CC3000HostDriver for device TI CC3000 some changes were made due to mbed compiler and the use of void*
Embed:
(wiki syntax)
Show/hide line numbers
wlan.cpp
00001 /***************************************************************************** 00002 * 00003 * wlan.c - CC3000 Host Driver Implementation. 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 00036 //***************************************************************************** 00037 // 00038 //! \addtogroup wlan_api 00039 //! @{ 00040 // 00041 //***************************************************************************** 00042 #include <string.h> 00043 #include "wlan.h" 00044 #include "hci.h" 00045 #include "mbed.h" 00046 #include "spi.h" 00047 #include "socket.h" 00048 #include "nvmem.h" 00049 #include "security.h" 00050 #include "evnt_handler.h" 00051 00052 00053 volatile sSimplLinkInformation tSLInformation; 00054 00055 #define SMART_CONFIG_PROFILE_SIZE 67 // 67 = 32 (max ssid) + 32 (max key) + 1 (SSID length) + 1 (security type) + 1 (key length) 00056 00057 #ifndef CC3000_UNENCRYPTED_SMART_CONFIG 00058 unsigned char key[AES128_KEY_SIZE]; 00059 unsigned char profileArray[SMART_CONFIG_PROFILE_SIZE]; 00060 #endif //CC3000_UNENCRYPTED_SMART_CONFIG 00061 00062 /* patches type */ 00063 #define PATCHES_HOST_TYPE_WLAN_DRIVER 0x01 00064 #define PATCHES_HOST_TYPE_WLAN_FW 0x02 00065 #define PATCHES_HOST_TYPE_BOOTLOADER 0x03 00066 00067 #define SL_SET_SCAN_PARAMS_INTERVAL_LIST_SIZE (16) 00068 #define SL_SIMPLE_CONFIG_PREFIX_LENGTH (3) 00069 #define ETH_ALEN (6) 00070 #define MAXIMAL_SSID_LENGTH (32) 00071 00072 #define SL_PATCHES_REQUEST_DEFAULT (0) 00073 #define SL_PATCHES_REQUEST_FORCE_HOST (1) 00074 #define SL_PATCHES_REQUEST_FORCE_NONE (2) 00075 00076 00077 #define WLAN_SEC_UNSEC (0) 00078 #define WLAN_SEC_WEP (1) 00079 #define WLAN_SEC_WPA (2) 00080 #define WLAN_SEC_WPA2 (3) 00081 00082 00083 #define WLAN_SL_INIT_START_PARAMS_LEN (1) 00084 #define WLAN_PATCH_PARAMS_LENGTH (8) 00085 #define WLAN_SET_CONNECTION_POLICY_PARAMS_LEN (12) 00086 #define WLAN_DEL_PROFILE_PARAMS_LEN (4) 00087 #define WLAN_SET_MASK_PARAMS_LEN (4) 00088 #define WLAN_SET_SCAN_PARAMS_LEN (100) 00089 #define WLAN_GET_SCAN_RESULTS_PARAMS_LEN (4) 00090 #define WLAN_ADD_PROFILE_NOSEC_PARAM_LEN (24) 00091 #define WLAN_ADD_PROFILE_WEP_PARAM_LEN (36) 00092 #define WLAN_ADD_PROFILE_WPA_PARAM_LEN (44) 00093 #define WLAN_CONNECT_PARAM_LEN (29) 00094 #define WLAN_SMART_CONFIG_START_PARAMS_LEN (4) 00095 00096 00097 00098 00099 //***************************************************************************** 00100 // 00101 //! SimpleLink_Init_Start 00102 //! 00103 //! @param usPatchesAvailableAtHost flag to indicate if patches available 00104 //! from host or from EEPROM. Due to the 00105 //! fact the patches are burn to the EEPROM 00106 //! using the patch programmer utility, the 00107 //! patches will be available from the EEPROM 00108 //! and not from the host. 00109 //! 00110 //! @return none 00111 //! 00112 //! @brief Send HCI_CMND_SIMPLE_LINK_START to CC3000 00113 // 00114 //***************************************************************************** 00115 static void SimpleLink_Init_Start(unsigned short usPatchesAvailableAtHost) 00116 { 00117 unsigned char *ptr; 00118 unsigned char *args; 00119 00120 ptr = tSLInformation.pucTxCommandBuffer; 00121 args = (unsigned char *)(ptr + HEADERS_SIZE_CMD); 00122 00123 UINT8_TO_STREAM(args, ((usPatchesAvailableAtHost) ? SL_PATCHES_REQUEST_FORCE_HOST : SL_PATCHES_REQUEST_DEFAULT)); 00124 00125 // IRQ Line asserted - send HCI_CMND_SIMPLE_LINK_START to CC3000 00126 hci_command_send(HCI_CMND_SIMPLE_LINK_START, ptr, WLAN_SL_INIT_START_PARAMS_LEN); 00127 00128 SimpleLinkWaitEvent(HCI_CMND_SIMPLE_LINK_START, 0); 00129 00130 } 00131 00132 00133 00134 //***************************************************************************** 00135 // 00136 //! wlan_init 00137 //! 00138 //! @param sWlanCB Asynchronous events callback. 00139 //! 0 no event call back. 00140 //! -call back parameters: 00141 //! 1) event_type: HCI_EVNT_WLAN_UNSOL_CONNECT connect event, 00142 //! HCI_EVNT_WLAN_UNSOL_DISCONNECT disconnect event, 00143 //! HCI_EVNT_WLAN_ASYNC_SIMPLE_CONFIG_DONE config done, 00144 //! HCI_EVNT_WLAN_UNSOL_DHCP dhcp report, 00145 //! HCI_EVNT_WLAN_ASYNC_PING_REPORT ping report OR 00146 //! HCI_EVNT_WLAN_KEEPALIVE keepalive. 00147 //! 2) data: pointer to extra data that received by the event 00148 //! (NULL no data). 00149 //! 3) length: data length. 00150 //! -Events with extra data: 00151 //! HCI_EVNT_WLAN_UNSOL_DHCP: 4 bytes IP, 4 bytes Mask, 00152 //! 4 bytes default gateway, 4 bytes DHCP server and 4 bytes 00153 //! for DNS server. 00154 //! HCI_EVNT_WLAN_ASYNC_PING_REPORT: 4 bytes Packets sent, 00155 //! 4 bytes Packets received, 4 bytes Min round time, 00156 //! 4 bytes Max round time and 4 bytes for Avg round time. 00157 //! 00158 //! @param sFWPatches 0 no patch or pointer to FW patches 00159 //! @param sDriverPatches 0 no patch or pointer to driver patches 00160 //! @param sBootLoaderPatches 0 no patch or pointer to bootloader patches 00161 //! @param sReadWlanInterruptPin init callback. the callback read wlan 00162 //! interrupt status. 00163 //! @param sWlanInterruptEnable init callback. the callback enable wlan 00164 //! interrupt. 