Library allowing up to 16 strings of 60 WS2811 or WS2812 LEDs to be driven from a single FRDM-KL25Z board. Uses hardware DMA to do a full 800 KHz rate without much CPU burden.
Colors.cpp
00001 #include <math.h> 00002 #include "Colors.h" 00003 00004 void HSBtoRGB(float hue, float saturation, float brightness, uint8_t *pr, uint8_t *pg, uint8_t *pb) 00005 { 00006 uint8_t r = 0, g = 0, b = 0; 00007 if (saturation == 0) { 00008 r = g = b = (uint8_t) (brightness * 255.0f + 0.5f); 00009 } else { 00010 float h = (hue - (float)floor(hue)) * 6.0f; 00011 float f = h - (float)floor(h); 00012 float p = brightness * (1.0f - saturation); 00013 float q = brightness * (1.0f - saturation * f); 00014 float t = brightness * (1.0f - (saturation * (1.0f - f))); 00015 switch ((int) h) { 00016 case 0: 00017 r = (int) (brightness * 255.0f + 0.5f); 00018 g = (int) (t * 255.0f + 0.5f); 00019 b = (int) (p * 255.0f + 0.5f); 00020 break; 00021 case 1: 00022 r = (int) (q * 255.0f + 0.5f); 00023 g = (int) (brightness * 255.0f + 0.5f); 00024 b = (int) (p * 255.0f + 0.5f); 00025 break; 00026 case 2: 00027 r = (int) (p * 255.0f + 0.5f); 00028 g = (int) (brightness * 255.0f + 0.5f); 00029 b = (int) (t * 255.0f + 0.5f); 00030 break; 00031 case 3: 00032 r = (int) (p * 255.0f + 0.5f); 00033 g = (int) (q * 255.0f + 0.5f); 00034 b = (int) (brightness * 255.0f + 0.5f); 00035 break; 00036 case 4: 00037 r = (int) (t * 255.0f + 0.5f); 00038 g = (int) (p * 255.0f + 0.5f); 00039 b = (int) (brightness * 255.0f + 0.5f); 00040 break; 00041 case 5: 00042 r = (int) (brightness * 255.0f + 0.5f); 00043 g = (int) (p * 255.0f + 0.5f); 00044 b = (int) (q * 255.0f + 0.5f); 00045 break; 00046 } 00047 } 00048 *pr = r; 00049 *pg = g; 00050 *pb = b; 00051 } 00052 00053 float* RGBtoHSB(uint8_t r, uint8_t g, uint8_t b, float* hsbvals) 00054 { 00055 float hue, saturation, brightness; 00056 if (!hsbvals) { 00057 hsbvals = new float[3]; 00058 } 00059 uint8_t cmax = (r > g) ? r : g; 00060 if (b > cmax) cmax = b; 00061 uint8_t cmin = (r < g) ? r : g; 00062 if (b < cmin) cmin = b; 00063 00064 brightness = ((float) cmax) / 255.0f; 00065 if (cmax != 0) 00066 saturation = ((float) (cmax - cmin)) / ((float) cmax); 00067 else 00068 saturation = 0; 00069 if (saturation == 0) 00070 hue = 0; 00071 else { 00072 float redc = ((float) (cmax - r)) / ((float) (cmax - cmin)); 00073 float greenc = ((float) (cmax - g)) / ((float) (cmax - cmin)); 00074 float bluec = ((float) (cmax - b)) / ((float) (cmax - cmin)); 00075 if (r == cmax) 00076 hue = bluec - greenc; 00077 else if (g == cmax) 00078 hue = 2.0f + redc - bluec; 00079 else 00080 hue = 4.0f + greenc - redc; 00081 hue = hue / 6.0f; 00082 if (hue < 0) 00083 hue = hue + 1.0f; 00084 } 00085 hsbvals[0] = hue; 00086 hsbvals[1] = saturation; 00087 hsbvals[2] = brightness; 00088 return hsbvals; 00089 }
Generated on Wed Jul 13 2022 13:18:58 by
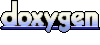