
ADC Niose test Connect four analog signals to your MBED. and then run the Windows app. The four traces are displayed on an oscilloscope like display. I have used a USB HID DEVICE link, so connections to D+, D- are required. The MBED code is otherwise quite basic, So you can modify it to your own test needs. Additionaly, there is a 16 bit count value, in my MBED code Mainly to test if MSB & LSB are correct.
USBBusInterface.h
00001 /* USBBusInterface.h */ 00002 /* USB Bus Interface */ 00003 /* Copyright (c) 2011 ARM Limited. All rights reserved. */ 00004 00005 #ifndef USBBUSINTERFACE_H 00006 #define USBBUSINTERFACE_H 00007 00008 #include "mbed.h" 00009 #include "USBEndpoints.h" 00010 00011 class USBHAL { 00012 public: 00013 /* Configuration */ 00014 USBHAL(); 00015 ~USBHAL(); 00016 void connect(void); 00017 void disconnect(void); 00018 void configureDevice(void); 00019 void unconfigureDevice(void); 00020 void setAddress(uint8_t address); 00021 void remoteWakeup(void); 00022 00023 /* Endpoint 0 */ 00024 void EP0setup(uint8_t *buffer); 00025 void EP0read(void); 00026 uint32_t EP0getReadResult(uint8_t *buffer); 00027 void EP0write(uint8_t *buffer, uint32_t size); 00028 void EP0getWriteResult(void); 00029 void EP0stall(void); 00030 00031 /* Other endpoints */ 00032 EP_STATUS endpointRead(uint8_t endpoint, uint32_t maximumSize); 00033 EP_STATUS endpointReadResult(uint8_t endpoint, uint8_t *data, uint32_t *bytesRead); 00034 EP_STATUS endpointWrite(uint8_t endpoint, uint8_t *data, uint32_t size); 00035 EP_STATUS endpointWriteResult(uint8_t endpoint); 00036 void stallEndpoint(uint8_t endpoint); 00037 void unstallEndpoint(uint8_t endpoint); 00038 bool realiseEndpoint(uint8_t endpoint, uint32_t maxPacket, uint32_t options); 00039 bool getEndpointStallState(unsigned char endpoint); 00040 00041 protected: 00042 virtual void busReset(void){}; 00043 virtual void EP0setupCallback(void){}; 00044 virtual void EP0out(void){}; 00045 virtual void EP0in(void){}; 00046 virtual void connectStateChanged(unsigned int connected){}; 00047 virtual void suspendStateChanged(unsigned int suspended){}; 00048 void SOF(int frameNumber){}; 00049 virtual bool EPBULK_OUT_callback(){return false;}; 00050 virtual bool EPBULK_IN_callback(){return false;}; 00051 00052 private: 00053 void usbisr(void); 00054 static void _usbisr(void); 00055 static USBHAL * instance; 00056 }; 00057 #endif 00058 00059
Generated on Tue Jul 12 2022 20:27:20 by
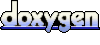