00165 //! @param sWlanInterruptDisable init callback. the callback disable wlan 00166 //! interrupt. 00167 //! @param sWriteWlanPin init callback. the callback write value 00168 //! to device pin. 00169 //! 00170 //! @return none 00171 //! 00172 //! @sa wlan_set_event_mask , wlan_start , wlan_stop 00173 //! 00174 //! @brief Initialize wlan driver 00175 //! 00176 //! @warning This function must be called before ANY other wlan driver function 00177 // 00178 //***************************************************************************** 00179 00180 void wlan_init( tWlanCB sWlanCB, 00181 tFWPatches sFWPatches, 00182 tDriverPatches sDriverPatches, 00183 tBootLoaderPatches sBootLoaderPatches, 00184 tWlanReadInteruptPin sReadWlanInterruptPin, 00185 tWlanInterruptEnable sWlanInterruptEnable, 00186 tWlanInterruptDisable sWlanInterruptDisable, 00187 tWriteWlanPin sWriteWlanPin) 00188 { 00189 00190 tSLInformation.sFWPatches = sFWPatches; 00191 tSLInformation.sDriverPatches = sDriverPatches; 00192 tSLInformation.sBootLoaderPatches = sBootLoaderPatches; 00193 00194 // init io callback 00195 tSLInformation.ReadWlanInterruptPin = sReadWlanInterruptPin; 00196 tSLInformation.WlanInterruptEnable = sWlanInterruptEnable; 00197 tSLInformation.WlanInterruptDisable = sWlanInterruptDisable; 00198 tSLInformation.WriteWlanPin = sWriteWlanPin; 00199 00200 //init asynchronous events callback 00201 tSLInformation.sWlanCB= sWlanCB; 00202 00203 // By default TX Complete events are routed to host too 00204 tSLInformation.InformHostOnTxComplete = 1; 00205 } 00206 00207 //***************************************************************************** 00208 // 00209 //! SpiReceiveHandler 00210 //! 00211 //! @param pvBuffer - pointer to the received data buffer 00212 //! The function triggers Received event/data processing 00213 //! 00214 //! @param Pointer to the received data 00215 //! @return none 00216 //! 00217 //! @brief The function triggers Received event/data processing. It is 00218 //! called from the SPI library to receive the data 00219 // 00220 //***************************************************************************** 00221 void SpiReceiveHandler(void *pvBuffer) 00222 { 00223 tSLInformation.usEventOrDataReceived = 1; 00224 tSLInformation.pucReceivedData = (unsigned char *)pvBuffer; 00225 00226 hci_unsolicited_event_handler(); 00227 } 00228 00229 00230 //***************************************************************************** 00231 // 00232 //! wlan_start 00233 //! 00234 //! @param usPatchesAvailableAtHost - flag to indicate if patches available 00235 //! from host or from EEPROM. Due to the 00236 //! fact the patches are burn to the EEPROM 00237 //! using the patch programmer utility, the 00238 //! patches will be available from the EEPROM 00239 //! and not from the host. 00240 //! 00241 //! @return none 00242 //! 00243 //! @brief Start WLAN device. This function asserts the enable pin of 00244 //! the device (WLAN_EN), starting the HW initialization process. 00245 //! The function blocked until device Initialization is completed. 00246 //! Function also configure patches (FW, driver or bootloader) 00247 //! and calls appropriate device callbacks. 00248 //! 00249 //! @Note Prior calling the function wlan_init shall be called. 00250 //! @Warning This function must be called after wlan_init and before any 00251 //! other wlan API 00252 //! @sa wlan_init , wlan_stop 00253 //! 00254 // 00255 //***************************************************************************** 00256 00257 void 00258 wlan_start(unsigned short usPatchesAvailableAtHost) 00259 { 00260 00261 unsigned long ulSpiIRQState; 00262 00263 tSLInformation.NumberOfSentPackets = 0; 00264 tSLInformation.NumberOfReleasedPackets = 0; 00265 tSLInformation.usRxEventOpcode = 0; 00266 tSLInformation.usNumberOfFreeBuffers = 0; 00267 tSLInformation.usSlBufferLength = 0; 00268 tSLInformation.usBufferSize = 0; 00269 tSLInformation.usRxDataPending = 0; 00270 tSLInformation.slTransmitDataError = 0; 00271 tSLInformation.usEventOrDataReceived = 0; 00272 tSLInformation.pucReceivedData = 0; 00273 00274 // Allocate the memory for the RX/TX data transactions 00275 tSLInformation.pucTxCommandBuffer = (unsigned char *)wlan_tx_buffer; 00276 00277 // init spi 00278 SpiOpen(SpiReceiveHandler); 00279 00280 // Check the IRQ line 00281 ulSpiIRQState = tSLInformation.ReadWlanInterruptPin(); 00282 00283 // ASIC 1273 chip enable: toggle WLAN EN line 00284 tSLInformation.WriteWlanPin( WLAN_ENABLE ); 00285 00286 if (ulSpiIRQState) 00287 { 00288 // wait till the IRQ line goes low 00289 while(tSLInformation.ReadWlanInterruptPin() != 0) 00290 { 00291 } 00292 00293 } 00294 else 00295 { 00296 // ASIC 1273 chip enable: toggle WLAN EN line 00297 tSLInformation.WriteWlanPin( WLAN_DISABLE ); 00298 // wait till the IRQ line goes high and than low 00299 while(tSLInformation.ReadWlanInterruptPin() == 0) 00300 { 00301 } 00302 // ASIC 1273 chip enable: toggle WLAN EN line 00303 tSLInformation.WriteWlanPin( WLAN_ENABLE ); 00304 while(tSLInformation.ReadWlanInterruptPin() != 0) 00305 { 00306 } 00307 } 00308 00309 SimpleLink_Init_Start(usPatchesAvailableAtHost); 00310 00311 // Read Buffer's size and finish 00312 hci_command_send(HCI_CMND_READ_BUFFER_SIZE, tSLInformation.pucTxCommandBuffer, 0); 00313 00314 SimpleLinkWaitEvent(HCI_CMND_READ_BUFFER_SIZE, 0); 00315 00316 } 00317 00318 00319 //***************************************************************************** 00320 // 00321 //! wlan_stop 00322 //! 