Driver for CC3000 Wi-Fi module
Dependencies: NVIC_set_all_priorities
Dependents: CC3000_Simple_Socket Wi-Go_IOT_Demo
evnt_handler.h
00001 /***************************************************************************** 00002 * 00003 * evnt_handler.h - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 #ifndef __EVENT_HANDLER_H__ 00036 #define __EVENT_HANDLER_H__ 00037 00038 #include "netapp.h" 00039 00040 //***************************************************************************** 00041 // 00042 //! \addtogroup evnt_handler 00043 //! @{ 00044 // 00045 //***************************************************************************** 00046 00047 /** CC3000 Host driver - event handler 00048 * 00049 */ 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 //***************************************************************************** 00056 // COMMON DEFINES 00057 //***************************************************************************** 00058 00059 #define FLOW_CONTROL_EVENT_HANDLE_OFFSET (0) 00060 #define FLOW_CONTROL_EVENT_BLOCK_MODE_OFFSET (1) 00061 #define FLOW_CONTROL_EVENT_FREE_BUFFS_OFFSET (2) 00062 #define FLOW_CONTROL_EVENT_SIZE (4) 00063 00064 #define BSD_RSP_PARAMS_SOCKET_OFFSET (0) 00065 #define BSD_RSP_PARAMS_STATUS_OFFSET (4) 00066 00067 #define GET_HOST_BY_NAME_RETVAL_OFFSET (0) 00068 #define GET_HOST_BY_NAME_ADDR_OFFSET (4) 00069 00070 #define ACCEPT_SD_OFFSET (0) 00071 #define ACCEPT_RETURN_STATUS_OFFSET (4) 00072 #define ACCEPT_ADDRESS__OFFSET (8) 00073 00074 #define SL_RECEIVE_SD_OFFSET (0) 00075 #define SL_RECEIVE_NUM_BYTES_OFFSET (4) 00076 #define SL_RECEIVE__FLAGS__OFFSET (8) 00077 00078 00079 #define SELECT_STATUS_OFFSET (0) 00080 #define SELECT_READFD_OFFSET (4) 00081 #define SELECT_WRITEFD_OFFSET (8) 00082 #define SELECT_EXFD_OFFSET (12) 00083 00084 00085 #define NETAPP_IPCONFIG_IP_OFFSET (0) 00086 #define NETAPP_IPCONFIG_SUBNET_OFFSET (4) 00087 #define NETAPP_IPCONFIG_GW_OFFSET (8) 00088 #define NETAPP_IPCONFIG_DHCP_OFFSET (12) 00089 #define NETAPP_IPCONFIG_DNS_OFFSET (16) 00090 #define NETAPP_IPCONFIG_MAC_OFFSET (20) 00091 #define NETAPP_IPCONFIG_SSID_OFFSET (26) 00092 00093 #define NETAPP_IPCONFIG_IP_LENGTH (4) 00094 #define NETAPP_IPCONFIG_MAC_LENGTH (6) 00095 #define NETAPP_IPCONFIG_SSID_LENGTH (32) 00096 00097 00098 #define NETAPP_PING_PACKETS_SENT_OFFSET (0) 00099 #define NETAPP_PING_PACKETS_RCVD_OFFSET (4) 00100 #define NETAPP_PING_MIN_RTT_OFFSET (8) 00101 #define NETAPP_PING_MAX_RTT_OFFSET (12) 00102 #define NETAPP_PING_AVG_RTT_OFFSET (16) 00103 00104 #define GET_SCAN_RESULTS_TABlE_COUNT_OFFSET (0) 00105 #define GET_SCAN_RESULTS_SCANRESULT_STATUS_OFFSET (4) 00106 #define GET_SCAN_RESULTS_ISVALID_TO_SSIDLEN_OFFSET (8) 00107 #define GET_SCAN_RESULTS_FRAME_TIME_OFFSET (10) 00108 #define GET_SCAN_RESULTS_SSID_MAC_LENGTH (38) 00109 00110 //***************************************************************************** 00111 // 00112 // Prototypes for the APIs. 00113 // 00114 //***************************************************************************** 00115 00116 /** Handle unsolicited event from type patch request. 00117 * @param event_hdr event header 00118 * @return none 00119 */ 00120 void hci_unsol_handle_patch_request(char *event_hdr); 00121 00122 00123 /** 00124 * Parse the incoming event packets and issue corresponding event handler from global array of handlers pointers. 00125 * @param pRetParams incoming data buffer 00126 * @param from from information (in case of data received) 00127 * @param fromlen from information length (in case of data received) 00128 * @return none 00129 */ 00130 extern unsigned char *hci_event_handler(void *pRetParams, unsigned char *from, unsigned char *fromlen); 00131 00132 /** 00133 * Handle unsolicited events. 00134 * @param event_hdr event header 00135 * @return 1 if event supported and handled 00136 * @return 0 if event is not supported 00137 */ 00138 extern long hci_unsol_event_handler(char *event_hdr); 00139 00140 /** 00141 * Parse the incoming unsolicited event packets and start corresponding event handler. 