
blablabla
Dependencies: MAG3110 MMA8451Q SLCD- TSI USBDevice mbed
SLCD.h
00001 #pragma once 00002 00003 #include "mbed.h" 00004 00005 00006 /* ------ sample usage------ 00007 00008 #include "mbed.h" 00009 #include "SLCD.h" 00010 00011 SLCD slcd; 00012 00013 main() 00014 { 00015 slcd.printf("1.2.3.4"); // standard printf function, only charaters in ASCII_TO_WF_CODIFICATION_TABLE will display 00016 // Dots printed using decimal points 00017 slcd.putc('A'); // prints a single character 00018 slcd.clear(); // All segments off 00019 slcd.All_Segments(y); // y=1 for ALL segments on, 0 for ALL segments off 00020 slcd.DP(x, true/false); // Set/Clear decimal point. x=0, 1 or 2. 00021 // Does nothing if invalid decimal point 00022 slcd.DPx(y); // x=DP1 to DP3, y=1 for on 0 for off (legacy) 00023 slcd.Colon(y); // y=1 for on, 0 for off 00024 slcd.CharPosition=x; // x=0 to 3, 0 is start position 00025 slcd.Home(); // sets next charater to posistion 0 (start) 00026 slcd.Contrast (x); // set contrast x=0 - 15, 0 lightest, 15 darkest 00027 slcd.blink(x); // set display to blink, 0-7 is blink rate (default = 3), -1 disables blink 00028 slcd.deepsleepEnable(x);// true (default) keeps the lcd enabled in deepsleep, false disables its 4MHz internal oscillator clock. Total power consumption ~= 40uA 00029 } 00030 */ 00031 00032 class SLCD : public Stream 00033 { 00034 public: 00035 SLCD(); 00036 00037 void Home (void); 00038 void Contrast (uint8_t lbContrast); 00039 void All_Segments (int); 00040 void clear(); 00041 void DP(int pos, bool on); 00042 void DP1 (int); 00043 void DP2 (int); 00044 void DP3 (int); 00045 void Colon (int); 00046 uint8_t CharPosition; 00047 void blink(int blink = 3); 00048 void deepsleepEnable(bool enable); 00049 00050 private: 00051 void Write_Char(char lbValue); 00052 void init(); 00053 virtual int _putc(int c); 00054 virtual int _getc() { 00055 return 0; 00056 } 00057 };
Generated on Tue Jul 12 2022 16:20:43 by
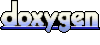