00323 //! @param none 00324 //! 00325 //! @return none 00326 //! 00327 //! @brief Stop WLAN device by putting it into reset state. 00328 //! 00329 //! @sa wlan_start 00330 // 00331 //***************************************************************************** 00332 00333 void 00334 wlan_stop(void) 00335 { 00336 // ASIC 1273 chip disable 00337 tSLInformation.WriteWlanPin( WLAN_DISABLE ); 00338 00339 // Wait till IRQ line goes high... 00340 while(tSLInformation.ReadWlanInterruptPin() == 0) 00341 { 00342 } 00343 00344 // Free the used by WLAN Driver memory 00345 if (tSLInformation.pucTxCommandBuffer) 00346 { 00347 tSLInformation.pucTxCommandBuffer = 0; 00348 } 00349 00350 SpiClose(); 00351 } 00352 00353 00354 //***************************************************************************** 00355 // 00356 //! wlan_connect 00357 //! 00358 //! @param sec_type security options: 00359 //! WLAN_SEC_UNSEC, 00360 //! WLAN_SEC_WEP (ASCII support only), 00361 //! WLAN_SEC_WPA or WLAN_SEC_WPA2 00362 //! @param ssid up to 32 bytes and is ASCII SSID of the AP 00363 //! @param ssid_len length of the SSID 00364 //! @param bssid 6 bytes specified the AP bssid 00365 //! @param key up to 16 bytes specified the AP security key 00366 //! @param key_len key length 00367 //! 00368 //! @return On success, zero is returned. On error, negative is returned. 00369 //! Note that even though a zero is returned on success to trigger 00370 //! connection operation, it does not mean that CCC3000 is already 00371 //! connected. An asynchronous "Connected" event is generated when 00372 //! actual association process finishes and CC3000 is connected to 00373 //! the AP. If DHCP is set, An asynchronous "DHCP" event is 00374 //! generated when DHCP process is finish. 00375 //! 00376 //! 00377 //! @brief Connect to AP 00378 //! @warning Please Note that when connection to AP configured with security 00379 //! type WEP, please confirm that the key is set as ASCII and not 00380 //! as HEX. 00381 //! @sa wlan_disconnect 00382 // 00383 //***************************************************************************** 00384 00385 #ifndef CC3000_TINY_DRIVER 00386 long 00387 wlan_connect(unsigned long ulSecType, char *ssid, long ssid_len, unsigned char *bssid, unsigned char *key, long key_len) 00388 { 00389 long ret; 00390 unsigned char *ptr; 00391 unsigned char *args; 00392 unsigned char bssid_zero[] = {0, 0, 0, 0, 0, 0}; 00393 00394 ret = EFAIL; 00395 ptr = tSLInformation.pucTxCommandBuffer; 00396 args = (ptr + HEADERS_SIZE_CMD); 00397 00398 // Fill in command buffer 00399 args = UINT32_TO_STREAM(args, 0x0000001c); 00400 args = UINT32_TO_STREAM(args, ssid_len); 00401 args = UINT32_TO_STREAM(args, ulSecType); 00402 args = UINT32_TO_STREAM(args, 0x00000010 + ssid_len); 00403 args = UINT32_TO_STREAM(args, key_len); 00404 args = UINT16_TO_STREAM(args, 0); 00405 00406 // padding shall be zeroed 00407 if(bssid) 00408 { 00409 ARRAY_TO_STREAM(args, bssid, ETH_ALEN); 00410 } 00411 else 00412 { 00413 ARRAY_TO_STREAM(args, bssid_zero, ETH_ALEN); 00414 } 00415 00416 ARRAY_TO_STREAM(args, ssid, ssid_len); 00417 00418 if(key_len && key) 00419 { 00420 ARRAY_TO_STREAM(args, key, key_len); 00421 } 00422 00423 // Initiate a HCI command 00424 hci_command_send(HCI_CMND_WLAN_CONNECT, ptr, WLAN_CONNECT_PARAM_LEN + ssid_len + key_len - 1); 00425 00426 // Wait for command complete event 00427 SimpleLinkWaitEvent(HCI_CMND_WLAN_CONNECT, &ret); 00428 errno = ret; 00429 00430 return(ret); 00431 } 00432 #else 00433 long 00434 wlan_connect(char *ssid, long ssid_len) 00435 { 00436 long ret; 00437 unsigned char *ptr; 00438 unsigned char *args; 00439 unsigned char bssid_zero[] = {0, 0, 0, 0, 0, 0}; 00440 00441 ret = EFAIL; 00442 ptr = tSLInformation.pucTxCommandBuffer; 00443 args = (ptr + HEADERS_SIZE_CMD); 00444 00445 // Fill in command buffer 00446 args = UINT32_TO_STREAM(args, 0x0000001c); 00447 args = UINT32_TO_STREAM(args, ssid_len); 00448 args = UINT32_TO_STREAM(args, 0); 00449 args = UINT32_TO_STREAM(args, 0x00000010 + ssid_len); 00450 args = UINT32_TO_STREAM(args, 0); 00451 args = UINT16_TO_STREAM(args, 0); 00452 00453 // padding shall be zeroed 00454 ARRAY_TO_STREAM(args, bssid_zero, ETH_ALEN); 00455 ARRAY_TO_STREAM(args, ssid, ssid_len); 00456 00457 // Initiate a HCI command 00458 hci_command_send(HCI_CMND_WLAN_CONNECT, ptr, WLAN_CONNECT_PARAM_LEN + ssid_len - 1); 00459 00460 // Wait for command complete event 00461 SimpleLinkWaitEvent(HCI_CMND_WLAN_CONNECT, &ret); 00462 errno = ret; 00463 00464 return(ret); 00465 } 00466 #endif 00467 00468 //***************************************************************************** 00469 // 00470 //! wlan_disconnect 00471 //! 00472 //! @return 0 disconnected done, other CC3000 already disconnected 00473 //! 00474 //! @brief Disconnect connection from AP. 00475 //! 