SCA61T Single Axis Inclinometer
Embed:
(wiki syntax)
Show/hide line numbers
SCA61T.cpp
00001 /* mbed SCA61T Single Axis Inclinometer 00002 * Copyright (c) 2010 Veikko Soininen 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #include "SCA61T.h" 00024 00025 // ** Class 00026 00027 static int sel; 00028 00029 SCA61T::SCA61T(PinName mosi, PinName miso, PinName sclk, PinName csb, int device_sel) 00030 : SPI_m(mosi, miso, sclk) 00031 , CSB_m(csb) { 00032 sel=device_sel; 00033 CSB_m=1; 00034 SPI_m.frequency(500000); 00035 } 00036 00037 // ** SPI 00038 00039 uint8_t SCA61T::SPI_ReadReg(uint8_t reg) 00040 { 00041 uint8_t reply; 00042 00043 CSB_m=0; 00044 SPI_m.write(reg); 00045 reply = SPI_m.write(0x00); 00046 CSB_m=1; 00047 00048 return reply; 00049 } 00050 00051 void SCA61T::SPI_ReadWord(uint8_t cmd, char* table) 00052 { 00053 CSB_m=0; 00054 SPI_m.write(cmd); 00055 for(int i=0;i<2;i++) 00056 *table++ = SPI_m.write(0x00); 00057 CSB_m=1; 00058 } 00059 00060 void SCA61T::SPI_Command(uint8_t cmd) 00061 { 00062 CSB_m=0; 00063 SPI_m.write(cmd); 00064 CSB_m=1; 00065 } 00066 00067 // ** SCA61T 00068 00069 float SCA61T::ReadX() 00070 { 00071 uint8_t temp[2]; 00072 uint16_t data; 00073 float device_sens; 00074 float angle; 00075 00076 if(sel) 00077 device_sens = 819.00; 00078 else 00079 device_sens = 1638.00; 00080 00081 SPI_ReadWord(0x10,(char*)temp); 00082 00083 temp[1] = (temp[1]>>5); 00084 data=(temp[0]<<3)+temp[1]; 00085 00086 angle=asinf((data-1024.00f)/device_sens)*(180.00f/3.14f); 00087 00088 return angle; 00089 } 00090 00091 int8_t SCA61T::ReadTemp() 00092 { 00093 int temp; 00094 00095 temp=SPI_ReadReg(0x08); 00096 temp=rint((temp-197.000)/(-1.083)); 00097 00098 return temp; 00099 } 00100 00101 void SCA61T::MeasMode() 00102 { 00103 SPI_Command(0x00); 00104 } 00105 00106 uint8_t SCA61T::ReadStatus() 00107 { 00108 return SPI_ReadReg(0x0A); 00109 } 00110 00111 void SCA61T::ReloadNV() 00112 { 00113 SPI_Command(0x0B); 00114 } 00115 00116 void SCA61T::SelfTest() 00117 { 00118 SPI_Command(0x0E); 00119 }
Generated on Tue Jul 12 2022 14:12:05 by
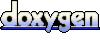