 00103 #define NVMEM_IP_CONFIG_SHADOW_FILEID (9) 00104 #define NVMEM_BOOTLOADER_SP_FILEID (10) 00105 #define NVMEM_RM_FILEID (11) 00106 00107 /* NVMEM file ID - user files*/ 00108 #define NVMEM_AES128_KEY_FILEID (12) 00109 #define NVMEM_SHARED_MEM_FILEID (13) 00110 #define NVMEM_USER_FILE_1_FILEID (14) 00111 #define NVMEM_USER_FILE_2_FILEID (15) 00112 00113 /* max entry in order to invalid nvmem */ 00114 #define NVMEM_MAX_ENTRY (16) 00115 00116 00117 /** 00118 * Read 'length' data at offset 'ulOffset' from nvmem to file 'ulFileId'. 00119 * @param ulFileId Possible nvmem file id values:\n 00120 * NVMEM_NVS_FILEID, NVMEM_NVS_SHADOW_FILEID,\n 00121 * NVMEM_WLAN_CONFIG_FILEID, NVMEM_WLAN_CONFIG_SHADOW_FILEID,\n 00122 * NVMEM_WLAN_DRIVER_SP_FILEID, NVMEM_WLAN_FW_SP_FILEID,\n 00123 * NVMEM_MAC_FILEID, NVMEM_FRONTEND_VARS_FILEID,\n 00124 * NVMEM_IP_CONFIG_FILEID, NVMEM_IP_CONFIG_SHADOW_FILEID,\n 00125 * NVMEM_BOOTLOADER_SP_FILEID, NVMEM_RM_FILEID,\n 00126 * and user files 12-15.\n 00127 * @param ulLength number of bytes to read 00128 * @param ulOffset ulOffset in file from where to read 00129 * @param buff output buffer pointer 00130 * @return number of bytes read, otherwise error.\n 00131 * Error conditions : file can't be used\n 00132 * file is invalid\n 00133 * read out of bounds. \n 00134 */ 00135 extern signed long nvmem_read(unsigned long file_id, unsigned long length, unsigned long offset, unsigned char *buff); 00136 00137 /** 00138 * Write 'length' data at offset 'ulOffset' from file 'ulFileId' to nvmem. 00139 * The file id is marked as invalid until writing is ended.\n 00140 * The file entry doesn't need to be valid - only allocated.\n 00141 * @param ulFileId Possible nvmem file id values:\n 00142 * NVMEM_WLAN_DRIVER_SP_FILEID, NVMEM_WLAN_FW_SP_FILEID,\n 00143 * NVMEM_MAC_FILEID, NVMEM_BOOTLOADER_SP_FILEID,\n 00144 * and user files 12-15.\n 00145 * @param ulLength number of bytes to write 00146 * @param ulEntryOffset offset in file to start write operation from 00147 * @param buff data to write 00148 * 00149 * @return 0 on success, error otherwise. 00150 */ 00151 extern signed long nvmem_write(unsigned long ulFileId, unsigned long ulLength, unsigned long ulEntryOffset, unsigned char *buff); 00152 00153 00154 /** 00155 * Write the MAC address to EEPROM (OUI first) 00156 * @param mac mac address to be set 00157 * @return 0 on success, error otherwise. 00158 */ 00159 extern unsigned char nvmem_set_mac_address(unsigned char *mac); 00160 00161 00162 /** 00163 * Read the MAC address from EEPROM (OUI first) 00164 * @param[out] mac mac address 00165 * 00166 * @return on success 0, error otherwise. 00167 */ 00168 extern unsigned char nvmem_get_mac_address(unsigned char *mac); 00169 00170 00171 /** 00172 * Write patch code to a specific file ID. Each write contains SP_PORTION_SIZE bytes. 00173 * @param ulFileId Possible nvmem file id values:\n 00174 * NVMEM_WLAN_DRIVER_SP_FILEID, NVMEM_WLAN_FW_SP_FILEID,\n 00175 * @param spLength number of bytes to write 00176 * @param spData SP data to write 00177 * 00178 * @return 0 on success, error otherwise. 00179 */ 00180 extern unsigned char nvmem_write_patch(unsigned long ulFileId, unsigned long spLength, const unsigned char *spData); 00181 00182 /** 00183 * Read the patch version. 00184 * Read package version (WiFi FW patch, driver-supplicant-NS patch, bootloader patch)\n 00185 * @param[out] patchVer first number indicates package ID and the second number indicates package build number 00186 * @return 0 on success, error otherwise. 00187 */ 00188 #ifndef CC3000_TINY_DRIVER 00189 extern unsigned char nvmem_read_sp_version(unsigned char* patchVer); 00190 #endif 00191 00192 /** 00193 * Create a new file entry and allocate space in NVMEM. 00194 * Applies only to user files.\n 00195 * Modify the size of file.\n 00196 * If the entry is unallocated - allocate it to size ulNewLen (marked invalid).\n 00197 * If it is allocated then deallocate it first.\n 00198 * To just mark the file as invalid without resizing - set ulNewLen=0.\n 00199 * @param ulFileId Possible nvmem file Ids:\n 00200 * * NVMEM_AES128_KEY_FILEID: 12\n 00201 * * NVMEM_SHARED_MEM_FILEID: 13\n 00202 * * and fileIDs 14 and 15\n 00203 * @param ulNewLen entry ulLength 00204 * 00205 * @return 0 on success, error otherwise. 00206 */ 00207 extern signed long nvmem_create_entry(unsigned long file_id, unsigned long newlen); 00208 00209 00210 00211 #ifdef __cplusplus 00212 } 00213 #endif // __cplusplus 00214 00215 //***************************************************************************** 00216 // 00217 // Close the Doxygen group. 00218 //! @} 00219 // 00220 //***************************************************************************** 00221 00222 #endif // __NVRAM_H__ 00223 00224 00225 00226 00227
Generated on Wed Jul 13 2022 18:31:31 by
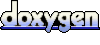