00476 //! @sa wlan_connect 00477 // 00478 //***************************************************************************** 00479 00480 long 00481 wlan_disconnect() 00482 { 00483 long ret; 00484 unsigned char *ptr; 00485 00486 ret = EFAIL; 00487 ptr = tSLInformation.pucTxCommandBuffer; 00488 00489 hci_command_send(HCI_CMND_WLAN_DISCONNECT, ptr, 0); 00490 00491 // Wait for command complete event 00492 SimpleLinkWaitEvent(HCI_CMND_WLAN_DISCONNECT, &ret); 00493 errno = ret; 00494 00495 return(ret); 00496 } 00497 00498 //***************************************************************************** 00499 // 00500 //! wlan_ioctl_set_connection_policy 00501 //! 00502 //! @param should_connect_to_open_ap enable(1), disable(0) connect to any 00503 //! available AP. This parameter corresponds to the configuration of 00504 //! item # 3 in the brief description. 00505 //! @param should_use_fast_connect enable(1), disable(0). if enabled, tries 00506 //! to connect to the last connected AP. This parameter corresponds 00507 //! to the configuration of item # 1 in the brief description. 00508 //! @param auto_start enable(1), disable(0) auto connect 00509 //! after reset and periodically reconnect if needed. This 00510 //! configuration configures option 2 in the above description. 00511 //! 00512 //! @return On success, zero is returned. On error, -1 is returned 00513 //! 00514 //! @brief When auto is enabled, the device tries to connect according 00515 //! the following policy: 00516 //! 1) If fast connect is enabled and last connection is valid, 00517 //! the device will try to connect to it without the scanning 00518 //! procedure (fast). The last connection will be marked as 00519 //! invalid, due to adding/removing profile. 00520 //! 2) If profile exists, the device will try to connect it 00521 //! (Up to seven profiles). 00522 //! 3) If fast and profiles are not found, and open mode is 00523 //! enabled, the device will try to connect to any AP. 00524 //! * Note that the policy settings are stored in the CC3000 NVMEM. 00525 //! 00526 //! @sa wlan_add_profile , wlan_ioctl_del_profile 00527 // 00528 //***************************************************************************** 00529 00530 long 00531 wlan_ioctl_set_connection_policy(unsigned long should_connect_to_open_ap, 00532 unsigned long ulShouldUseFastConnect, 00533 unsigned long ulUseProfiles) 00534 { 00535 long ret; 00536 unsigned char *ptr; 00537 unsigned char *args; 00538 00539 ret = EFAIL; 00540 ptr = tSLInformation.pucTxCommandBuffer; 00541 args = (unsigned char *)(ptr + HEADERS_SIZE_CMD); 00542 00543 // Fill in HCI packet structure 00544 args = UINT32_TO_STREAM(args, should_connect_to_open_ap); 00545 args = UINT32_TO_STREAM(args, ulShouldUseFastConnect); 00546 args = UINT32_TO_STREAM(args, ulUseProfiles); 00547 00548 // Initiate a HCI command 00549 hci_command_send(HCI_CMND_WLAN_IOCTL_SET_CONNECTION_POLICY, 00550 ptr, WLAN_SET_CONNECTION_POLICY_PARAMS_LEN); 00551 00552 // Wait for command complete event 00553 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_SET_CONNECTION_POLICY, &ret); 00554 00555 return(ret); 00556 } 00557 00558 //***************************************************************************** 00559 // 00560 //! wlan_add_profile 00561 //! 00562 //! @param ulSecType WLAN_SEC_UNSEC,WLAN_SEC_WEP,WLAN_SEC_WPA,WLAN_SEC_WPA2 00563 //! @param ucSsid ssid SSID up to 32 bytes 00564 //! @param ulSsidLen ssid length 00565 //! @param ucBssid bssid 6 bytes 00566 //! @param ulPriority ulPriority profile priority. Lowest priority:0. 00567 //! @param ulPairwiseCipher_Or_TxKeyLen key length for WEP security 00568 //! @param ulGroupCipher_TxKeyIndex key index 00569 //! @param ulKeyMgmt KEY management 00570 //! @param ucPf_OrKey security key 00571 //! @param ulPassPhraseLen security key length for WPA\WPA2 00572 //! 00573 //! @return On success, zero is returned. On error, -1 is returned 00574 //! 00575 //! @brief When auto start is enabled, the device connects to 00576 //! station from the profiles table. Up to 7 profiles are supported. 00577 //! If several profiles configured the device choose the highest 00578 //! priority profile, within each priority group, device will choose 00579 //! profile based on security policy, signal strength, etc 00580 //! parameters. All the profiles are stored in CC3000 NVMEM. 00581 //! 00582 //! @sa wlan_ioctl_del_profile 00583 // 00584 //***************************************************************************** 00585 00586 #ifndef CC3000_TINY_DRIVER 00587 long 00588 wlan_add_profile(unsigned long ulSecType, 00589 unsigned char* ucSsid, 00590 unsigned long ulSsidLen, 00591 unsigned char *ucBssid, 00592 unsigned long ulPriority, 00593 unsigned long ulPairwiseCipher_Or_TxKeyLen, 00594 unsigned long ulGroupCipher_TxKeyIndex, 00595 unsigned long ulKeyMgmt, 00596 unsigned char* ucPf_OrKey, 00597 unsigned long ulPassPhraseLen) 00598 { 00599 unsigned short arg_len; 00600 long ret; 00601 unsigned char *ptr; 00602 long i = 0; 00603 unsigned char *args; 00604 unsigned char bssid_zero[] = {0, 0, 0, 0, 0, 0}; 00605 00606 ptr = tSLInformation.pucTxCommandBuffer; 00607 args = (ptr + HEADERS_SIZE_CMD); 00608 00609 args = UINT32_TO_STREAM(args, ulSecType); 00610 00611 // Setup arguments in accordance with the security type 00612 switch (ulSecType) 00613 { 00614 //OPEN 00615 case WLAN_SEC_UNSEC: 00616 { 