
This example establishes a transparent link between the mbed serial port and the modem (LISA or SARA) on the C027. You can use it to use the standard u-blox tools such as m-center or any terminal program. These tools can then connect to the serial port and talk directly to the modem. Baudrate should be set to 115200 baud and is fixed. m-center can be downloaded from u-blox website following this link: http://www.u-blox.com/en/evaluation-tools-a-software/u-center/m-center.html
Fork of C027_ModemTransparentSerial by
main.cpp
00001 #include "mbed.h" 00002 #include "C027.h" 00003 00004 DigitalOut mdm_activity(LED); 00005 00006 int main() 00007 { 00008 int led_toggle_count = 5; 00009 00010 C027 c027; 00011 00012 // enable, and use the uart 00013 c027.mdmPower(true,true); 00014 00015 // enable the GPS. 00016 c027.gpsPower(true, false); 00017 00018 #if 0 00019 while(1) { 00020 mdm_activity = !mdm_activity; 00021 wait(0.2); 00022 } 00023 #else 00024 while( led_toggle_count-- > 0 ) 00025 { 00026 mdm_activity = !mdm_activity; 00027 wait(0.2); 00028 } 00029 #endif 00030 00031 // open the mdm serial port 00032 Serial mdm(MDMTXD, MDMRXD); 00033 mdm.baud(MDMBAUD); 00034 // tell the modem that we can always receive data 00035 DigitalOut mdmRts(MDMRTS); 00036 mdmRts = 0; // (not using flow control) 00037 00038 // open the PC serial port and (use the same baudrate) 00039 Serial pc(USBTX, USBRX); 00040 pc.baud(MDMBAUD); 00041 00042 pc.printf( "M3->UART connection ready\r\n"); 00043 00044 while (1) 00045 { 00046 // transfer data from pc to modem 00047 if (pc.readable() && mdm.writeable()) 00048 mdm.putc(pc.getc()); 00049 // transfer data from modem to pc 00050 if (mdm.readable() && pc.writeable()) 00051 pc.putc(mdm.getc()); 00052 } 00053 }
Generated on Mon Aug 15 2022 12:05:05 by
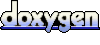