
MP3 Player without external hardware MP3 Player without external hardware. A software based MP3 player based on a modified version of libmad. Mono output (at the moment) via AnalogOut. Files are read from an USB drive. This is a demo program, it plays only one file at the moment. Documentation is in "main.cpp" and "config.h"
Stream.h
00001 /* mbed Microcontroller Library - Stream 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 * sford 00004 */ 00005 00006 #ifndef MBED_STREAM_H 00007 #define MBED_STREAM_H 00008 00009 #include "FileLike.h" 00010 #include "platform.h" 00011 #include <cstdio> 00012 00013 namespace mbed { 00014 00015 class Stream : public FileLike { 00016 00017 public: 00018 00019 Stream(const char *name = NULL); 00020 virtual ~Stream(); 00021 00022 int putc(int c) { 00023 fflush(_file); 00024 return std::fputc(c, _file); 00025 } 00026 int puts(const char *s) { 00027 fflush(_file); 00028 return std::fputs(s, _file); 00029 } 00030 int getc() { 00031 fflush(_file); 00032 return std::fgetc(_file); 00033 } 00034 char *gets(char *s, int size) { 00035 fflush(_file); 00036 return std::fgets(s,size,_file);; 00037 } 00038 int printf(const char* format, ...); 00039 int scanf(const char* format, ...); 00040 00041 operator std::FILE*() { return _file; } 00042 00043 #ifdef MBED_RPC 00044 virtual const struct rpc_method *get_rpc_methods(); 00045 #endif 00046 00047 protected: 00048 00049 virtual int close(); 00050 virtual ssize_t write(const void* buffer, size_t length); 00051 virtual ssize_t read(void* buffer, size_t length); 00052 virtual off_t lseek(off_t offset, int whence); 00053 virtual int isatty(); 00054 virtual int fsync(); 00055 virtual off_t flen(); 00056 00057 virtual int _putc(int c) = 0; 00058 virtual int _getc() = 0; 00059 00060 std::FILE *_file; 00061 00062 }; 00063 00064 } // namespace mbed 00065 00066 #endif 00067
Generated on Tue Jul 12 2022 23:11:09 by
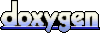