Driver for CC3000 Wi-Fi module
Dependencies: NVIC_set_all_priorities
Dependents: CC3000_Simple_Socket Wi-Go_IOT_Demo
netapp.h
00001 /***************************************************************************** 00002 * 00003 * netapp.h - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 #ifndef __NETAPP_H__ 00036 #define __NETAPP_H__ 00037 00038 #include "nvmem.h" 00039 00040 //***************************************************************************** 00041 // 00042 //! \addtogroup netapp 00043 //! @{ 00044 // 00045 //***************************************************************************** 00046 00047 /** CC3000 Host driver - netapp 00048 * 00049 */ 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 #define MIN_TIMER_VAL_SECONDS 20 00056 #define MIN_TIMER_SET(t) if ((0 != t) && (t < MIN_TIMER_VAL_SECONDS)) \ 00057 { \ 00058 t = MIN_TIMER_VAL_SECONDS; \ 00059 } 00060 00061 00062 #define NETAPP_DHCP_PARAMS_LEN (20) 00063 #define NETAPP_SET_TIMER_PARAMS_LEN (20) 00064 #define NETAPP_SET_DEBUG_LEVEL_PARAMS_LEN (4) 00065 #define NETAPP_PING_SEND_PARAMS_LEN (16) 00066 00067 00068 typedef struct _netapp_dhcp_ret_args_t 00069 { 00070 unsigned char aucIP[4]; 00071 unsigned char aucSubnetMask[4]; 00072 unsigned char aucDefaultGateway[4]; 00073 unsigned char aucDHCPServer[4]; 00074 unsigned char aucDNSServer[4]; 00075 }tNetappDhcpParams; 00076 00077 typedef struct _netapp_ipconfig_ret_args_t 00078 { 00079 unsigned char aucIP[4]; 00080 unsigned char aucSubnetMask[4]; 00081 unsigned char aucDefaultGateway[4]; 00082 unsigned char aucDHCPServer[4]; 00083 unsigned char aucDNSServer[4]; 00084 unsigned char uaMacAddr[6]; 00085 unsigned char uaSSID[32]; 00086 }tNetappIpconfigRetArgs; 00087 00088 00089 /*Ping send report parameters*/ 00090 typedef struct _netapp_pingreport_args 00091 { 00092 unsigned long packets_sent; 00093 unsigned long packets_received; 00094 unsigned long min_round_time; 00095 unsigned long max_round_time; 00096 unsigned long avg_round_time; 00097 } netapp_pingreport_args_t; 00098 00099 00100 /** 00101 * Configure device MAC address and store it in NVMEM. 00102 * The value of the MAC address configured through the API will be\n 00103 * stored in CC3000 non volatile memory, thus preserved over resets.\n 00104 * @param mac device mac address, 6 bytes. Saved: yes 00105 * @return return on success 0, otherwise error. 00106 */ 00107 extern long netapp_config_mac_adrress( unsigned char *mac ); 00108 00109 /** 00110 * Configure the network interface, static or dynamic (DHCP). 00111 * In order to activate DHCP mode, aucIP, aucSubnetMask, aucDefaultGateway must be 0.\n 00112 * The default mode of CC3000 is DHCP mode. The configuration is saved in non volatile memory\n 00113 * and thus preserved over resets.\n 00114 * @param aucIP device mac address, 6 bytes. Saved: yes 00115 * @param aucSubnetMask device mac address, 6 bytes. Saved: yes 00116 * @param aucDefaultGateway device mac address, 6 bytes. Saved: yes 00117 * @param aucDNSServer device mac address, 6 bytes. Saved: yes 00118 * @return 0 on success, otherwise error. 00119 * @note If the mode is altered, a reset of CC3000 device is required to apply the changes.\n 00120 * Also note that an asynchronous event of type 'DHCP_EVENT' is generated only when\n 00121 * a connection to the AP was established. This event is generated when an IP address\n 00122 * is allocated either by the DHCP server or by static allocation.\n 00123 */ 00124 extern long netapp_dhcp(unsigned long *aucIP, unsigned long *aucSubnetMask,unsigned long *aucDefaultGateway, unsigned long *aucDNSServer); 00125 00126 /** 00127 * Set new timeout values for DHCP lease timeout, ARP refresh timeout, keepalive event timeout and socket inactivity timeout 00128 * @param aucDHCP DHCP lease time request, also impact\n 00129 * the DHCP renew timeout.\n 00130 * Range: [0-0xffffffff] seconds,\n 00131 * 0 or 0xffffffff = infinite lease timeout.\n 00132 * Resolution: 10 seconds.\n 00133 * Influence: only after reconnecting to the AP. \n 00134 * Minimal bound value: MIN_TIMER_VAL_SECONDS - 20 seconds.\n 00135 * The parameter is saved into the CC3000 NVMEM.\n 00136 * The default value on CC3000 is 14400 seconds.\n 00137 * 00138 * @param aucARP ARP refresh timeout, if ARP entry is not updated by\n 00139 * incoming packet, the ARP entry will be deleted by\n 00140 * the end of the timeout. \n 00141 * Range: [0-0xffffffff] seconds, 0 = infinite ARP timeout\n 00142 * Resolution: 10 seconds.\n 00143 * Influence: at runtime.\n 00144 * Minimal bound value: MIN_TIMER_VAL_SECONDS - 20 seconds\n 00145 * The parameter is saved into the CC3000 NVMEM.\n 00146 * The default value on CC3000 is 3600 seconds.