
MP3 Player without external hardware MP3 Player without external hardware. A software based MP3 player based on a modified version of libmad. Mono output (at the moment) via AnalogOut. Files are read from an USB drive. This is a demo program, it plays only one file at the moment. Documentation is in "main.cpp" and "config.h"
decoder.cpp
00001 /* 00002 * libmad - MPEG audio decoder library 00003 * Copyright (C) 2000-2004 Underbit Technologies, Inc. 00004 * 00005 * This program is free software; you can redistribute it and/or modify 00006 * it under the terms of the GNU General Public License as published by 00007 * the Free Software Foundation; either version 2 of the License, or 00008 * (at your option) any later version. 00009 * 00010 * This program is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00013 * GNU General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU General Public License 00016 * along with this program; if not, write to the Free Software 00017 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00018 * 00019 * $Id: decoder.c,v 1.1 2010/11/23 20:12:57 andy Exp $ 00020 */ 00021 00022 # include "config.h" 00023 00024 # include "global.h" 00025 00026 00027 # ifdef HAVE_SYS_TYPES_H 00028 # include <sys/types.h> 00029 # endif 00030 00031 # ifdef HAVE_SYS_WAIT_H 00032 # include <sys/wait.h> 00033 # endif 00034 00035 # ifdef HAVE_UNISTD_H 00036 # include <unistd.h> 00037 # endif 00038 00039 # ifdef HAVE_FCNTL_H 00040 # include <fcntl.h> 00041 # endif 00042 00043 # include <stdlib.h> 00044 00045 # ifdef HAVE_ERRNO_H 00046 # include <errno.h> 00047 # endif 00048 00049 # include "stream.h" 00050 # include "frame.h" 00051 # include "synth.h" 00052 # include "decoder.h" 00053 00054 /* 00055 * NAME: decoder->init() 00056 * DESCRIPTION: initialize a decoder object with callback routines 00057 */ 00058 void mad_decoder_init(struct mad_decoder *decoder, void *data, 00059 enum mad_flow (*input_func)(void *, 00060 struct mad_stream *), 00061 enum mad_flow (*header_func)(void *, 00062 struct mad_header const *), 00063 enum mad_flow (*filter_func)(void *, 00064 struct mad_stream const *, 00065 struct mad_frame *), 00066 enum mad_flow (*output_func)(void *, 00067 struct mad_header const *, 00068 struct mad_pcm *), 00069 enum mad_flow (*error_func)(void *, 00070 struct mad_stream *, 00071 struct mad_frame *), 00072 enum mad_flow (*message_func)(void *, 00073 void *, unsigned int *)) 00074 { 00075 decoder->mode = (enum mad_decoder_mode)-1; 00076 00077 decoder->options = 0; 00078 00079 00080 decoder->sync = 0; 00081 00082 decoder->cb_data = data; 00083 00084 decoder->input_func = input_func; 00085 decoder->header_func = header_func; 00086 decoder->filter_func = filter_func; 00087 decoder->output_func = output_func; 00088 decoder->error_func = error_func; 00089 decoder->message_func = message_func; 00090 } 00091 00092 int mad_decoder_finish(struct mad_decoder *decoder) 00093 { 00094 return 0; 00095 } 00096 00097 00098 static 00099 enum mad_flow error_default(void *data, struct mad_stream *stream, 00100 struct mad_frame *frame) 00101 { 00102 int *bad_last_frame = (int *)data; 00103 00104 switch (stream->error) { 00105 case MAD_ERROR_BADCRC: 00106 if (*bad_last_frame) 00107 mad_frame_mute(frame); 00108 else 00109 *bad_last_frame = 1; 00110 00111 return MAD_FLOW_IGNORE; 00112 00113 default: 00114 return MAD_FLOW_CONTINUE; 00115 } 00116 } 00117 00118 static 00119 int run_sync(struct mad_decoder *decoder) 00120 { 00121 enum mad_flow (*error_func)(void *, struct mad_stream *, struct mad_frame *); 00122 void *error_data; 00123 int bad_last_frame = 0; 00124 struct mad_stream *stream; 00125 struct mad_frame *frame; 00126 struct mad_synth *synth; 00127 int result = 0; 00128 00129 if (decoder->input_func == 0) 00130 return 0; 00131 00132 if (decoder->error_func) { 00133 error_func = decoder->error_func; 00134 error_data = decoder->cb_data; 00135 } 00136 else { 00137 error_func = error_default; 00138 error_data = &bad_last_frame; 00139 } 00140 00141 