This library implements some hash and cryptographic algorithms.
Dependents: mBuinoBlinky PB_Emma_Ethernet SLOTrashHTTP Garagem ... more
AES.h
00001 #ifndef AES_H 00002 #define AES_H 00003 00004 #include "BlockCipher.h" 00005 00006 enum AES_TYPE 00007 { 00008 AES_128 = 4, 00009 AES_192 = 6, 00010 AES_256 = 8 00011 }; 00012 00013 class AES : public BlockCipher 00014 { 00015 public : 00016 00017 AES(const AES_TYPE type, uint8_t *key); 00018 AES(const AES_TYPE type, uint8_t *key, uint8_t *iv); 00019 00020 private : 00021 00022 virtual void encryptBlock(uint8_t *out, uint8_t *in); 00023 virtual void decryptBlock(uint8_t *out, uint8_t *in); 00024 00025 void keyExpansion(uint8_t *key); 00026 uint32_t rotWord(uint32_t w); 00027 uint32_t invRotWord(uint32_t w); 00028 uint32_t subWord(uint32_t w); 00029 void subBytes(); 00030 void invSubBytes(); 00031 void shiftRows(); 00032 void invShiftRows(); 00033 void mul(uint8_t *r); 00034 void invMul(uint8_t *r); 00035 void mixColumns(); 00036 void invMixColumns(); 00037 void addRoundKey(int round); 00038 00039 uint8_t state[16]; 00040 uint32_t w[60]; 00041 uint8_t nr,nk; 00042 }; 00043 00044 #endif
Generated on Tue Jul 12 2022 18:54:16 by
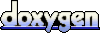