SCA61T Single Axis Inclinometer
Embed:
(wiki syntax)
Show/hide line numbers
SCA61T.h
00001 /* mbed SCA61T Single Axis Inclinometer 00002 * Copyright (c) 2010 Veikko Soininen 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef _SCA61T_H_ 00024 #define _SCA61T_H_ 00025 00026 #include "mbed.h" 00027 00028 /** SCA61T Inclinometer class 00029 * 00030 * Example: 00031 * @code 00032 * // Send temperature and angle to serial port every one second. 00033 * #include "mbed.h" 00034 * #include "SCA61T.h" 00035 * 00036 * SCA61T sca61t(p11,p12,p13,p21,0); // MOSI, MISO, SCLK, CSB, device selection 00037 * // 0=SCA61T-FAHH1G, 1=SCA61T-FA1H1G 00038 * Serial pc(USBTX, USBRX); 00039 * 00040 * int main(void) 00041 * { 00042 * while(1) 00043 * { 00044 * pc.printf("%i [C]\r\n",sca61t.ReadTemp()); // Writes the temperature to serial port. 00045 * pc.printf("%.1f [deg]\r\n",sca61t.ReadX()); // Writes the angle to serial port. 00046 * wait(1); // Waits for one second and repeats. 00047 * } 00048 * } 00049 * @endcode 00050 */ 00051 00052 class SCA61T { 00053 public: 00054 SCA61T(PinName mosi, PinName miso, PinName sclk, PinName csb, int device_sel); 00055 ~SCA61T() {}; 00056 00057 /** Reads the angle 00058 * 00059 * @returns angle in degrees 00060 */ 00061 float ReadX(); 00062 00063 /** Reads the temperature 00064 * 00065 * @returns temperature in Celcius 00066 */ 00067 int8_t ReadTemp(); 00068 00069 /** Sets the sensor to measurement mode 00070 * 00071 * Default mode, not needed to call 00072 * first 00073 */ 00074 void MeasMode(); 00075 00076 /** Reads the status register 00077 * 00078 * @returns value of status register 00079 */ 00080 uint8_t ReadStatus(); 00081 00082 /** Reloads NV. 00083 * 00084 */ 00085 void ReloadNV(); 00086 00087 /** Sets the sensor to self test mode 00088 * 00089 * Discharges the sensor element. De-activated 00090 * by MeasMode(). 00091 */ 00092 void SelfTest(); 00093 00094 private: 00095 SPI SPI_m; 00096 DigitalOut CSB_m; 00097 00098 void SPI_SendByte(uint8_t byte); 00099 uint8_t SPI_ReadReg(uint8_t reg); 00100 void SPI_ReadWord(uint8_t cmd, char* table); 00101 void SPI_Command(uint8_t cmd); 00102 }; 00103 00104 #endif
Generated on Tue Jul 12 2022 14:12:05 by
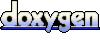