00617 args = UINT32_TO_STREAM(args, 0x00000014); 00618 args = UINT32_TO_STREAM(args, ulSsidLen); 00619 args = UINT16_TO_STREAM(args, 0); 00620 if(ucBssid) 00621 { 00622 ARRAY_TO_STREAM(args, ucBssid, ETH_ALEN); 00623 } 00624 else 00625 { 00626 ARRAY_TO_STREAM(args, bssid_zero, ETH_ALEN); 00627 } 00628 args = UINT32_TO_STREAM(args, ulPriority); 00629 ARRAY_TO_STREAM(args, ucSsid, ulSsidLen); 00630 00631 arg_len = WLAN_ADD_PROFILE_NOSEC_PARAM_LEN + ulSsidLen; 00632 } 00633 break; 00634 00635 //WEP 00636 case WLAN_SEC_WEP: 00637 { 00638 args = UINT32_TO_STREAM(args, 0x00000020); 00639 args = UINT32_TO_STREAM(args, ulSsidLen); 00640 args = UINT16_TO_STREAM(args, 0); 00641 if(ucBssid) 00642 { 00643 ARRAY_TO_STREAM(args, ucBssid, ETH_ALEN); 00644 } 00645 else 00646 { 00647 ARRAY_TO_STREAM(args, bssid_zero, ETH_ALEN); 00648 } 00649 args = UINT32_TO_STREAM(args, ulPriority); 00650 args = UINT32_TO_STREAM(args, 0x0000000C + ulSsidLen); 00651 args = UINT32_TO_STREAM(args, ulPairwiseCipher_Or_TxKeyLen); 00652 args = UINT32_TO_STREAM(args, ulGroupCipher_TxKeyIndex); 00653 ARRAY_TO_STREAM(args, ucSsid, ulSsidLen); 00654 00655 for(i = 0; i < 4; i++) 00656 { 00657 unsigned char *p = &ucPf_OrKey[i * ulPairwiseCipher_Or_TxKeyLen]; 00658 00659 ARRAY_TO_STREAM(args, p, ulPairwiseCipher_Or_TxKeyLen); 00660 } 00661 00662 arg_len = WLAN_ADD_PROFILE_WEP_PARAM_LEN + ulSsidLen + 00663 ulPairwiseCipher_Or_TxKeyLen * 4; 00664 00665 } 00666 break; 00667 00668 //WPA 00669 //WPA2 00670 case WLAN_SEC_WPA: 00671 case WLAN_SEC_WPA2: 00672 { 00673 args = UINT32_TO_STREAM(args, 0x00000028); 00674 args = UINT32_TO_STREAM(args, ulSsidLen); 00675 args = UINT16_TO_STREAM(args, 0); 00676 if(ucBssid) 00677 { 00678 ARRAY_TO_STREAM(args, ucBssid, ETH_ALEN); 00679 } 00680 else 00681 { 00682 ARRAY_TO_STREAM(args, bssid_zero, ETH_ALEN); 00683 } 00684 args = UINT32_TO_STREAM(args, ulPriority); 00685 args = UINT32_TO_STREAM(args, ulPairwiseCipher_Or_TxKeyLen); 00686 args = UINT32_TO_STREAM(args, ulGroupCipher_TxKeyIndex); 00687 args = UINT32_TO_STREAM(args, ulKeyMgmt); 00688 args = UINT32_TO_STREAM(args, 0x00000008 + ulSsidLen); 00689 args = UINT32_TO_STREAM(args, ulPassPhraseLen); 00690 ARRAY_TO_STREAM(args, ucSsid, ulSsidLen); 00691 ARRAY_TO_STREAM(args, ucPf_OrKey, ulPassPhraseLen); 00692 00693 arg_len = WLAN_ADD_PROFILE_WPA_PARAM_LEN + ulSsidLen + ulPassPhraseLen; 00694 } 00695 00696 break; 00697 } 00698 00699 // Initiate a HCI command 00700 hci_command_send(HCI_CMND_WLAN_IOCTL_ADD_PROFILE, ptr, arg_len); 00701 00702 // Wait for command complete event 00703 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_ADD_PROFILE, &ret); 00704 00705 return(ret); 00706 } 00707 #else 00708 long 00709 wlan_add_profile(unsigned long ulSecType, 00710 unsigned char* ucSsid, 00711 unsigned long ulSsidLen, 00712 unsigned char *ucBssid, 00713 unsigned long ulPriority, 00714 unsigned long ulPairwiseCipher_Or_TxKeyLen, 00715 unsigned long ulGroupCipher_TxKeyIndex, 00716 unsigned long ulKeyMgmt, 00717 unsigned char* ucPf_OrKey, 00718 unsigned long ulPassPhraseLen) 00719 { 00720 return -1; 00721 } 00722 #endif 00723 00724 //***************************************************************************** 00725 // 00726 //! wlan_ioctl_del_profile 00727 //! 00728 //! @param index number of profile to delete 00729 //! 00730 //! @return On success, zero is returned. On error, -1 is returned 00731 //! 00732 //! @brief Delete WLAN profile 00733 //! 00734 //! @Note In order to delete all stored profile, set index to 255. 00735 //! 00736 //! @sa wlan_add_profile 00737 // 00738 //***************************************************************************** 00739 00740 long 00741 wlan_ioctl_del_profile(unsigned long ulIndex) 00742 { 00743 long ret; 00744 unsigned char *ptr; 00745 unsigned char *args; 00746 00747 ptr = tSLInformation.pucTxCommandBuffer; 00748 args = (unsigned char *)(ptr + HEADERS_SIZE_CMD); 00749 00750 // Fill in HCI packet structure 00751 args = UINT32_TO_STREAM(args, ulIndex); 00752 ret = EFAIL; 00753 00754 // Initiate a HCI command 00755 hci_command_send(HCI_CMND_WLAN_IOCTL_DEL_PROFILE, ptr, WLAN_DEL_PROFILE_PARAMS_LEN); 00756 00757 // Wait for command complete event 00758 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_DEL_PROFILE, &ret); 00759 00760 return(ret); 00761 } 00762 00763 //***************************************************************************** 00764 // 00765 //! wlan_ioctl_get_scan_results 00766 //! 00767 //! @param[in] scan_timeout parameter not supported 00768 //! @param[out] ucResults scan results (_wlan_full_scan_results_args_t) 00769 //! 00770 //! @return On success, zero is returned. On error, -1 is returned 00771 //! 00772 //! @brief Gets entry from scan result table. 00773 //! The scan results are returned one by one, and each entry 00774 //! represents a single AP found in the area. The following is a 00775 //! format of the scan result: 00776 //! - 4 Bytes: number of networks found 00777 //! - 4 Bytes: The status of the scan: 0 - aged results, 00778 //! 1 - results valid, 2 - no results 00779 //! - 42 bytes: Result entry, where the bytes are arranged as follows: 00780 //! 00781 //! - 1 bit isValid - is result valid or not 00782 //! - 7 bits rssi - RSSI value; 00783 //! - 2 bits: securityMode - security mode of the AP: 00784 //! 