This library provides a class to generate differents signale wave form. Note that the maximum update rate of 1 MHz, so Fmax = 1MHz / _num_pixels (see UM10360 - Chapter 30: LPC17xx Digital-to-Analog Converter (DAC).
SignalGenerator.h
00001 /* mbed signal generator implementation using LPC1768 DAC 00002 * Copyright (c) 2010 ygarcia 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #if !defined(__SIGNALGERNERATOR_H__) 00024 #define __SIGNALGERNERATOR_H__ 00025 00026 #include "Debug.h" 00027 00028 /** This class provides methods to generate differents signale wave form. V0.0.0.2 00029 * 00030 * Note that the maximum update rate of 1 MHz, so Fmax = 1MHz / _num_pixels 00031 * 00032 * Note that Vout is given by the relation below (UM10360 - Table 537. D/A Pin Description): 00033 * Vout = VALUE * ((Vrefp - Vrefn) / 1024) + Vrefn 00034 * in which: 00035 * - Vrefp: tied to VDD (e.g. 3.3V) 00036 * - Vrefn: tied to Vss 00037 * @see UM10360 - Chapter 30: LPC17xx Digital-to-Analog Converter (DAC) 00038 * @remark This class was validated with Tektronix TDS2014 oscilloscope 00039 * @author Yann Garcia (Don't hesitate to contact me: garcia.yann@gmail.com) 00040 * References: 00041 * - http://fabrice.sincere.pagesperso-orange.fr/cm_electronique/projet_pic/gbf/gbf.htm 00042 * @todo 1) Enhance comments 2) Add support of user-defined signal in byte array format 00043 */ 00044 class SignalGenerator 00045 { 00046 public: 00047 /** List of supported signals 00048 */ 00049 enum SignalGeneratorType { 00050 SquareSignal = 0, //<! Square signal (50% duty cycle) 00051 TriangleSignal = 1, //<! Triangle signal 00052 SawtoothSignal = 2, //<! Sawtooth signal 00053 ReverseSawtoothSignal = 3, //<! Reversed sawtooth signal 00054 SinusSignal = 4 //<! Sinusoidal signal 00055 }; // End of SignalGeneratorType enum 00056 public: 00057 /** Default ctor 00058 * 00059 * Initialize the internal datas. Default values are: 00060 * - signal type: SquareSignal 00061 * - frequency: 1000Hz 00062 * - # of pixels is fixed, _twait is adjusted 00063 * 00064 * @param p_outPort: Pin name of the Analog out port (e.g. p18 for LPC1768) 00065 */ 00066 SignalGenerator(PinName p_outPort); 00067 /** Default dtor 00068 * 00069 * Free resources 00070 */ 00071 virtual ~SignalGenerator(); 00072 00073 /** Set the frequency of the signal. 00074 * 00075 * @param p_signalType: Signal type. Default value is square signal 00076 * @param p_frequency: Signal frequency. Default value is 1000Hz 00077 * @param p_num_pixels: Number of pixels ber period. Default value is 64 00078 * @see UM10360 - Chapter 30: LPC17xx Digital-to-Analog Converter (DAC) 00079 * @code 00080 * // Prepare a sinus signal at 500Hz 00081 * signal.SetSignalFrequency(SignalGenerator::SinusSignal,500); 00082 * // Launch execution 00083 * signal.BeginRunAsync(); 00084 * // Here signal generation is running. Use Led or oscilloscope to vizualize output 00085 * printf("Press any key to terminate"); 00086 * getchar(); 00087 * // Terminate execution 00088 * signal.EndRunAsync(); 00089 * @endcode 00090 */ 00091 void SetSignalFrequency(SignalGenerator::SignalGeneratorType p_signalType = SignalGenerator::SquareSignal, int p_frequency = 1000, int p_num_pixels = 64); 00092 /** Generate the signal in synchrnous mode 00093 * 00094 * @see Stop() method 00095 */ 00096 void Run(); 00097 /** Method called to terminate synchronous running mode 00098 */ 00099 void Stop(); 00100 /** Method called to prepare an asynchronous running mode 00101 * 00102 * @return 0 on success, -1 if _twait less that 7us. On error, you shall use synchronous method 00103 */ 00104 int BeginRunAsync(); 00105 /** Method called to launch the asynchronous running mode 00106 */ 00107 void RunAsync(); 00108 /** Method called to terminate asynchronous running mode 00109 */ 00110 void EndRunAsync(); 00111 00112 SignalGenerator& operator =(const bool& p_mode); 00113 SignalGenerator& operator =(const int& p_frequency); 00114 SignalGenerator& operator =(const SignalGenerator::SignalGeneratorType& p_signalType); 00115 private: 00116 /** Set the signal type to generate and build the lookup table according to the signal frequency. 00117 */ 00118 void PrepareSignal(); 00119 /** Build the lookup table for a square signal according to the signal frequency. 00120 * 00121 * Note that the duty cycle is 50%. For different values, please refer to PwmOut class 00122 */ 00123 void PrepareSquareSignal(); 00124 /** Build the lookup table for a triangle signal according to the signal frequency. 00125 */ 00126 void PrepareTriangleSignal(); 00127 /** Build the lookup table for a reverse sawtooth signal according to the signal frequency. 00128 */ 00129 void PrepareSawtoothSignal(); 00130 /** Build the lookup table for a sawtooth sinal according to the signal frequency. 00131 */ 00132 void PrepareReverseSawtoothSignal(); 00133 /** Build the lookup table for a 'sinus' sinal according to the signal frequency. 00134 */ 00135 void PrepareSinusSignal(); 00136 private: 00137 SignalGenerator::SignalGeneratorType _signalType; //<! Signal selected - Default value is SquareSignal 00138 int _frequency; // Required frequency of the signal 00139 float *_values; //<! Pointer to the array of calculated values for the selected signal 00140 int _num_pixels; // <! Number of calculated points, this is the _values length 00141 float _twait; //<! Time between each changes of the analog output 00142 bool _mode; //<! # of pixels is fixed (128 pixels) 00143 AnalogOut _out; //<! Analog out pin of the LPC1768 00144 bool _stop; //<! Set to tru to stop the signal generation 00145 int _asynckCounter; //<! Index on _values when asynchronous run is used 00146 Ticker _asyncTicker; //<! Ticker to ipdate DAC when asynchronous run is used 00147 DigitalOut _debugLed; //<! For debug with oscilloscope purpose 00148 }; // End of class SignalGenerator 00149 00150 #endif // __SIGNALGERNERATOR_H__
Generated on Tue Jul 19 2022 21:58:57 by
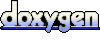