Date: March 20, 2011 This library is created from "LPC17xx CMSIS-Compliant Standard Peripheral Firmware Driver Library (GNU, Keil, IAR) (Jan 28, 2011)", available from NXP's website, under "All microcontrollers support documents" [[http://ics.nxp.com/support/documents/microcontrollers/?type=software]] You will need to follow [[/projects/libraries/svn/mbed/trunk/LPC1768/LPC17xx.h]] while using this library Examples provided here [[/users/frank26080115/programs/LPC1700CMSIS_Examples/]] The beautiful thing is that NXP does not place copyright protection on any of the files in here Only a few modifications are made to make it compile with the mbed online compiler, I fixed some warnings as well. This is untested as of March 20, 2011 Forum post about this library: [[/forum/mbed/topic/2030/]]
lpc17xx_timer.c
00001 /***********************************************************************//** 00002 * @file lpc17xx_timer.c 00003 * @brief Contains all functions support for Timer firmware library on LPC17xx 00004 * @version 3.0 00005 * @date 18. June. 2010 00006 * @author NXP MCU SW Application Team 00007 ************************************************************************** 00008 * Software that is described herein is for illustrative purposes only 00009 * which provides customers with programming information regarding the 00010 * products. This software is supplied "AS IS" without any warranties. 00011 * NXP Semiconductors assumes no responsibility or liability for the 00012 * use of the software, conveys no license or title under any patent, 00013 * copyright, or mask work right to the product. NXP Semiconductors 00014 * reserves the right to make changes in the software without 00015 * notification. NXP Semiconductors also make no representation or 00016 * warranty that such application will be suitable for the specified 00017 * use without further testing or modification. 00018 **********************************************************************/ 00019 00020 /* Peripheral group ----------------------------------------------------------- */ 00021 /** @addtogroup TIM 00022 * @{ 00023 */ 00024 00025 /* Includes ------------------------------------------------------------------- */ 00026 #include "lpc17xx_timer.h" 00027 #include "lpc17xx_clkpwr.h" 00028 #include "lpc17xx_pinsel.h" 00029 00030 /* If this source file built with example, the LPC17xx FW library configuration 00031 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00032 * otherwise the default FW library configuration file must be included instead 00033 */ 00034 #ifdef __BUILD_WITH_EXAMPLE__ 00035 #include "lpc17xx_libcfg.h" 00036 #else 00037 #include "lpc17xx_libcfg_default.h" 00038 #endif /* __BUILD_WITH_EXAMPLE__ */ 00039 00040 #ifdef _TIM 00041 00042 /* Private Functions ---------------------------------------------------------- */ 00043 00044 static uint32_t getPClock (uint32_t timernum); 00045 static uint32_t converUSecToVal (uint32_t timernum, uint32_t usec); 00046 static uint32_t converPtrToTimeNum (LPC_TIM_TypeDef *TIMx); 00047 00048 00049 /*********************************************************************//** 00050 * @brief Get peripheral clock of each timer controller 00051 * @param[in] timernum Timer number 00052 * @return Peripheral clock of timer 00053 **********************************************************************/ 00054 static uint32_t getPClock (uint32_t timernum) 00055 { 00056 uint32_t clkdlycnt = 0; 00057 switch (timernum) 00058 { 00059 case 0: 00060 clkdlycnt = CLKPWR_GetPCLK (CLKPWR_PCLKSEL_TIMER0); 00061 break; 00062 00063 case 1: 00064 clkdlycnt = CLKPWR_GetPCLK (CLKPWR_PCLKSEL_TIMER1); 00065 break; 00066 00067 case 2: 00068 clkdlycnt = CLKPWR_GetPCLK (CLKPWR_PCLKSEL_TIMER2); 00069 break; 00070 00071 case 3: 00072 clkdlycnt = CLKPWR_GetPCLK (CLKPWR_PCLKSEL_TIMER3); 00073 break; 00074 } 00075 return clkdlycnt; 00076 } 00077 00078 00079 /*********************************************************************//** 00080 * @brief Convert a time to a timer count value 00081 * @param[in] timernum Timer number 00082 * @param[in] usec Time in microseconds 00083 * @return The number of required clock ticks to give the time delay 00084 **********************************************************************/ 00085 uint32_t converUSecToVal (uint32_t timernum, uint32_t usec) 00086 { 00087 uint64_t clkdlycnt; 00088 00089 // Get Pclock of timer 00090 clkdlycnt = (uint64_t) getPClock(timernum); 00091 00092 clkdlycnt = (clkdlycnt * usec) / 1000000; 00093 return (uint32_t) clkdlycnt; 00094 } 00095 00096 00097 /*********************************************************************//** 00098 * @brief Convert a timer register pointer to a timer number 00099 * @param[in] TIMx Pointer to LPC_TIM_TypeDef, should be: 00100 * - LPC_TIM0: TIMER0 peripheral 00101 * - LPC_TIM1: TIMER1 peripheral 00102 * - LPC_TIM2: TIMER2 peripheral 00103 * - LPC_TIM3: TIMER3 peripheral 00104 * @return The timer number (0 to 3) or -1 if register pointer is bad 00105 **********************************************************************/ 00106 uint32_t converPtrToTimeNum (LPC_TIM_TypeDef *TIMx) 00107 { 00108 uint32_t tnum = 0xFFFFFFFF; 00109 00110 if (TIMx == LPC_TIM0) 00111 { 00112 tnum = 0; 00113 } 00114 else if (TIMx == LPC_TIM1) 00115 { 00116 tnum = 1; 00117 } 00118 else if (TIMx == LPC_TIM2) 00119 { 00120 tnum = 2; 00121 } 00122 else if (TIMx == LPC_TIM3) 00123 { 00124 tnum = 3; 00125 } 00126 00127 return tnum; 00128 } 00129 00130 /* End of Private Functions ---------------------------------------------------- */ 00131 00132 00133 /* Public Functions ----------------------------------------------------------- */ 00134 /** @addtogroup TIM_Public_Functions 00135 * @{ 00136 */ 00137 00138 /*********************************************************************//** 00139 * @brief Get Interrupt Status 00140 * @param[in] TIMx Timer selection, should be: 00141 * - LPC_TIM0: TIMER0 peripheral 00142 * - LPC_TIM1: TIMER1 peripheral 00143 * - LPC_TIM2: TIMER2 peripheral 00144 * - LPC_TIM3: TIMER3 peripheral 00145 * @param[in] IntFlag: interrupt type, should be: 00146 * - TIM_MR0_INT: Interrupt for Match channel 0 00147 * - TIM_MR1_INT: Interrupt for Match channel 1 00148 * - TIM_MR2_INT: Interrupt for Match channel 2 00149 * - TIM_MR3_INT: Interrupt for Match channel 3 00150 * - TIM_CR0_INT: Interrupt for Capture channel 0 00151 * - TIM_CR1_INT: Interrupt for Capture channel 1 00152 * @return FlagStatus 00153 * - SET : interrupt 00154 * - RESET : no interrupt 00155 **********************************************************************/ 