0 - Open, 1 - WEP, 2 WPA, 3 WPA2 00785 //! - 6 bits: SSID name length 00786 //! - 2 bytes: the time at which the entry has entered into 00787 //! scans result table 00788 //! - 32 bytes: SSID name 00789 //! - 6 bytes: BSSID 00790 //! 00791 //! @Note scan_timeout, is not supported on this version. 00792 //! 00793 //! @sa wlan_ioctl_set_scan_params 00794 // 00795 //***************************************************************************** 00796 00797 #ifndef CC3000_TINY_DRIVER 00798 long 00799 wlan_ioctl_get_scan_results(unsigned long ulScanTimeout, unsigned char *ucResults) 00800 { 00801 unsigned char *ptr; 00802 unsigned char *args; 00803 00804 ptr = tSLInformation.pucTxCommandBuffer; 00805 args = (ptr + HEADERS_SIZE_CMD); 00806 00807 // Fill in temporary command buffer 00808 args = UINT32_TO_STREAM(args, ulScanTimeout); 00809 00810 // Initiate a HCI command 00811 hci_command_send(HCI_CMND_WLAN_IOCTL_GET_SCAN_RESULTS, 00812 ptr, WLAN_GET_SCAN_RESULTS_PARAMS_LEN); 00813 00814 // Wait for command complete event 00815 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_GET_SCAN_RESULTS, (long*)ucResults); 00816 00817 return(0); 00818 } 00819 #endif 00820 00821 //***************************************************************************** 00822 // 00823 //! wlan_ioctl_set_scan_params 00824 //! 00825 //! @param uiEnable - start/stop application scan: 00826 //! 1 = start scan with default interval value of 10 min. 00827 //! in order to set a different scan interval value apply the value 00828 //! in milliseconds. minimum 1 second. 0=stop). Wlan reset 00829 //! (wlan_stop() wlan_start()) is needed when changing scan interval 00830 //! value. Saved: No 00831 //! @param uiMinDwellTime minimum dwell time value to be used for each 00832 //! channel, in milliseconds. Saved: yes 00833 //! Recommended Value: 100 (Default: 20) 00834 //! @param uiMaxDwellTime maximum dwell time value to be used for each 00835 //! channel, in milliseconds. Saved: yes 00836 //! Recommended Value: 100 (Default: 30) 00837 //! @param uiNumOfProbeRequests max probe request between dwell time. 00838 //! Saved: yes. Recommended Value: 5 (Default:2) 00839 //! @param uiChannelMask bitwise, up to 13 channels (0x1fff). 00840 //! Saved: yes. Default: 0x7ff 00841 //! @param uiRSSIThreshold RSSI threshold. Saved: yes (Default: -80) 00842 //! @param uiSNRThreshold NSR threshold. Saved: yes (Default: 0) 00843 //! @param uiDefaultTxPower probe Tx power. Saved: yes (Default: 205) 00844 //! @param aiIntervalList pointer to array with 16 entries (16 channels) 00845 //! each entry (unsigned long) holds timeout between periodic scan 00846 //! (connection scan) - in millisecond. Saved: yes. Default 2000ms. 00847 //! 00848 //! @return On success, zero is returned. On error, -1 is returned 00849 //! 00850 //! @brief start and stop scan procedure. Set scan parameters. 00851 //! 00852 //! @Note uiDefaultTxPower, is not supported on this version. 00853 //! 00854 //! @sa wlan_ioctl_get_scan_results 00855 // 00856 //***************************************************************************** 00857 00858 #ifndef CC3000_TINY_DRIVER 00859 long 00860 wlan_ioctl_set_scan_params(unsigned long uiEnable, unsigned long uiMinDwellTime, 00861 unsigned long uiMaxDwellTime, 00862 unsigned long uiNumOfProbeRequests, 00863 unsigned long uiChannelMask,long iRSSIThreshold, 00864 unsigned long uiSNRThreshold, 00865 unsigned long uiDefaultTxPower, 00866 unsigned long *aiIntervalList) 00867 { 00868 unsigned long uiRes; 00869 unsigned char *ptr; 00870 unsigned char *args; 00871 00872 ptr = tSLInformation.pucTxCommandBuffer; 00873 args = (ptr + HEADERS_SIZE_CMD); 00874 00875 // Fill in temporary command buffer 00876 args = UINT32_TO_STREAM(args, 36); 00877 args = UINT32_TO_STREAM(args, uiEnable); 00878 args = UINT32_TO_STREAM(args, uiMinDwellTime); 00879 args = UINT32_TO_STREAM(args, uiMaxDwellTime); 00880 args = UINT32_TO_STREAM(args, uiNumOfProbeRequests); 00881 args = UINT32_TO_STREAM(args, uiChannelMask); 00882 args = UINT32_TO_STREAM(args, iRSSIThreshold); 00883 args = UINT32_TO_STREAM(args, uiSNRThreshold); 00884 args = UINT32_TO_STREAM(args, uiDefaultTxPower); 00885 ARRAY_TO_STREAM(args, aiIntervalList, sizeof(unsigned long) * SL_SET_SCAN_PARAMS_INTERVAL_LIST_SIZE); 00886 00887 // Initiate a HCI command 00888 hci_command_send(HCI_CMND_WLAN_IOCTL_SET_SCANPARAM, ptr, WLAN_SET_SCAN_PARAMS_LEN); 00889 00890 // Wait for command complete event 00891 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_SET_SCANPARAM, (long*)&uiRes); 00892 00893 return(uiRes); 00894 } 00895 #endif 00896 00897 //***************************************************************************** 00898 // 00899 //! wlan_set_event_mask 00900 //! 00901 //! @param mask mask option: 00902 //! HCI_EVNT_WLAN_UNSOL_CONNECT connect event 00903 //! HCI_EVNT_WLAN_UNSOL_DISCONNECT disconnect event 00904 //! HCI_EVNT_WLAN_ASYNC_SIMPLE_CONFIG_DONE smart config done 00905 //! HCI_EVNT_WLAN_UNSOL_INIT init done 00906 //! HCI_EVNT_WLAN_UNSOL_DHCP dhcp event report 00907 //! HCI_EVNT_WLAN_ASYNC_PING_REPORT ping report 00908 //! HCI_EVNT_WLAN_KEEPALIVE keepalive 00909 //! HCI_EVNT_WLAN_TX_COMPLETE - disable information on end of transmission 00910 //! Saved: no. 00911 //! 