
This is a low-level network debugging utility that utilizes raw packet i/o to construct and deconstruct tcp, udp, ipv4, arp, and icmp packets over ethernet.
udp.h
00001 #ifndef UDP_H 00002 #define UDP_H 00003 00004 #include "net.h" 00005 00006 /** 00007 \file udp.h 00008 \brief UDP packet 00009 00010 This file contains the memory map and associated functions for UDP packet 00011 creation and deconstruction. 00012 */ 00013 00014 #define IPPROTO_UDP 0x11 00015 00016 /// UDP Packet memory map 00017 typedef struct { 00018 u16 source_port; ///< Source port (1-65535) 00019 u16 destination_port; ///< Destination port (1-65535) 00020 u16 length; ///< Entire datagram size in bytes 00021 u16 checksum; ///< Checksum 00022 u8 data[]; ///< Data memory map 00023 } UDP_Packet; 00024 00025 /// Convert from wire to host or host to wire endianness 00026 inline void fix_endian_udp(UDP_Packet *segment) 00027 { 00028 fix_endian_u16(&segment->source_port); 00029 fix_endian_u16(&segment->destination_port); 00030 fix_endian_u16(&segment->length); 00031 } 00032 00033 /// Print the UDP packet 00034 inline void print_udp(UDP_Packet *segment) 00035 { 00036 main_log.printf("UDP Packet:"); 00037 main_log.printf(" Source: PORT %d", segment->source_port); 00038 main_log.printf(" Dest: PORT %d", segment->destination_port); 00039 main_log.printf(" Length: %d", segment->length); 00040 } 00041 00042 #endif
Generated on Tue Jul 12 2022 11:59:40 by
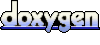