
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // AD-12864-SPI test program 00002 // About AD-12864-SPI, see http://www.aitendo.co.jp/product/1622. 00003 00004 // Pin allocation 00005 // 1 p21 #CS1 with 10k ohm pull-up 00006 // 2 p22 #RESET with 10k ohm pull-up 00007 // 3 p23 A0 ... 0:command 1:data 00008 // 4 p13 SCK 00009 // 5 p11 MOSI 00010 // 6 Vdd 00011 // 7 Vss 00012 // 8 NC LED_A 00013 00014 #include "mbed.h" 00015 #include "font8.h" 00016 00017 DigitalOut cs(p21); 00018 DigitalOut rst(p22); 00019 DigitalOut a0(p23); 00020 SPI spi(p11, p12, p13); // mosi, miso, sclk 00021 00022 void regwrite(unsigned char c){ 00023 cs = a0 = 0; 00024 spi.write(c); 00025 cs = 1; 00026 } 00027 00028 void datawrite(unsigned char c){ 00029 cs = 0; 00030 a0 = 1; 00031 spi.write(c); 00032 cs = 1; 00033 } 00034 00035 // set position (x, 8*y) 00036 void locate(int x, int y){ 00037 regwrite(0xb0 | (y & 0x0f)); // Page Address Set (see 2.4.3) 00038 regwrite(0x10 | (x >> 4 & 0x0f)); // Column Address Set (see 2.4.4) 00039 regwrite(x & 0x0f); 00040 } 00041 00042 void cls(void){ 00043 int x, y; 00044 for(y = 0; y < 8; y++){ 00045 locate(0, y); 00046 for(x = 0; x < 128; x++) datawrite(0x00); 00047 } 00048 } 00049 00050 void plot(int x, int y){ 00051 locate(x, y >> 3); 00052 datawrite(1 << (y & 7)); 00053 } 00054 00055 void init(){ 00056 spi.format(8,0); // nazo 00057 spi.frequency(10000000); // modify later 00058 00059 // reset 00060 wait_ms(200); 00061 rst = 0; 00062 wait_ms(200); 00063 rst = 1; 00064 00065 // initialize sequence 00066 regwrite(0xaf); // display on (see 2.4.1) 00067 regwrite(0x2f); // power control set (see 2.4.16) 00068 regwrite(0x81); // set electronic volume mode (see 2.4.18) 00069 // regwrite(0x1f); // electronic volume data 00-3f 00070 regwrite(0x00); // electronic volume data 00-3f 00071 regwrite(0x27); // V5 Volatge Regulator Internal Resister Ratio Set (see 2.4.17) 00072 regwrite(0xa2); // LCD Bias Set ... 1/9 bias (see 2.4.11) 00073 regwrite(0xc8); // Common Output Mode Select ... Reverse (see 2.4.15) 00074 regwrite(0xa0); // ADC Select ... Normal (see 2.4.8) 00075 regwrite(0xa4); // Display All Points ON/OFF ... normal (see 2.4.10) 00076 regwrite(0xa6); // Display Normal/Reverse ... normal (see 2.4.9) 00077 regwrite(0xac); // Static Indicator ... off (see 2.4.19) 00078 regwrite(0x00); // off 00079 regwrite(0x40); // Display Strat Line Set ... 0 (see 2.4.2) 00080 regwrite(0xe0); // Write Mode Set 00081 } 00082 00083 void drawchar(unsigned char c){ 00084 const unsigned char *p = &font8[c << 3]; 00085 datawrite(p[0]); 00086 datawrite(p[1]); 00087 datawrite(p[2]); 00088 datawrite(p[3]); 00089 datawrite(p[4]); 00090 datawrite(p[5]); 00091 datawrite(p[6]); 00092 datawrite(p[7]); 00093 } 00094 00095 void drawtext(const char *s){ 00096 unsigned char c; 00097 while((c = *s++) != '\0') drawchar(c); 00098 } 00099 00100 int main() { 00101 int x, y, c; 00102 char buf[16]; 00103 00104 init(); 00105 cls(); 00106 00107 locate(0, 0); 00108 sprintf(buf, "%08x", 0xdeadbeef); 00109 drawtext(buf); 00110 wait_ms(2000); 00111 00112 c = 0; 00113 while(1) { 00114 for(y = 0; y < 8; y++){ 00115 locate(0, y); 00116 for(x = 0; x < 16; x++) drawchar(c++); 00117 } 00118 wait_ms(800); 00119 } 00120 }
Generated on Tue Jul 12 2022 19:56:32 by
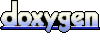