Program to record speech audio into RAM and then play it back, moving Billy Bass's mouth in sync with the speech.
AudioAnalyzer.cpp
00001 #include "mbed.h" 00002 #include "AudioAnalyzer.h" 00003 00004 namespace NK 00005 { 00006 00007 void AudioAnalyzer::analyze() 00008 { 00009 if (analyzed) return; 00010 00011 // compute the sum of the squares of the input samples 00012 power = 0; 00013 zeroCrossings = 0; 00014 minValue = 127; 00015 maxValue = -128; 00016 int8_t const * const lastSample = samples + nsamples - 1; 00017 bool sign, lastSign = samples[0] < 0; 00018 for (int8_t const *p = samples; p <= lastSample; p++) { 00019 int8_t val = *p++; 00020 power += ((int16_t)val * val); 00021 sign = (val < 0); 00022 if (sign != lastSign) 00023 zeroCrossings++; 00024 lastSign = sign; 00025 if (val > maxValue) 00026 maxValue = val; 00027 if (val < minValue) 00028 minValue = val; 00029 } 00030 // normalize power 00031 power /= nsamples; 00032 analyzed = true; 00033 } 00034 00035 }
Generated on Wed Jul 13 2022 10:13:19 by
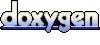