00912 //! @return On success, zero is returned. On error, -1 is returned 00913 //! 00914 //! @brief Mask event according to bit mask. In case that event is 00915 //! masked (1), the device will not send the masked event to host. 00916 // 00917 //***************************************************************************** 00918 00919 long 00920 wlan_set_event_mask(unsigned long ulMask) 00921 { 00922 long ret; 00923 unsigned char *ptr; 00924 unsigned char *args; 00925 00926 00927 if ((ulMask & HCI_EVNT_WLAN_TX_COMPLETE) == HCI_EVNT_WLAN_TX_COMPLETE) 00928 { 00929 tSLInformation.InformHostOnTxComplete = 0; 00930 00931 // Since an event is a virtual event - i.e. it is not coming from CC3000 00932 // there is no need to send anything to the device if it was an only event 00933 if (ulMask == HCI_EVNT_WLAN_TX_COMPLETE) 00934 { 00935 return 0; 00936 } 00937 00938 ulMask &= ~HCI_EVNT_WLAN_TX_COMPLETE; 00939 ulMask |= HCI_EVNT_WLAN_UNSOL_BASE; 00940 } 00941 else 00942 { 00943 tSLInformation.InformHostOnTxComplete = 1; 00944 } 00945 00946 ret = EFAIL; 00947 ptr = tSLInformation.pucTxCommandBuffer; 00948 args = (unsigned char *)(ptr + HEADERS_SIZE_CMD); 00949 00950 // Fill in HCI packet structure 00951 args = UINT32_TO_STREAM(args, ulMask); 00952 00953 // Initiate a HCI command 00954 hci_command_send(HCI_CMND_EVENT_MASK, ptr, WLAN_SET_MASK_PARAMS_LEN); 00955 00956 // Wait for command complete event 00957 SimpleLinkWaitEvent(HCI_CMND_EVENT_MASK, &ret); 00958 00959 return(ret); 00960 } 00961 00962 //***************************************************************************** 00963 // 00964 //! wlan_ioctl_statusget 00965 //! 00966 //! @param none 00967 //! 00968 //! @return WLAN_STATUS_DISCONNECTED, WLAN_STATUS_SCANING, 00969 //! STATUS_CONNECTING or WLAN_STATUS_CONNECTED 00970 //! 00971 //! @brief get wlan status: disconnected, scanning, connecting or connected 00972 // 00973 //***************************************************************************** 00974 00975 #ifndef CC3000_TINY_DRIVER 00976 long 00977 wlan_ioctl_statusget(void) 00978 { 00979 long ret; 00980 unsigned char *ptr; 00981 00982 ret = EFAIL; 00983 ptr = tSLInformation.pucTxCommandBuffer; 00984 00985 hci_command_send(HCI_CMND_WLAN_IOCTL_STATUSGET, ptr, 0); 00986 00987 // Wait for command complete event 00988 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_STATUSGET, &ret); 00989 00990 return(ret); 00991 } 00992 #endif 00993 00994 //***************************************************************************** 00995 // 00996 //! wlan_smart_config_start 00997 //! 00998 //! @param algoEncryptedFlag indicates whether the information is encrypted 00999 //! 01000 //! @return On success, zero is returned. On error, -1 is returned 01001 //! 01002 //! @brief Start to acquire device profile. The device acquire its own 01003 //! profile, if profile message is found. The acquired AP information 01004 //! is stored in CC3000 EEPROM only in case AES128 encryption is used. 01005 //! In case AES128 encryption is not used, a profile is created by 01006 //! CC3000 internally. 01007 //! 01008 //! @Note An asynchronous event - Smart Config Done will be generated as soon 01009 //! as the process finishes successfully. 01010 //! 01011 //! @sa wlan_smart_config_set_prefix , wlan_smart_config_stop 01012 // 01013 //***************************************************************************** 01014 01015 long 01016 wlan_smart_config_start(unsigned long algoEncryptedFlag) 01017 { 01018 long ret; 01019 unsigned char *ptr; 01020 unsigned char *args; 01021 01022 ret = EFAIL; 01023 ptr = tSLInformation.pucTxCommandBuffer; 01024 args = (unsigned char *)(ptr + HEADERS_SIZE_CMD); 01025 01026 // Fill in HCI packet structure 01027 args = UINT32_TO_STREAM(args, algoEncryptedFlag); 01028 ret = EFAIL; 01029 01030 hci_command_send(HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_START, ptr, WLAN_SMART_CONFIG_START_PARAMS_LEN); 01031 01032 // Wait for command complete event 01033 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_START, &ret); 01034 01035 return(ret); 01036 } 01037 01038 //***************************************************************************** 01039 // 01040 //! wlan_smart_config_stop 01041 //! 01042 //! @param algoEncryptedFlag indicates whether the information is encrypted 01043 //! 01044 //! @return On success, zero is returned. On error, -1 is returned 01045 //! 01046 //! @brief Stop the acquire profile procedure 01047 //! 01048 //! @sa wlan_smart_config_start , wlan_smart_config_set_prefix 01049 // 01050 //***************************************************************************** 01051 01052 long 01053 wlan_smart_config_stop(void) 01054 { 01055 long ret; 01056 unsigned char *ptr; 01057 01058 ret = EFAIL; 01059 ptr = tSLInformation.pucTxCommandBuffer; 01060 01061 hci_command_send(HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_STOP, ptr, 0); 01062 01063 // Wait for command complete event 01064 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_STOP, &ret); 01065 01066 return(ret); 01067 } 01068 01069 //***************************************************************************** 01070 // 01071 //! wlan_smart_config_set_prefix 01072 //! 