00142 * @param None 00143 * @return ESUCCESS if successful, EFAIL if an error occurred 00144 */ 00145 extern long hci_unsolicited_event_handler(void); 00146 00147 00148 #define M_BSD_RESP_PARAMS_OFFSET(hci_event_hdr)((char *)(hci_event_hdr) + HCI_EVENT_HEADER_SIZE) 00149 00150 #define SOCKET_STATUS_ACTIVE 0 00151 #define SOCKET_STATUS_INACTIVE 1 00152 /* Init socket_active_status = 'all ones': init all sockets with SOCKET_STATUS_INACTIVE. 00153 Will be changed by 'set_socket_active_status' upon 'connect' and 'accept' calls */ 00154 #define SOCKET_STATUS_INIT_VAL 0xFFFF 00155 #define M_IS_VALID_SD(sd) ((0 <= (sd)) && ((sd) <= 7)) 00156 #define M_IS_VALID_STATUS(status) (((status) == SOCKET_STATUS_ACTIVE)||((status) == SOCKET_STATUS_INACTIVE)) 00157 00158 extern unsigned long socket_active_status; 00159 00160 /** 00161 * Check if the socket ID and status are valid and set the global socket status accordingly. 00162 * @param Sd 00163 * @param Status 00164 * @return none 00165 */ 00166 extern void set_socket_active_status(long Sd, long Status); 00167 00168 /** 00169 * Keep track on the number of packets transmitted and update the number of free buffer in the SL device. 00170 * Called when unsolicited event = HCI_EVNT_DATA_UNSOL_FREE_BUFF has received.\n 00171 * @param pEvent pointer to the string contains parameters for IPERF 00172 * @return ESUCCESS if successful, EFAIL if an error occurred 00173 */ 00174 long hci_event_unsol_flowcontrol_handler(char *pEvent); 00175 00176 /** 00177 * Get the socket status. 00178 * @param Sd Socket IS 00179 * @return Current status of the socket. 00180 */ 00181 extern long get_socket_active_status(long Sd); 00182 00183 /** 00184 * Update the socket status. 00185 * @param resp_params Socket IS 00186 * @return Current status of the socket. 00187 */ 00188 void update_socket_active_status(char *resp_params); 00189 00190 /** 00191 * Wait for event, pass it to the hci_event_handler and update the event opcode in a global variable. 00192 * @param usOpcode command operation code 00193 * @param pRetParams command return parameters 00194 * @return none 00195 */ 00196 void SimpleLinkWaitEvent(unsigned short usOpcode, void *pRetParams); 00197 00198 /** 00199 * Wait for data, pass it to the hci_event_handler and set the data available flag. 00200 * @param pBuf data buffer 00201 * @param from from information 00202 * @param fromlen from information length 00203 * @return none 00204 */ 00205 void SimpleLinkWaitData(unsigned char *pBuf, unsigned char *from, unsigned char *fromlen); 00206 00207 00208 typedef struct _bsd_accept_return_t 00209 { 00210 long iSocketDescriptor; 00211 long iStatus; 00212 sockaddr tSocketAddress; 00213 00214 } tBsdReturnParams; 00215 00216 00217 typedef struct _bsd_read_return_t 00218 { 00219 long iSocketDescriptor; 00220 long iNumberOfBytes; 00221 unsigned long uiFlags; 00222 } tBsdReadReturnParams; 00223 00224 #define BSD_RECV_FROM_FROMLEN_OFFSET (4) 00225 #define BSD_RECV_FROM_FROM_OFFSET (16) 00226 00227 00228 typedef struct _bsd_select_return_t 00229 { 00230 long iStatus; 00231 unsigned long uiRdfd; 00232 unsigned long uiWrfd; 00233 unsigned long uiExfd; 00234 } tBsdSelectRecvParams; 00235 00236 00237 typedef struct _bsd_getsockopt_return_t 00238 { 00239 unsigned char ucOptValue[4]; 00240 char iStatus; 00241 } tBsdGetSockOptReturnParams; 00242 00243 typedef struct _bsd_gethostbyname_return_t 00244 { 00245 long retVal; 00246 long outputAddress; 00247 } tBsdGethostbynameParams; 00248 00249 #ifdef __cplusplus 00250 } 00251 #endif // __cplusplus 00252 00253 //***************************************************************************** 00254 // 00255 // Close the Doxygen group. 00256 //! @} 00257 // 00258 //***************************************************************************** 00259 00260 #endif // __EVENT_HANDLER_H__ 00261 00262 00263 00264 00265 00266 00267 00268
Generated on Wed Jul 13 2022 18:31:31 by
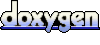