stream = &decoder->sync->stream; 00142 frame = &decoder->sync->frame; 00143 synth = decoder->sync->synth; 00144 00145 mad_stream_init(stream); 00146 mad_frame_init(frame); 00147 mad_synth_init(synth); 00148 00149 mad_stream_options(stream, decoder->options); 00150 00151 do { 00152 switch (decoder->input_func(decoder->cb_data, stream)) { 00153 case MAD_FLOW_STOP: 00154 goto done; 00155 case MAD_FLOW_BREAK: 00156 goto fail; 00157 case MAD_FLOW_IGNORE: 00158 continue; 00159 case MAD_FLOW_CONTINUE: 00160 break; 00161 } 00162 00163 while (1) { 00164 if (decoder->header_func) { 00165 if (mad_header_decode(&frame->header, stream) == -1) { 00166 if (!MAD_RECOVERABLE(stream->error)) 00167 break; 00168 00169 switch (error_func(error_data, stream, frame)) { 00170 case MAD_FLOW_STOP: 00171 goto done; 00172 case MAD_FLOW_BREAK: 00173 goto fail; 00174 case MAD_FLOW_IGNORE: 00175 case MAD_FLOW_CONTINUE: 00176 default: 00177 continue; 00178 } 00179 } 00180 00181 switch (decoder->header_func(decoder->cb_data, &frame->header)) { 00182 case MAD_FLOW_STOP: 00183 goto done; 00184 case MAD_FLOW_BREAK: 00185 goto fail; 00186 case MAD_FLOW_IGNORE: 00187 continue; 00188 case MAD_FLOW_CONTINUE: 00189 break; 00190 } 00191 } 00192 00193 if (mad_frame_decode(frame, stream) == -1) { 00194 if (!MAD_RECOVERABLE(stream->error)) 00195 break; 00196 00197 switch (error_func(error_data, stream, frame)) { 00198 case MAD_FLOW_STOP: 00199 goto done; 00200 case MAD_FLOW_BREAK: 00201 goto fail; 00202 case MAD_FLOW_IGNORE: 00203 break; 00204 case MAD_FLOW_CONTINUE: 00205 default: 00206 continue; 00207 } 00208 } 00209 else 00210 bad_last_frame = 0; 00211 00212 if (decoder->filter_func) { 00213 switch (decoder->filter_func(decoder->cb_data, stream, frame)) { 00214 case MAD_FLOW_STOP: 00215 goto done; 00216 case MAD_FLOW_BREAK: 00217 goto fail; 00218 case MAD_FLOW_IGNORE: 00219 continue; 00220 case MAD_FLOW_CONTINUE: 00221 break; 00222 } 00223 } 00224 00225 mad_synth_frame(synth, frame); 00226 00227 if (decoder->output_func) { 00228 switch (decoder->output_func(decoder->cb_data, 00229 &frame->header, &synth->pcm)) { 00230 case MAD_FLOW_STOP: 00231 goto done; 00232 case MAD_FLOW_BREAK: 00233 goto fail; 00234 case MAD_FLOW_IGNORE: 00235 case MAD_FLOW_CONTINUE: 00236 break; 00237 } 00238 } 00239 } 00240 } 00241 while (stream->error == MAD_ERROR_BUFLEN); 00242 00243 fail: 00244 result = -1; 00245 00246 done: 00247 mad_synth_finish(synth); 00248 mad_frame_finish(frame); 00249 mad_stream_finish(stream); 00250 00251 return result; 00252 } 00253 00254 00255 /* 00256 * NAME: decoder->run() 00257 * DESCRIPTION: run the decoder thread either synchronously or asynchronously 00258 */ 00259 int mad_decoder_run(struct mad_decoder *decoder, enum mad_decoder_mode mode) 00260 { 00261 int result; 00262 int (*run)(struct mad_decoder *) = 0; 00263 00264 switch (decoder->mode = mode) { 00265 case MAD_DECODER_MODE_SYNC: 00266 run = run_sync; 00267 break; 00268 00269 case MAD_DECODER_MODE_ASYNC: 00270 break; 00271 } 00272 00273 if (run == 0) 00274 return -1; 00275 // static struct mad_sync_s sync_mem; 00276 decoder->sync = (struct mad_sync_s *)malloc(sizeof(*decoder->sync)); 00277 #if defined(TARGET_LPC1768) 00278 decoder->sync->synth = (struct mad_synth *)mad_malloc(sizeof(struct mad_synth)); 00279 #else 00280 decoder->sync->synth = (struct mad_synth *)malloc(sizeof(struct mad_synth)); 00281 #endif 00282 if (decoder->sync == 0) 00283 return -1; 00284 00285 result = run(decoder); 00286 #if !defined(TARGET_LPC1768) 00287 free(decoder->sync->synth); 00288 #endif 00289 free(decoder->sync); 00290 decoder->sync = 0; 00291 reset_ahb_mem(); 00292 return result; 00293 } 00294 00295 /* 00296 * NAME: decoder->message() 00297 * DESCRIPTION: send a message to and receive a reply from the decoder process 00298 */ 00299 int mad_decoder_message(struct mad_decoder *decoder, 00300 void *message, unsigned int *len) 00301 { 00302 return -1; 00303 }
Generated on Tue Jul 12 2022 23:11:09 by
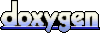