This is a fork of the mbed port of axTLS
Dependents: TLS_axTLS-Example HTTPSClientExample
os_port_old.h
00001 /* 00002 * Copyright (c) 2007, Cameron Rich 00003 * 00004 * All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions are met: 00008 * 00009 * * Redistributions of source code must retain the above copyright notice, 00010 * this list of conditions and the following disclaimer. 00011 * * Redistributions in binary form must reproduce the above copyright notice, 00012 * this list of conditions and the following disclaimer in the documentation 00013 * and/or other materials provided with the distribution. 00014 * * Neither the name of the axTLS project nor the names of its contributors 00015 * may be used to endorse or promote products derived from this software 00016 * without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00019 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00020 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00021 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR 00022 * CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00023 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, 00024 * PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 00025 * PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00026 * LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00027 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00028 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00029 */ 00030 00031 /** 00032 * @file os_port.h 00033 * 00034 * Some stuff to minimise the differences between windows and linux/unix 00035 */ 00036 00037 #ifndef HEADER_OS_PORT_H 00038 #define HEADER_OS_PORT_H 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 #include "os_int.h" 00045 #include <stdio.h> 00046 00047 #if defined(WIN32) 00048 #define STDCALL __stdcall 00049 #define EXP_FUNC __declspec(dllexport) 00050 #else 00051 #define STDCALL 00052 #define EXP_FUNC 00053 #endif 00054 00055 #if defined(_WIN32_WCE) 00056 #undef WIN32 00057 #define WIN32 00058 #endif 00059 00060 #ifdef WIN32 00061 00062 /* Windows CE stuff */ 00063 #if defined(_WIN32_WCE) 00064 #include <basetsd.h> 00065 #define abort() exit(1) 00066 #else 00067 #include <io.h> 00068 #include <process.h> 00069 #include <sys/timeb.h> 00070 #include <fcntl.h> 00071 #endif /* _WIN32_WCE */ 00072 00073 #include <winsock.h> 00074 #include <direct.h> 00075 #undef getpid 00076 #undef open 00077 #undef close 00078 #undef sleep 00079 #undef gettimeofday 00080 #undef dup2 00081 #undef unlink 00082 00083 #define SOCKET_READ(A,B,C) recv(A,B,C,0) 00084 #define SOCKET_WRITE(A,B,C) send(A,B,C,0) 00085 #define SOCKET_CLOSE(A) closesocket(A) 00086 #define srandom(A) srand(A) 00087 #define random() rand() 00088 #define getpid() _getpid() 00089 #define snprintf _snprintf 00090 #define open(A,B) _open(A,B) 00091 #define dup2(A,B) _dup2(A,B) 00092 #define unlink(A) _unlink(A) 00093 #define close(A) _close(A) 00094 #define read(A,B,C) _read(A,B,C) 00095 #define write(A,B,C) _write(A,B,C) 00096 #define sleep(A) Sleep(A*1000) 00097 #define usleep(A) Sleep(A/1000) 00098 #define strdup(A) _strdup(A) 00099 #define chroot(A) _chdir(A) 00100 #define chdir(A) _chdir(A) 00101 #define alloca(A) _alloca(A) 00102 00103 00104 #ifndef lseek 00105 #define lseek(A,B,C) _lseek(A,B,C) 00106 00107 #endif 00108 00109 /* This fix gets around a problem where a win32 application on a cygwin xterm 00110 doesn't display regular output (until a certain buffer limit) - but it works 00111 fine under a normal DOS window. This is a hack to get around the issue - 00112 see http://www.khngai.com/emacs/tty.php */ 00113 #define TTY_FLUSH() if (!_isatty(_fileno(stdout))) fflush(stdout); 00114 00115 /* 00116 * automatically build some library dependencies. 00117 */ 00118 #pragma comment(lib, "WS2_32.lib") 00119 #pragma comment(lib, "AdvAPI32.lib") 00120 00121 typedef int socklen_t; 00122 00123 EXP_FUNC void STDCALL gettimeofday(struct timeval* t,void* timezone); 00124 EXP_FUNC int STDCALL strcasecmp(const char *s1, const char *s2); 00125 EXP_FUNC int STDCALL getdomainname(char *buf, int buf_size); 00126 00127 #else /* Not Win32 */ 00128 00129 //#include <unistd.h> 00130 //#include <pwd.h> 00131 //#include <netdb.h> 00132 //#include <dirent.h> 00133 //#include <fcntl.h> 00134 #include <errno.h> 00135 //#include <sys/stat.h> 00136 #include <time.h> 00137 #include <socket.h> 00138 //#include <sys/wait.h> 00139 #include <netinet/in.h> 00140 #include <inet.h> 00141 00142 #define SOCKET_READ(A,B,C) read(A,B,C) 00143 #define SOCKET_WRITE(A,B,C) write(A,B,C) 00144 #define SOCKET_CLOSE(A) if (A >= 0) close(A) 00145 #define TTY_FLUSH() 00146 00147 #endif /* Not Win32 */ 00148 00149 /* some functions to mutate the way these work */ 00150 #define malloc(A) ax_malloc(A) 00151 #ifndef realloc 00152 #define realloc(A,B) ax_realloc(A,B) 00153 #endif 00154 #define calloc(A,B) ax_calloc(A,B) 00155 00156 EXP_FUNC void * STDCALL ax_malloc(size_t s); 00157 EXP_FUNC void * STDCALL ax_realloc(void *y, size_t s); 00158 EXP_FUNC void * STDCALL ax_calloc(size_t n, size_t s); 00159 EXP_FUNC int STDCALL ax_open(const char *pathname, int flags); 00160 00161 #ifdef CONFIG_PLATFORM_LINUX 00162 void exit_now(const char *format, ...) __attribute((noreturn)); 00163 #else 00164 void exit_now(const char *format, ...); 00165 #endif 00166 00167 /* Mutexing definitions */ 00168 #if defined(CONFIG_SSL_CTX_MUTEXING) 00169 #if defined(WIN32) 00170 #define SSL_CTX_MUTEX_TYPE HANDLE 00171 #define SSL_CTX_MUTEX_INIT(A) A=CreateMutex(0, FALSE, 0) 00172 #define SSL_CTX_MUTEX_DESTROY(A) CloseHandle(A) 00173 #define SSL_CTX_LOCK(A) WaitForSingleObject(A, INFINITE) 00174 #define SSL_CTX_UNLOCK(A) ReleaseMutex(A) 00175 #else 00176 #include <pthread.h> 00177 #define SSL_CTX_MUTEX_TYPE pthread_mutex_t 00178 #define SSL_CTX_MUTEX_INIT(A) pthread_mutex_init(&A, NULL) 00179 #define SSL_CTX_MUTEX_DESTROY(A) pthread_mutex_destroy(&A) 00180 #define SSL_CTX_LOCK(A) pthread_mutex_lock(&A) 00181 #define SSL_CTX_UNLOCK(A) pthread_mutex_unlock(&A) 00182 #endif 00183 #else /* no mutexing */ 00184 #define SSL_CTX_MUTEX_INIT(A) 00185 #define SSL_CTX_MUTEX_DESTROY(A) 00186 #define SSL_CTX_LOCK(A) 00187 #define SSL_CTX_UNLOCK(A) 00188 #endif 00189 00190 #ifdef __cplusplus 00191 } 00192 #endif 00193 00194 #endif 00195 00196
Generated on Wed Jul 13 2022 19:30:07 by
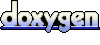