Generic driver for the RWD RFID Modules from IB Technology.
Embed:
(wiki syntax)
Show/hide line numbers
RWDModule.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef RWD_MODULE_H 00025 #define RWD_MODULE_H 00026 00027 #include "mbed.h" 00028 00029 //!Generic driver for the RWD RFID Modules from IB Technology. 00030 /*! 00031 The RWD modules from IB Technology are RFID readers working with different frequencies and protocols but with a common instructions set and pinout. 00032 */ 00033 class RWDModule 00034 { 00035 public: 00036 //!Creates an instance of the class. 00037 /*! 00038 Connect module using serial port pins tx, rx and DigitalIn pin cts (clear-to-send). 00039 */ 00040 RWDModule(PinName tx, PinName rx, PinName cts); 00041 00042 /*! 00043 Destroys instance. 00044 */ 00045 virtual ~RWDModule (); 00046 00047 //!Executes a command. 00048 /*! 00049 Executes the command cmd on the reader, with parameters set in params buffer of paramsLen length. The acknowledge byte sent back by the reader masked with ackOkMask must be equal to ackOk for the command to be considered a success. If so, the result is stored in buffer resp of length respLen. 00050 This is a non-blocking function, and ready() should be called to check completion. 00051 Please note that the buffers references must remain valid until the command has been executed. 00052 */ 00053 void command(uint8_t cmd, const uint8_t* params, int paramsLen, uint8_t* resp, size_t respLen, uint8_t ackOk, size_t ackOkMask); //Ack Byte is not included in the resp buf 00054 00055 //!Ready for a command / response is available. 00056 /*! 00057 Returns true if the previous command has been executed and an other command is ready to be sent. 00058 */ 00059 bool ready(); 00060 00061 //!Get whether last command was succesful, and complete ack byte if a ptr is provided. 00062 /*! 00063 Returns true if the previous command was successful. If pAck is provided, the actual acknowledge byte returned by the reader is stored in that variable. 00064 */ 00065 bool result(uint8_t* pAck = NULL); 00066 00067 private: 00068 void intClearToSend(); //Called on interrupt when CTS line falls 00069 void intTx(); //Called on interrupt when TX buffer is not full anymore (bytes sent) 00070 void intRx(); //Called on interrrupt when RX buffer is not empty anymore (bytes received) 00071 00072 Serial m_serial; 00073 InterruptIn m_cts; 00074 00075 uint8_t m_cmd; 00076 uint8_t* m_paramsBuf; 00077 uint8_t* m_respBuf; 00078 size_t m_pos; 00079 size_t m_paramsLen; 00080 size_t m_respLen; 00081 00082 uint8_t m_ackOk; 00083 uint8_t m_ackOkMask; 00084 00085 uint8_t m_ack; 00086 00087 enum 00088 { 00089 READY, 00090 CMD_QUEUED, 00091 SENDING_CMD, 00092 WAITING_FOR_ACK, 00093 RECEIVING_ACK 00094 } m_state; 00095 00096 }; 00097 00098 #endif
Generated on Thu Jul 14 2022 03:09:25 by
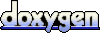