This library implements some hash and cryptographic algorithms.
Dependents: mBuinoBlinky PB_Emma_Ethernet SLOTrashHTTP Garagem ... more
TDES.cpp
00001 #include "TDES.h" 00002 00003 TDES::TDES(uint8_t *key1, uint8_t *key2, uint8_t *key3): 00004 BlockCipher(8,ECB_MODE), 00005 a(key1), 00006 b(key2), 00007 c(key3) 00008 { 00009 00010 } 00011 00012 TDES::TDES(uint8_t *key1, uint8_t *key2, uint8_t *key3, uint8_t *iv): 00013 BlockCipher(8,CBC_MODE,iv), 00014 a(key1), 00015 b(key2), 00016 c(key3) 00017 { 00018 00019 } 00020 00021 void TDES::encryptBlock(uint8_t *out, uint8_t *in) 00022 { 00023 uint8_t tmp[8], tmp2[8]; 00024 a.encryptBlock(tmp,in); 00025 b.decryptBlock(tmp2,tmp); 00026 c.encryptBlock(out, tmp2); 00027 } 00028 00029 void TDES::decryptBlock(uint8_t *out, uint8_t *in) 00030 { 00031 uint8_t tmp[8], tmp2[8]; 00032 c.decryptBlock(tmp, in); 00033 b.encryptBlock(tmp2, tmp); 00034 a.decryptBlock(out, tmp2); 00035 }
Generated on Tue Jul 12 2022 18:54:16 by
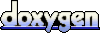