01073 //! @param newPrefix 3 bytes identify the SSID prefix for the Smart Config. 01074 //! 01075 //! @return On success, zero is returned. On error, -1 is returned 01076 //! 01077 //! @brief Configure station ssid prefix. The prefix is used internally 01078 //! in CC3000. It should always be TTT. 01079 //! 01080 //! @Note The prefix is stored in CC3000 NVMEM 01081 //! 01082 //! @sa wlan_smart_config_start , wlan_smart_config_stop 01083 // 01084 //***************************************************************************** 01085 01086 long 01087 wlan_smart_config_set_prefix(char* cNewPrefix) 01088 { 01089 long ret; 01090 unsigned char *ptr; 01091 unsigned char *args; 01092 01093 ret = EFAIL; 01094 ptr = tSLInformation.pucTxCommandBuffer; 01095 args = (ptr + HEADERS_SIZE_CMD); 01096 01097 if (cNewPrefix == NULL) 01098 return ret; 01099 else // with the new Smart Config, prefix must be TTT 01100 { 01101 *cNewPrefix = 'T'; 01102 *(cNewPrefix + 1) = 'T'; 01103 *(cNewPrefix + 2) = 'T'; 01104 } 01105 01106 ARRAY_TO_STREAM(args, cNewPrefix, SL_SIMPLE_CONFIG_PREFIX_LENGTH); 01107 01108 hci_command_send(HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_SET_PREFIX, ptr, SL_SIMPLE_CONFIG_PREFIX_LENGTH); 01109 01110 // Wait for command complete event 01111 SimpleLinkWaitEvent(HCI_CMND_WLAN_IOCTL_SIMPLE_CONFIG_SET_PREFIX, &ret); 01112 01113 return(ret); 01114 } 01115 01116 //***************************************************************************** 01117 // 01118 //! wlan_smart_config_process 01119 //! 01120 //! @param none 01121 //! 01122 //! @return On success, zero is returned. On error, -1 is returned 01123 //! 01124 //! @brief process the acquired data and store it as a profile. The acquired 01125 //! AP information is stored in CC3000 EEPROM encrypted. 01126 //! The encrypted data is decrypted and stored as a profile. 01127 //! behavior is as defined by connection policy. 01128 // 01129 //***************************************************************************** 01130 01131 01132 #ifndef CC3000_UNENCRYPTED_SMART_CONFIG 01133 long 01134 wlan_smart_config_process() 01135 { 01136 signed long returnValue; 01137 unsigned long ssidLen, keyLen; 01138 unsigned char *decKeyPtr; 01139 unsigned char *ssidPtr; 01140 01141 // read the key from EEPROM - fileID 12 01142 returnValue = aes_read_key(key); 01143 01144 if (returnValue != 0) 01145 return returnValue; 01146 01147 // read the received data from fileID #13 and parse it according to the followings: 01148 // 1) SSID LEN - not encrypted 01149 // 2) SSID - not encrypted 01150 // 3) KEY LEN - not encrypted. always 32 bytes long 01151 // 4) Security type - not encrypted 01152 // 5) KEY - encrypted together with true key length as the first byte in KEY 01153 // to elaborate, there are two corner cases: 01154 // 1) the KEY is 32 bytes long. In this case, the first byte does not represent KEY length 01155 // 2) the KEY is 31 bytes long. In this case, the first byte represent KEY length and equals 31 01156 returnValue = nvmem_read(NVMEM_SHARED_MEM_FILEID, SMART_CONFIG_PROFILE_SIZE, 0, profileArray); 01157 01158 if (returnValue != 0) 01159 return returnValue; 01160 01161 ssidPtr = &profileArray[1]; 01162 01163 ssidLen = profileArray[0]; 01164 01165 decKeyPtr = &profileArray[profileArray[0] + 3]; 01166 01167 aes_decrypt(decKeyPtr, key); 01168 if (profileArray[profileArray[0] + 1] > 16) 01169 aes_decrypt((unsigned char *)(decKeyPtr + 16), key); 01170 01171 if (*(unsigned char *)(decKeyPtr +31) != 0) 01172 { 01173 if (*decKeyPtr == 31) 01174 { 01175 keyLen = 31; 01176 decKeyPtr++; 01177 } 01178 else 01179 { 01180 keyLen = 32; 01181 } 01182 } 01183 else 01184 { 01185 keyLen = *decKeyPtr; 01186 decKeyPtr++; 01187 } 01188 01189 // add a profile 01190 switch (profileArray[profileArray[0] + 2]) 01191 { 01192 case WLAN_SEC_UNSEC://None 01193 { 01194 returnValue = wlan_add_profile(profileArray[profileArray[0] + 2], // security type 01195 ssidPtr, // SSID 01196 ssidLen, // SSID length 01197 NULL, // BSSID 01198 1, // Priority 01199 0, 0, 0, 0, 0); 01200 01201 break; 01202 } 01203 01204 case WLAN_SEC_WEP://WEP 01205 { 01206 returnValue = wlan_add_profile(profileArray[profileArray[0] + 2], // security type 01207 ssidPtr, // SSID 01208 ssidLen, // SSID length 01209 NULL, // BSSID 01210 1, // Priority 01211 keyLen, // KEY length 01212 0, // KEY index 01213 0, 01214 decKeyPtr, // KEY 01215 0); 01216 01217 break; 01218 } 01219 01220 case WLAN_SEC_WPA://WPA 01221 case WLAN_SEC_WPA2://WPA2 01222 { 01223 returnValue = wlan_add_profile(WLAN_SEC_WPA2, // security type 01224 ssidPtr, 01225 ssidLen, 01226 NULL, // BSSID 01227 1, // Priority 01228 0x18, // PairwiseCipher 01229 0x1e, // GroupCipher 01230 2, // KEY management 01231 decKeyPtr, // KEY 01232 keyLen); // KEY length 01233 01234 break; 01235 } 01236 } 01237 01238 return returnValue; 01239 } 01240 #endif //CC3000_UNENCRYPTED_SMART_CONFIG 01241 01242 //***************************************************************************** 01243 // 01244 // Close the Doxygen group. 01245 //! @} 01246 // 01247 //***************************************************************************** 01248
Generated on Tue Jul 12 2022 19:26:44 by
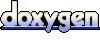