00156 FlagStatus TIM_GetIntStatus(LPC_TIM_TypeDef *TIMx, TIM_INT_TYPE IntFlag) 00157 { 00158 uint8_t temp; 00159 CHECK_PARAM(PARAM_TIMx(TIMx)); 00160 CHECK_PARAM(PARAM_TIM_INT_TYPE(IntFlag)); 00161 temp = (TIMx->IR)& TIM_IR_CLR(IntFlag); 00162 if (temp) 00163 return SET; 00164 00165 return RESET; 00166 00167 } 00168 /*********************************************************************//** 00169 * @brief Get Capture Interrupt Status 00170 * @param[in] TIMx Timer selection, should be: 00171 * - LPC_TIM0: TIMER0 peripheral 00172 * - LPC_TIM1: TIMER1 peripheral 00173 * - LPC_TIM2: TIMER2 peripheral 00174 * - LPC_TIM3: TIMER3 peripheral 00175 * @param[in] IntFlag: interrupt type, should be: 00176 * - TIM_MR0_INT: Interrupt for Match channel 0 00177 * - TIM_MR1_INT: Interrupt for Match channel 1 00178 * - TIM_MR2_INT: Interrupt for Match channel 2 00179 * - TIM_MR3_INT: Interrupt for Match channel 3 00180 * - TIM_CR0_INT: Interrupt for Capture channel 0 00181 * - TIM_CR1_INT: Interrupt for Capture channel 1 00182 * @return FlagStatus 00183 * - SET : interrupt 00184 * - RESET : no interrupt 00185 **********************************************************************/ 00186 FlagStatus TIM_GetIntCaptureStatus(LPC_TIM_TypeDef *TIMx, TIM_INT_TYPE IntFlag) 00187 { 00188 uint8_t temp; 00189 CHECK_PARAM(PARAM_TIMx(TIMx)); 00190 CHECK_PARAM(PARAM_TIM_INT_TYPE(IntFlag)); 00191 temp = (TIMx->IR) & (1<<(4+IntFlag)); 00192 if(temp) 00193 return SET; 00194 return RESET; 00195 } 00196 /*********************************************************************//** 00197 * @brief Clear Interrupt pending 00198 * @param[in] TIMx Timer selection, should be: 00199 * - LPC_TIM0: TIMER0 peripheral 00200 * - LPC_TIM1: TIMER1 peripheral 00201 * - LPC_TIM2: TIMER2 peripheral 00202 * - LPC_TIM3: TIMER3 peripheral 00203 * @param[in] IntFlag: interrupt type, should be: 00204 * - TIM_MR0_INT: Interrupt for Match channel 0 00205 * - TIM_MR1_INT: Interrupt for Match channel 1 00206 * - TIM_MR2_INT: Interrupt for Match channel 2 00207 * - TIM_MR3_INT: Interrupt for Match channel 3 00208 * - TIM_CR0_INT: Interrupt for Capture channel 0 00209 * - TIM_CR1_INT: Interrupt for Capture channel 1 00210 * @return None 00211 **********************************************************************/ 00212 void TIM_ClearIntPending(LPC_TIM_TypeDef *TIMx, TIM_INT_TYPE IntFlag) 00213 { 00214 CHECK_PARAM(PARAM_TIMx(TIMx)); 00215 CHECK_PARAM(PARAM_TIM_INT_TYPE(IntFlag)); 00216 TIMx->IR |= TIM_IR_CLR(IntFlag); 00217 } 00218 00219 /*********************************************************************//** 00220 * @brief Clear Capture Interrupt pending 00221 * @param[in] TIMx Timer selection, should be 00222 * - LPC_TIM0: TIMER0 peripheral 00223 * - LPC_TIM1: TIMER1 peripheral 00224 * - LPC_TIM2: TIMER2 peripheral 00225 * - LPC_TIM3: TIMER3 peripheral 00226 * @param[in] IntFlag interrupt type, should be: 00227 * - TIM_MR0_INT: Interrupt for Match channel 0 00228 * - TIM_MR1_INT: Interrupt for Match channel 1 00229 * - TIM_MR2_INT: Interrupt for Match channel 2 00230 * - TIM_MR3_INT: Interrupt for Match channel 3 00231 * - TIM_CR0_INT: Interrupt for Capture channel 0 00232 * - TIM_CR1_INT: Interrupt for Capture channel 1 00233 * @return None 00234 **********************************************************************/ 00235 void TIM_ClearIntCapturePending(LPC_TIM_TypeDef *TIMx, TIM_INT_TYPE IntFlag) 00236 { 00237 CHECK_PARAM(PARAM_TIMx(TIMx)); 00238 CHECK_PARAM(PARAM_TIM_INT_TYPE(IntFlag)); 00239 TIMx->IR |= (1<<(4+IntFlag)); 00240 } 00241 00242 /*********************************************************************//** 00243 * @brief Configuration for Timer at initial time 00244 * @param[in] TimerCounterMode timer counter mode, should be: 00245 * - TIM_TIMER_MODE: Timer mode 00246 * - TIM_COUNTER_RISING_MODE: Counter rising mode 00247 * - TIM_COUNTER_FALLING_MODE: Counter falling mode 00248 * - TIM_COUNTER_ANY_MODE:Counter on both edges 00249 * @param[in] TIM_ConfigStruct pointer to TIM_TIMERCFG_Type or 00250 * TIM_COUNTERCFG_Type 00251 * @return None 00252 **********************************************************************/ 00253 void TIM_ConfigStructInit(TIM_MODE_OPT TimerCounterMode, void *TIM_ConfigStruct) 00254 { 00255 if (TimerCounterMode == TIM_TIMER_MODE ) 00256 { 00257 TIM_TIMERCFG_Type * pTimeCfg = (TIM_TIMERCFG_Type *)TIM_ConfigStruct; 00258 pTimeCfg->PrescaleOption = TIM_PRESCALE_USVAL ; 00259 pTimeCfg->PrescaleValue = 1; 00260 } 00261 else 00262 { 00263 TIM_COUNTERCFG_Type * pCounterCfg = (TIM_COUNTERCFG_Type *)TIM_ConfigStruct; 00264 pCounterCfg->CountInputSelect = TIM_COUNTER_INCAP0 ; 00265 } 00266 } 00267 00268 /*********************************************************************//** 00269 * @brief Initial Timer/Counter device 00270 * Set Clock frequency for Timer 00271 * Set initial configuration for Timer 00272 * @param[in] TIMx Timer selection, should be: 00273 * - LPC_TIM0: TIMER0 peripheral 00274 * - LPC_TIM1: TIMER1 peripheral 00275 * - LPC_TIM2: TIMER2 peripheral 00276 * - LPC_TIM3: TIMER3 peripheral 00277 * @param[in] TimerCounterMode Timer counter mode, should be: 00278 * - TIM_TIMER_MODE: Timer mode 00279 * - TIM_COUNTER_RISING_MODE: Counter rising mode 00280 * - TIM_COUNTER_FALLING_MODE: Counter falling mode 00281 * - TIM_COUNTER_ANY_MODE:Counter on both edges 00282 * @param[in] TIM_ConfigStruct pointer to TIM_TIMERCFG_Type 00283 * that contains the configuration information for the 00284 * specified Timer peripheral. 00285 * @return None 00286 **********************************************************************/ 00287 void TIM_Init(LPC_TIM_TypeDef *TIMx, TIM_MODE_OPT TimerCounterMode, void *TIM_ConfigStruct) 00288 { 00289 TIM_TIMERCFG_Type *pTimeCfg; 00290 TIM_COUNTERCFG_Type *pCounterCfg; 00291 00292 CHECK_PARAM(PARAM_TIMx(TIMx)); 00293 CHECK_PARAM(PARAM_TIM_MODE_OPT(TimerCounterMode)); 00294 00295 //set power 00296 if (TIMx== LPC_TIM0) 00297 { 00298 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM0, ENABLE); 00299 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_TIMER0, CLKPWR_PCLKSEL_CCLK_DIV_4); 00300 } 00301 else if (TIMx== LPC_TIM1) 00302 { 00303 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM1, ENABLE); 00304 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_TIMER1, CLKPWR_PCLKSEL_CCLK_DIV_4); 00305 00306 } 00307 00308 else if (TIMx== LPC_TIM2) 00309 { 00310 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM2, ENABLE); 00311 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_TIMER2, CLKPWR_PCLKSEL_CCLK_DIV_4); 00312 } 00313 else if (TIMx== LPC_TIM3) 00314 { 00315 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM3, ENABLE); 00316 CLKPWR_SetPCLKDiv (CLKPWR_PCLKSEL_TIMER3, CLKPWR_PCLKSEL_CCLK_DIV_4); 00317 00318 } 00319 00320 TIMx->CCR &= ~TIM_CTCR_MODE_MASK; 00321 TIMx->CCR |= TIM_TIMER_MODE ; 00322 00323 TIMx->TC =0; 00324 TIMx->PC =0; 00325 TIMx->PR =0; 00326 TIMx->TCR |= (1<<1); //Reset Counter 00327 TIMx->TCR &= ~(1<<1); //release reset 00328 if (TimerCounterMode == TIM_TIMER_MODE ) 00329 { 00330 pTimeCfg = (TIM_TIMERCFG_Type *)TIM_ConfigStruct; 00331 if (pTimeCfg->PrescaleOption == TIM_PRESCALE_TICKVAL ) 00332 { 00333 TIMx->PR = pTimeCfg->PrescaleValue -1 ; 00334 } 00335 else 00336 { 00337 TIMx->PR = converUSecToVal (converPtrToTimeNum(TIMx),pTimeCfg->PrescaleValue)-1; 00338 } 00339 } 00340 else 00341 { 00342 00343 pCounterCfg = (TIM_COUNTERCFG_Type *)TIM_ConfigStruct; 00344 TIMx->CCR &= ~TIM_CTCR_INPUT_MASK; 00345 if (pCounterCfg->CountInputSelect == TIM_COUNTER_INCAP1 ) 00346 TIMx->CCR |= _BIT(2); 00347 } 00348 00349 // Clear interrupt pending 00350 TIMx->IR = 0xFFFFFFFF; 00351 00352 } 00353 00354 /*********************************************************************//** 00355 * @brief Close Timer/Counter device 00356 * @param[in] TIMx Pointer to timer device, should be: 00357 * - LPC_TIM0: TIMER0 peripheral 00358 * - LPC_TIM1: TIMER1 peripheral 00359 * - LPC_TIM2: TIMER2 peripheral 00360 * - LPC_TIM3: TIMER3 peripheral 00361 * @return None 00362 **********************************************************************/ 00363 void TIM_DeInit (LPC_TIM_TypeDef *TIMx) 00364 { 00365 CHECK_PARAM(PARAM_TIMx(TIMx)); 00366 // Disable timer/counter 00367 TIMx->TCR = 0x00; 00368 00369 // Disable power 00370 if (TIMx== LPC_TIM0) 00371 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM0, DISABLE); 00372 00373 else if (TIMx== LPC_TIM1) 00374 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM1, DISABLE); 00375 00376 else if (TIMx== LPC_TIM2) 00377 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM2, DISABLE); 00378 00379 else if (TIMx== LPC_TIM3) 00380 CLKPWR_ConfigPPWR (CLKPWR_PCONP_PCTIM2, DISABLE); 00381 00382 } 00383 00384 /*********************************************************************//** 00385 * @brief Start/Stop Timer/Counter device 00386 * @param[in] TIMx Pointer to timer device, should be: 00387 * - LPC_TIM0: TIMER0 peripheral 00388 * - LPC_TIM1: TIMER1 peripheral 00389 * - LPC_TIM2: TIMER2 peripheral 00390 * - LPC_TIM3: TIMER3 peripheral 00391 * @param[in] NewState 00392 * - ENABLE : set timer enable 00393 * - DISABLE : disable timer 00394 * @return None 00395 **********************************************************************/ 00396 void TIM_Cmd(LPC_TIM_TypeDef *TIMx, FunctionalState NewState) 00397 { 00398 CHECK_PARAM(PARAM_TIMx(TIMx)); 00399 if (NewState == ENABLE) 00400 { 00401 TIMx->TCR |= TIM_ENABLE; 00402 } 00403 else 00404 { 00405 TIMx->TCR &= ~TIM_ENABLE; 00406 } 00407 } 00408 00409 /*********************************************************************//** 00410 * @brief Reset Timer/Counter device, 00411 * Make TC and PC are synchronously reset on the next 00412 * positive edge of PCLK 00413 * @param[in] TIMx Pointer to timer device, should be: 00414 * - LPC_TIM0: TIMER0 peripheral 00415 * - LPC_TIM1: TIMER1 peripheral 00416 * - LPC_TIM2: TIMER2 peripheral 00417 * - LPC_TIM3: TIMER3 peripheral 00418 * @return None 00419 **********************************************************************/ 00420 void TIM_ResetCounter(LPC_TIM_TypeDef *TIMx) 00421 { 00422 CHECK_PARAM(PARAM_TIMx(TIMx)); 00423 TIMx->TCR |= TIM_RESET; 00424 TIMx->TCR &= ~TIM_RESET; 00425 } 00426 00427 /*********************************************************************//** 00428 * @brief Configuration for Match register 00429 * @param[in] TIMx Pointer to timer device, should be: 00430 * - LPC_TIM0: TIMER0 peripheral 00431 * - LPC_TIM1: TIMER1 peripheral 00432 * - LPC_TIM2: TIMER2 peripheral 00433 * - LPC_TIM3: TIMER3 peripheral 00434 * @param[in] TIM_MatchConfigStruct Pointer to TIM_MATCHCFG_Type 00435 * - MatchChannel : choose channel 0 or 1 00436 * - IntOnMatch : if SET, interrupt will be generated when MRxx match 00437 * the value in TC 00438 * - StopOnMatch : if SET, TC and PC will be stopped whenM Rxx match 00439 * the value in TC 00440 * - ResetOnMatch : if SET, Reset on MR0 when MRxx match 00441 * the value in TC 00442 * -ExtMatchOutputType: Select output for external match 00443 * + 0: Do nothing for external output pin if match 00444 * + 1: Force external output pin to low if match 00445 * + 2: Force external output pin to high if match 00446 * + 3: Toggle external output pin if match 00447 * MatchValue: Set the value to be compared with TC value 00448 * @return None 00449 **********************************************************************/ 00450 void TIM_ConfigMatch(LPC_TIM_TypeDef *TIMx, TIM_MATCHCFG_Type *TIM_MatchConfigStruct) 00451 { 00452 00453 CHECK_PARAM(PARAM_TIMx(TIMx)); 00454 CHECK_PARAM(PARAM_TIM_EXTMATCH_OPT(TIM_MatchConfigStruct->ExtMatchOutputType)); 00455 00456 switch(TIM_MatchConfigStruct->MatchChannel) 00457 { 00458 case 0: 00459 TIMx->MR0 = TIM_MatchConfigStruct->MatchValue; 00460 break; 00461 case 1: 00462 TIMx->MR1 = TIM_MatchConfigStruct->MatchValue; 00463 break; 00464 case 2: 00465 TIMx->MR2 = TIM_MatchConfigStruct->MatchValue; 00466 break; 00467 case 3: 00468 TIMx->MR3 = TIM_MatchConfigStruct->MatchValue; 00469 break; 00470 default: 00471 //Error match value 00472 //Error loop 00473 while(1); 00474 } 00475 //interrupt on MRn 00476 TIMx->MCR &=~TIM_MCR_CHANNEL_MASKBIT(TIM_MatchConfigStruct->MatchChannel); 00477 00478 if (TIM_MatchConfigStruct->IntOnMatch) 00479 TIMx->MCR |= TIM_INT_ON_MATCH(TIM_MatchConfigStruct->MatchChannel); 00480 00481 //reset on MRn 00482 if (TIM_MatchConfigStruct->ResetOnMatch) 00483 TIMx->MCR |= TIM_RESET_ON_MATCH(TIM_MatchConfigStruct->MatchChannel); 00484 00485 //stop on MRn 00486 if (TIM_MatchConfigStruct->StopOnMatch) 00487 TIMx->MCR |= TIM_STOP_ON_MATCH(TIM_MatchConfigStruct->MatchChannel); 00488 00489 // match output type 00490 00491 TIMx->EMR &= ~TIM_EM_MASK(TIM_MatchConfigStruct->MatchChannel); 00492 TIMx->EMR |= TIM_EM_SET(TIM_MatchConfigStruct->MatchChannel,TIM_MatchConfigStruct->ExtMatchOutputType); 00493 } 00494 /*********************************************************************//** 00495 * @brief Update Match value 00496 * @param[in] TIMx Pointer to timer device, should be: 00497 * - LPC_TIM0: TIMER0 peripheral 00498 * - LPC_TIM1: TIMER1 peripheral 00499 * - LPC_TIM2: TIMER2 peripheral 00500 * - LPC_TIM3: TIMER3 