Simple driver for the ST7920 graphic LCD 128x64. Basic functions for displaying internal ROM fonts like a 16x4 LCD Extended function to fill the screen with a bitmap
st7920.cpp
00001 #include "st7920.h" 00002 00003 ST7920::ST7920 (PinName _RS,PinName _RW, PinName _E, PinName DB0, PinName DB1, PinName DB2, PinName DB3, PinName DB4, PinName DB5, PinName DB6, PinName DB7) 00004 : DB(DB0,DB1,DB2,DB3,DB4,DB5,DB6,DB7),RS(_RS), RW(_RW), E(_E) { 00005 00006 DB.output(); 00007 E.write(0); 00008 RS.write(0); 00009 RW.write(0); 00010 InitDisplay(); 00011 } 00012 00013 unsigned int ST7920::ByteReadLCD() { 00014 unsigned int data; 00015 00016 DB.input(); 00017 E.write(1); 00018 data = DB.read(); 00019 E.write(0); 00020 DB.output(); 00021 00022 return data; 00023 } 00024 00025 void ST7920::ByteWriteLCD(unsigned int data) { 00026 DB.output(); 00027 E.write(1); 00028 DB.write(data); 00029 E.write(0); 00030 } 00031 00032 void ST7920::WriteInstruction(unsigned int Command) { 00033 ReadBusyFlag(); 00034 RS.write(0); 00035 RW.write(0); 00036 ByteWriteLCD(Command); 00037 } 00038 00039 void ST7920::WriteRAM(unsigned int data) { 00040 ReadBusyFlag(); 00041 RS.write(1); 00042 RW.write(0); 00043 ByteWriteLCD(data); 00044 } 00045 00046 void ST7920::ReadBusyFlag() { 00047 unsigned char data; 00048 00049 RS.write(0); 00050 RW.write(1); 00051 while ((data & 0x7F) == BUSY_FLAG_BF) { 00052 data = ByteReadLCD(); 00053 } 00054 } 00055 00056 unsigned int ST7920::Read_AC() { 00057 RS.write(0); 00058 RW.write(1); 00059 return (ByteReadLCD() & 0x7F); 00060 } 00061 00062 unsigned int ST7920::ReadRAM() { 00063 ReadBusyFlag(); 00064 RS.write(1); 00065 RW.write(1); 00066 return ByteReadLCD(); 00067 } 00068 00069 void ST7920::InitDisplay() { 00070 wait_ms(40); // wait 40ms 00071 E.write(0); 00072 WriteInstruction(FUNCTION_SET | DATA_LENGTH_DL); // 8 bits interface, RE=0 00073 wait_us(100); // wait 100us 00074 WriteInstruction(FUNCTION_SET | DATA_LENGTH_DL); // again 00075 wait_us(37); // wait 37us 00076 WriteInstruction(DISPLAY_CONTROL | DISPLAY_ON_D ); // display on 00077 wait_us(100); // wait 100us 00078 WriteInstruction(DISPLAY_CLEAR); // clear display 00079 wait_ms(10); // wait 10ms 00080 WriteInstruction(ENTRY_MODE_SET | INCREASE_DECREASE_ID); // move cursor right 00081 wait_ms(10); // wait 10ms 00082 WriteInstruction(RETURN_HOME); 00083 SetGraphicsMode(); 00084 } 00085 00086 //************************************************************************************************ 00087 //public methodes 00088 void ST7920::SetGraphicsMode() { 00089 WriteInstruction(EXTENDED_FUNCTION_SET | DATA_LENGTH_DL); 00090 WriteInstruction(EXTENDED_FUNCTION_SET | DATA_LENGTH_DL | EXTENDED_INSTRUCTION_RE); //RE=1 (Extended funtion set) 00091 WriteInstruction(EXTENDED_FUNCTION_SET | DATA_LENGTH_DL | EXTENDED_INSTRUCTION_RE | GRAPHIC_ON_G); 00092 } 00093 00094 void ST7920::SetTextMode() { 00095 WriteInstruction(FUNCTION_SET | DATA_LENGTH_DL); // RE=0 (Basic funtion set) 00096 } 00097 00098 void ST7920::ClearScreen() { 00099 WriteInstruction(FUNCTION_SET | DATA_LENGTH_DL); // RE=0 (Basic funtion set) 00100 WriteInstruction(DISPLAY_CLEAR); 00101 } 00102 00103 void ST7920::ReturnHome() { 00104 WriteInstruction(FUNCTION_SET | DATA_LENGTH_DL); //RE=0 (Basic funtion set) 00105 WriteInstruction(RETURN_HOME); 00106 } 00107 00108 void ST7920::Standby() { 00109 WriteInstruction(EXTENDED_FUNCTION_SET | DATA_LENGTH_DL | EXTENDED_INSTRUCTION_RE); //RE=1 (Extended funtion set) 00110 WriteInstruction(STANDBY); 00111 } 00112 00113 //Basic text functions 00114 void ST7920::DisplayString(int Row,int Column,unsigned char *ptr,int length) { 00115 int i=0; 00116 00117 switch (Row) { 00118 case 0: 00119 Column|=0x80; 00120 break; 00121 case 1: 00122 Column|=0x90; 00123 break; 00124 case 2: 00125 Column|=0x88; 00126 break; 00127 case 3: 00128 Column|=0x98; 00129 break; 00130 default: 00131 Column=0x80; 00132 break; 00133 } 00134 00135 if (Column%2!=0) { 00136 Column-=1; 00137 i=1; 00138 } 00139 WriteInstruction((unsigned int)Column); 00140 00141 if (i==1) { 00142 WriteRAM(' '); 00143 } 00144 for (i=0; i<length; i++) { 00145 WriteRAM((unsigned int)ptr[i]); 00146 } 00147 } 00148 00149 void ST7920::DisplayChar(int Row,int Column,int inpChr) { 00150 int i=0; 00151 00152 switch (Row) { 00153 case 0: 00154 Column|=0x80; // SET_DDRAM_ADDRESS 00155 break; 00156 case 1: 00157 Column|=0x90; 00158 break; 00159 case 2: 00160 Column|=0x88; 00161 break; 00162 case 3: 00163 Column|=0x98; 00164 break; 00165 default: 00166 Column=0x80; 00167 break; 00168 } 00169 00170 if (Column%2!=0) { 00171 Column-=1; 00172 i=1; 00173 } 00174 WriteInstruction((unsigned int)Column); 00175 00176 if (i==1) { 00177 WriteRAM(' '); 00178 } 00179 WriteRAM((unsigned int)inpChr); 00180 } 00181 00182 // Graphic functions 00183 void ST7920::FillGDRAM(unsigned char *bitmap) { 00184 unsigned char i, j, k ; 00185 00186 for ( i = 0 ; i < 2 ; i++ ) { 00187 for ( j = 0 ; j < 32 ; j++ ) { 00188 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS | j) ; 00189 if ( i == 0 ) { 00190 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS) ; 00191 } else { 00192 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS | 0x08) ; 00193 } 00194 for ( k = 0 ; k < 16 ; k++ ) { 00195 WriteRAM( *bitmap++ ) ; 00196 } 00197 } 00198 } 00199 } 00200 00201 void ST7920::FillGDRAM_Turned(unsigned char *bitmap) { 00202 int i, j, k, m, offset_row, mask ; 00203 unsigned char data; 00204 00205 for ( i = 0 ; i < 2 ; i++ ) { //upper and lower page 00206 for ( j = 0 ; j < 32 ; j++ ) { //32 lines per page 00207 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS | j) ; 00208 if ( i == 0 ) { 00209 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS) ; 00210 } else { 00211 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS | 0x08) ; 00212 } 00213 mask=1<<(j%8); // extract bitnumber 00214 //printf("mask: %d\r\n",mask); 00215 for ( k = 0 ; k < 16 ; k++ ) { //16 bytes per line 00216 offset_row=((i*32+j)/8)*128 + k*8; //y coordinate/8 = row 0-7 * 128 = byte offset, read 8 bytes 00217 data=0; 00218 for (m = 0 ; m < 8 ; m++) { // read 8 bytes from source 00219 00220 if ((bitmap[offset_row+m] & mask)) { //pixel = 1 00221 data|=(128>>m); 00222 } 00223 } 00224 WriteRAM(data) ; 00225 } 00226 } 00227 } 00228 } 00229 00230 void ST7920::ClearGDRAM() { 00231 unsigned char i, j, k ; 00232 00233 for ( i = 0 ; i < 2 ; i++ ) { 00234 for ( j = 0 ; j < 32 ; j++ ) { 00235 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS | j) ; 00236 if ( i == 0 ) { 00237 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS) ; 00238 } else { 00239 WriteInstruction(SET_GRAPHIC_RAM_ADDRESS | 0x08) ; 00240 } 00241 for ( k = 0 ; k < 16 ; k++ ) { 00242 WriteRAM(0); 00243 } 00244 } 00245 } 00246 }
Generated on Wed Jul 13 2022 13:05:09 by
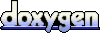