\n 00147 * 00148 * @param aucKeepalive Keepalive event sent by the end of keepalive timeout\n 00149 * Range: [0-0xffffffff] seconds, 0 == infinite timeout\n 00150 * Resolution: 10 seconds.\n 00151 * Influence: at runtime.\n 00152 * Minimal bound value: MIN_TIMER_VAL_SECONDS - 20 sec\n 00153 * The parameter is saved into the CC3000 NVMEM. \n 00154 * The default value on CC3000 is 10 seconds.\n 00155 * 00156 * @param aucInactivity Socket inactivity timeout, socket timeout is\n 00157 * refreshed by incoming or outgoing packet, by the\n 00158 * end of the socket timeout the socket will be closed\n 00159 * Range: [0-0xffffffff] sec, 0 == infinite timeout.\n 00160 * Resolution: 10 seconds.\n 00161 * Influence: at runtime.\n 00162 * Minimal bound value: MIN_TIMER_VAL_SECONDS - 20 sec\n 00163 * The parameter is saved into the CC3000 NVMEM.\n 00164 * The default value on CC3000 is 60 seconds.\n 00165 * 00166 * @return 0 on success,otherwise error. 00167 * 00168 * @note A parameter set to a non zero value less than 20s automatically changes to 20s. 00169 */ 00170 #ifndef CC3000_TINY_DRIVER 00171 extern long netapp_timeout_values(unsigned long *aucDHCP, unsigned long *aucARP,unsigned long *aucKeepalive, unsigned long *aucInactivity); 00172 #endif 00173 00174 /** 00175 * send ICMP ECHO_REQUEST to network hosts 00176 * @param ip destination IP address 00177 * @param pingAttempts number of echo requests to send 00178 * @param pingSize send buffer size which may be up to 1400 bytes 00179 * @param pingTimeout Time to wait for a response,in milliseconds. 00180 * @return 0 on success, otherwise error. 00181 * 00182 * @note A succesful operation will generate an asynchronous ping report event.\n 00183 * The report structure is defined by structure netapp_pingreport_args_t.\n 00184 * @warning Calling this function while a Ping Request is in progress will kill the ping request in progress. 00185 */ 00186 #ifndef CC3000_TINY_DRIVER 00187 extern long netapp_ping_send(unsigned long *ip, unsigned long ulPingAttempts, unsigned long ulPingSize, unsigned long ulPingTimeout); 00188 #endif 00189 00190 /** 00191 * Stop any ping request. 00192 * @param none 00193 * @return 0 on success 00194 * -1 on error 00195 */ 00196 #ifndef CC3000_TINY_DRIVER 00197 extern long netapp_ping_stop(void); 00198 #endif 00199 00200 /** 00201 * Ping status request. 00202 * This API triggers the CC3000 to send asynchronous events: HCI_EVNT_WLAN_ASYNC_PING_REPORT.\n 00203 * This event will create the report structure in netapp_pingreport_args_t.\n 00204 * This structure is filled with ping results until the API is triggered.\n 00205 * netapp_pingreport_args_t: packets_sent - echo sent\n 00206 * packets_received - echo reply\n 00207 * min_round_time - minimum round time\n 00208 * max_round_time - max round time\n 00209 * avg_round_time - average round time\n 00210 * 00211 * @param none 00212 * @return none 00213 * @note When a ping operation is not active, the returned structure fields are 0. 00214 */ 00215 #ifndef CC3000_TINY_DRIVER 00216 extern void netapp_ping_report(void); 00217 #endif 00218 00219 00220 /** 00221 * Get the CC3000 Network interface information. 00222 * This information is only available after establishing a WLAN connection.\n 00223 * Undefined values are returned when this function is called before association.\n 00224 * @param[out] ipconfig pointer to a tNetappIpconfigRetArgs structure for storing the network interface configuration.\n 00225 * tNetappIpconfigRetArgs: aucIP - ip address,\n 00226 * aucSubnetMask - mask 00227 * aucDefaultGateway - default gateway address\n 00228 * aucDHCPServer - dhcp server address\n 00229 * aucDNSServer - dns server address\n 00230 * uaMacAddr - mac address\n 00231 * uaSSID - connected AP ssid\n 00232 * @return none 00233 * @note This function is useful for figuring out the IP Configuration of\n 00234 * the device when DHCP is used and for figuring out the SSID of\n 00235 * the Wireless network the device is associated with.\n 00236 */ 00237 extern void netapp_ipconfig( tNetappIpconfigRetArgs * ipconfig ); 00238 00239 00240 /** 00241 * Flush ARP table 00242 * @param none 00243 * @return none 00244 */ 00245 #ifndef CC3000_TINY_DRIVER 00246 extern long netapp_arp_flush(void); 00247 #endif 00248 00249 00250 #ifdef __cplusplus 00251 } 00252 #endif // __cplusplus 00253 00254 //***************************************************************************** 00255 // 00256 // Close the Doxygen group. 00257 //! @} 00258 // 00259 //***************************************************************************** 00260 00261 #endif // __NETAPP_H__ 00262 00263 00264 00265 00266 00267
Generated on Wed Jul 13 2022 18:31:31 by
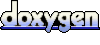