This is a fork of the mbed port of axTLS
Dependents: TLS_axTLS-Example HTTPSClientExample
TLSConnection.h
00001 #ifndef TLSCONNECTION_H 00002 #define TLSCONNECTION_H 00003 00004 #include "Socket/Socket.h" 00005 #include "Socket/Endpoint.h" 00006 #include "axTLS/ssl/ssl.h" 00007 00008 /** This class provides a user-friendly interface for the 00009 axTLS library. 00010 */ 00011 class TLSConnection : public Socket, public Endpoint 00012 { 00013 public : 00014 00015 TLSConnection(); 00016 00017 /** This function tries to establish a TLS connection 00018 with the given host. 00019 It will first try to establish a TCP connection on 00020 port 443 with the host. Then, it runs the TLS 00021 handshake protocol. 00022 00023 \param host A valid hostname (e.g. "mbed.org") 00024 \return True if it managed to establish a connection 00025 with the host. False otherwise. 00026 */ 00027 bool connect(const char *host); 00028 00029 /** Indicates whether a connection is established or not. 00030 00031 \return true if a connection is established, otherwise 00032 returns false. 00033 */ 00034 bool is_connected(void); 00035 00036 /** Sends some data to the host. This method does not return 00037 until length bytes have been sent. 00038 00039 \param data A pointer to some data 00040 \param length Number of bytes to send 00041 \return Number of bytes sent, or -1 if an error occured. 00042 */ 00043 int send_all(char *data, int length); 00044 00045 /** Receive some data from the host. 00046 00047 \param data 00048 \param length Maximum number of bytes to receive 00049 \return Number of bytes read in range 0..length, or -1 00050 if an error occured. 00051 */ 00052 int receive(char *data, int length); 00053 00054 /** Close the connection. 00055 00056 \param shutdown 00057 \return True if the connection was closed with success, 00058 false otherwise. If no connection was established, 00059 returns true immediately. 00060 */ 00061 bool close(bool shutdown = true); 00062 00063 private : 00064 00065 bool _is_connected; 00066 00067 SSL_CTX _ssl_ctx; 00068 SSL _ssl; 00069 }; 00070 00071 #endif 00072 00073
Generated on Wed Jul 13 2022 19:30:08 by
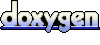