Add functions to get Mx, My and Mz.
Dependents: 9DOF-Stick aigamozu_program_ver2 aigamozu_program_ver2_yokokawa aigamozu_auto_ver1 ... more
HMC5843.cpp
00001 /** 00002 * @author Jose R. Padron 00003 * @author Used HMC6352 library developed by Aaron Berk as template 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * Honeywell HMC5843digital compass. 00029 * 00030 * Datasheet: 00031 * 00032 * http://www.ssec.honeywell.com/magnetic/datasheets/HMC5843.pdf 00033 */ 00034 00035 /** 00036 * Includes 00037 */ 00038 #include "HMC5843.h" 00039 00040 HMC5843::HMC5843(PinName sda, PinName scl) { 00041 00042 i2c_ = new I2C(sda, scl); 00043 //100KHz, as specified by the datasheet. 00044 i2c_->frequency(100000); 00045 00046 00047 } 00048 00049 00050 void HMC5843::write(int address, int data) { 00051 00052 char tx[2]; 00053 00054 tx[0]=address; 00055 tx[1]=data; 00056 00057 i2c_->write(HMC5843_I2C_WRITE,tx,2); 00058 00059 wait_ms(100); 00060 00061 } 00062 00063 00064 void HMC5843::setSleepMode() { 00065 00066 write(HMC5843_MODE, HMC5843_SLEEP); 00067 } 00068 00069 void HMC5843::setDefault(void) { 00070 00071 write(HMC5843_CONFIG_A,HMC5843_10HZ_NORMAL); 00072 write(HMC5843_CONFIG_B,HMC5843_1_0GA); 00073 write(HMC5843_MODE,HMC5843_CONTINUOUS); 00074 wait_ms(100); 00075 } 00076 00077 00078 void HMC5843::getAddress(char *buffer) { 00079 00080 char rx[3]; 00081 char tx[1]; 00082 tx[0]=HMC5843_IDENT_A; 00083 00084 00085 i2c_->write(HMC5843_I2C_WRITE, tx,1); 00086 00087 wait_ms(1); 00088 00089 i2c_->read(HMC5843_I2C_READ,rx,3); 00090 00091 buffer[0]=rx[0]; 00092 buffer[1]=rx[1]; 00093 buffer[2]=rx[2]; 00094 } 00095 00096 00097 00098 void HMC5843::setOpMode(int mode, int ConfigA, int ConfigB) { 00099 00100 00101 write(HMC5843_CONFIG_A,ConfigA); 00102 write(HMC5843_CONFIG_B,ConfigB); 00103 write(HMC5843_MODE,mode); 00104 00105 00106 } 00107 00108 00109 00110 00111 void HMC5843::readData(int* readings) { 00112 00113 00114 char tx[1]; 00115 char rx[2]; 00116 00117 00118 tx[0]=HMC5843_X_MSB; 00119 i2c_->write(HMC5843_I2C_READ,tx,1); 00120 i2c_->read(HMC5843_I2C_READ,rx,2); 00121 readings[0]= (int)rx[0]<<8|(int)rx[1]; 00122 00123 00124 tx[0]=HMC5843_Y_MSB; 00125 i2c_->write(HMC5843_I2C_READ,tx,1); 00126 i2c_->read(HMC5843_I2C_READ,rx,2); 00127 readings[1]= (int)rx[0]<<8|(int)rx[1]; 00128 00129 tx[0]=HMC5843_Z_MSB; 00130 i2c_->write(HMC5843_I2C_READ,tx,1); 00131 i2c_->read(HMC5843_I2C_READ,rx,2); 00132 readings[2]= (int)rx[0]<<8|(int)rx[1]; 00133 00134 } 00135 00136 int HMC5843::getMx() { 00137 00138 char tx[1]; 00139 char rx[2]; 00140 00141 00142 tx[0]=HMC5843_X_MSB; 00143 i2c_->write(HMC5843_I2C_READ,tx,1); 00144 i2c_->read(HMC5843_I2C_READ,rx,2); 00145 return ((int)rx[0]<<8|(int)rx[1]); 00146 00147 } 00148 00149 int HMC5843::getMy() { 00150 00151 char tx[1]; 00152 char rx[2]; 00153 00154 00155 tx[0]=HMC5843_Y_MSB; 00156 i2c_->write(HMC5843_I2C_READ,tx,1); 00157 i2c_->read(HMC5843_I2C_READ,rx,2); 00158 return ((int)rx[0]<<8|(int)rx[1]); 00159 00160 } 00161 00162 00163 int HMC5843::getMz(){ 00164 00165 char tx[1]; 00166 char rx[2]; 00167 00168 00169 tx[0]=HMC5843_Z_MSB; 00170 i2c_->write(HMC5843_I2C_READ,tx,1); 00171 i2c_->read(HMC5843_I2C_READ,rx,2); 00172 return ((int)rx[0]<<8|(int)rx[1]); 00173 00174 } 00175 00176
Generated on Tue Jul 12 2022 23:07:16 by
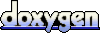