peripheral 00501 * @param[in] MatchChannel Match channel, should be: 0..3 00502 * @param[in] MatchValue updated match value 00503 * @return None 00504 **********************************************************************/ 00505 void TIM_UpdateMatchValue(LPC_TIM_TypeDef *TIMx,uint8_t MatchChannel, uint32_t MatchValue) 00506 { 00507 CHECK_PARAM(PARAM_TIMx(TIMx)); 00508 switch(MatchChannel) 00509 { 00510 case 0: 00511 TIMx->MR0 = MatchValue; 00512 break; 00513 case 1: 00514 TIMx->MR1 = MatchValue; 00515 break; 00516 case 2: 00517 TIMx->MR2 = MatchValue; 00518 break; 00519 case 3: 00520 TIMx->MR3 = MatchValue; 00521 break; 00522 default: 00523 //Error Loop 00524 while(1); 00525 } 00526 00527 } 00528 /*********************************************************************//** 00529 * @brief Configuration for Capture register 00530 * @param[in] TIMx Pointer to timer device, should be: 00531 * - LPC_TIM0: TIMER0 peripheral 00532 * - LPC_TIM1: TIMER1 peripheral 00533 * - LPC_TIM2: TIMER2 peripheral 00534 * - LPC_TIM3: TIMER3 peripheral 00535 * - CaptureChannel: set the channel to capture data 00536 * - RisingEdge : if SET, Capture at rising edge 00537 * - FallingEdge : if SET, Capture at falling edge 00538 * - IntOnCaption : if SET, Capture generate interrupt 00539 * @param[in] TIM_CaptureConfigStruct Pointer to TIM_CAPTURECFG_Type 00540 * @return None 00541 **********************************************************************/ 00542 void TIM_ConfigCapture(LPC_TIM_TypeDef *TIMx, TIM_CAPTURECFG_Type *TIM_CaptureConfigStruct) 00543 { 00544 00545 CHECK_PARAM(PARAM_TIMx(TIMx)); 00546 TIMx->CCR &= ~TIM_CCR_CHANNEL_MASKBIT(TIM_CaptureConfigStruct->CaptureChannel); 00547 00548 if (TIM_CaptureConfigStruct->RisingEdge) 00549 TIMx->CCR |= TIM_CAP_RISING(TIM_CaptureConfigStruct->CaptureChannel); 00550 00551 if (TIM_CaptureConfigStruct->FallingEdge) 00552 TIMx->CCR |= TIM_CAP_FALLING(TIM_CaptureConfigStruct->CaptureChannel); 00553 00554 if (TIM_CaptureConfigStruct->IntOnCaption) 00555 TIMx->CCR |= TIM_INT_ON_CAP(TIM_CaptureConfigStruct->CaptureChannel); 00556 } 00557 00558 /*********************************************************************//** 00559 * @brief Read value of capture register in timer/counter device 00560 * @param[in] TIMx Pointer to timer/counter device, should be: 00561 * - LPC_TIM0: TIMER0 peripheral 00562 * - LPC_TIM1: TIMER1 peripheral 00563 * - LPC_TIM2: TIMER2 peripheral 00564 * - LPC_TIM3: TIMER3 peripheral 00565 * @param[in] CaptureChannel: capture channel number, should be: 00566 * - TIM_COUNTER_INCAP0: CAPn.0 input pin for TIMERn 00567 * - TIM_COUNTER_INCAP1: CAPn.1 input pin for TIMERn 00568 * @return Value of capture register 00569 **********************************************************************/ 00570 uint32_t TIM_GetCaptureValue(LPC_TIM_TypeDef *TIMx, TIM_COUNTER_INPUT_OPT CaptureChannel) 00571 { 00572 CHECK_PARAM(PARAM_TIMx(TIMx)); 00573 CHECK_PARAM(PARAM_TIM_COUNTER_INPUT_OPT(CaptureChannel)); 00574 00575 if(CaptureChannel==0) 00576 return TIMx->CR0; 00577 else 00578 return TIMx->CR1; 00579 } 00580 00581 /** 00582 * @} 00583 */ 00584 00585 #endif /* _TIMER */ 00586 00587 /** 00588 * @} 00589 */ 00590 00591 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:33:24 by
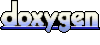