
MP3 Player without external hardware MP3 Player without external hardware. A software based MP3 player based on a modified version of libmad. Mono output (at the moment) via AnalogOut. Files are read from an USB drive. This is a demo program, it plays only one file at the moment. Documentation is in "main.cpp" and "config.h"
stream.cpp
00001 /* 00002 * libmad - MPEG audio decoder library 00003 * Copyright (C) 2000-2004 Underbit Technologies, Inc. 00004 * 00005 * This program is free software; you can redistribute it and/or modify 00006 * it under the terms of the GNU General Public License as published by 00007 * the Free Software Foundation; either version 2 of the License, or 00008 * (at your option) any later version. 00009 * 00010 * This program is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00013 * GNU General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU General Public License 00016 * along with this program; if not, write to the Free Software 00017 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00018 * 00019 * $Id: stream.c,v 1.1 2010/11/23 20:12:57 andy Exp $ 00020 */ 00021 00022 # include "config.h" 00023 00024 # include "global.h" 00025 00026 # include <stdlib.h> 00027 00028 # include "bit.h" 00029 # include "stream.h" 00030 00031 /* 00032 * NAME: stream->init() 00033 * DESCRIPTION: initialize stream struct 00034 */ 00035 void mad_stream_init(struct mad_stream *stream) 00036 { 00037 stream->buffer = 0; 00038 stream->bufend = 0; 00039 stream->skiplen = 0; 00040 00041 stream->sync = 0; 00042 stream->freerate = 0; 00043 00044 stream->this_frame = 0; 00045 stream->next_frame = 0; 00046 mad_bit_init(&stream->ptr, 0); 00047 00048 mad_bit_init(&stream->anc_ptr, 0); 00049 stream->anc_bitlen = 0; 00050 00051 stream->main_data = 0; 00052 stream->md_len = 0; 00053 00054 stream->options = 0; 00055 stream->error = MAD_ERROR_NONE; 00056 } 00057 00058 /* 00059 * NAME: stream->finish() 00060 * DESCRIPTION: deallocate any dynamic memory associated with stream 00061 */ 00062 void mad_stream_finish(struct mad_stream *stream) 00063 { 00064 if (stream->main_data) { 00065 #if !defined(TARGET_LPC1768) 00066 free(stream->main_data); 00067 #endif 00068 stream->main_data = 0; 00069 } 00070 00071 mad_bit_finish(&stream->anc_ptr); 00072 mad_bit_finish(&stream->ptr); 00073 } 00074 00075 /* 00076 * NAME: stream->buffer() 00077 * DESCRIPTION: set stream buffer pointers 00078 */ 00079 void mad_stream_buffer(struct mad_stream *stream, 00080 unsigned char const *buffer, unsigned long length) 00081 { 00082 stream->buffer = buffer; 00083 stream->bufend = buffer + length; 00084 00085 stream->this_frame = buffer; 00086 stream->next_frame = buffer; 00087 00088 stream->sync = 1; 00089 00090 mad_bit_init(&stream->ptr, buffer); 00091 } 00092 00093 /* 00094 * NAME: stream->skip() 00095 * DESCRIPTION: arrange to skip bytes before the next frame 00096 */ 00097 void mad_stream_skip(struct mad_stream *stream, unsigned long length) 00098 { 00099 stream->skiplen += length; 00100 } 00101 00102 /* 00103 * NAME: stream->sync() 00104 * DESCRIPTION: locate the next stream sync word 00105 */ 00106 int mad_stream_sync(struct mad_stream *stream) 00107 { 00108 register unsigned char const *ptr, *end; 00109 00110 ptr = mad_bit_nextbyte(&stream->ptr); 00111 end = stream->bufend; 00112 00113 while (ptr < end - 1 && 00114 !(ptr[0] == 0xff && (ptr[1] & 0xe0) == 0xe0)) 00115 ++ptr; 00116 00117 if (end - ptr < MAD_BUFFER_GUARD) 00118 return -1; 00119 00120 mad_bit_init(&stream->ptr, ptr); 00121 00122 return 0; 00123 } 00124 00125 /* 00126 * NAME: stream->errorstr() 00127 * DESCRIPTION: return a string description of the current error condition 00128 */ 00129 char const *mad_stream_errorstr(struct mad_stream const *stream) 00130 { 00131 switch (stream->error) { 00132 case MAD_ERROR_NONE: return "no error"; 00133 00134 case MAD_ERROR_BUFLEN: return "input buffer too small (or EOF)"; 00135 case MAD_ERROR_BUFPTR: return "invalid (null) buffer pointer"; 00136 00137 case MAD_ERROR_NOMEM: return "not enough memory"; 00138 00139 case MAD_ERROR_LOSTSYNC: return "lost synchronization"; 00140 case MAD_ERROR_BADLAYER: return "reserved header layer value"; 00141 case MAD_ERROR_BADBITRATE: return "forbidden bitrate value"; 00142 case MAD_ERROR_BADSAMPLERATE: return "reserved sample frequency value"; 00143 case MAD_ERROR_BADEMPHASIS: return "reserved emphasis value"; 00144 00145 case MAD_ERROR_BADCRC: return "CRC check failed"; 00146 case MAD_ERROR_BADBITALLOC: return "forbidden bit allocation value"; 00147 case MAD_ERROR_BADSCALEFACTOR: return "bad scalefactor index"; 00148 case MAD_ERROR_BADMODE: return "bad bitrate/mode combination"; 00149 case MAD_ERROR_BADFRAMELEN: return "bad frame length"; 00150 case MAD_ERROR_BADBIGVALUES: return "bad big_values count"; 00151 case MAD_ERROR_BADBLOCKTYPE: return "reserved block_type"; 00152 case MAD_ERROR_BADSCFSI: return "bad scalefactor selection info"; 00153 case MAD_ERROR_BADDATAPTR: return "bad main_data_begin pointer"; 00154 case MAD_ERROR_BADPART3LEN: return "bad audio data length"; 00155 case MAD_ERROR_BADHUFFTABLE: return "bad Huffman table select"; 00156 case MAD_ERROR_BADHUFFDATA: return "Huffman data overrun"; 00157 case MAD_ERROR_BADSTEREO: return "incompatible block_type for JS"; 00158 } 00159 00160 return 0; 00161 }
Generated on Tue Jul 12 2022 23:11:09 by
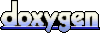