
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
xbox360gamepad.h
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef XBOX360GAMEPAD_H 00024 #define XBOX360GAMEPAD_H 00025 00026 #include "usbeh_api.h" 00027 00028 #define BUTTON_PRESSED 1 00029 #define BUTTON_RELEASED 0 00030 00031 #define BUTTON_HOLD_TIME 3000 00032 00033 #define BUTT_LS_PRESS 1 00034 #define BUTT_RS_PRESS 2 00035 #define BUTT_XBOX_PRESS 3 00036 #define BUTT_UNUSED_PRESS 4 00037 #define BUTT_A_PRESS 5 00038 #define BUTT_B_PRESS 6 00039 #define BUTT_X_PRESS 7 00040 #define BUTT_Y_PRESS 8 00041 #define BUTT_DPAD_UP_PRESS 9 00042 #define BUTT_DPAD_DOWN_PRESS 10 00043 #define BUTT_DPAD_LEFT_PRESS 11 00044 #define BUTT_DPAD_RIGHT_PRESS 12 00045 #define BUTT_START_PRESS 13 00046 #define BUTT_BACK_PRESS 14 00047 #define BUTT_LEFT_HAT_PRESS 15 00048 #define BUTT_RIGHT_HAT_PRESS 16 00049 00050 #define BUTT_LS_RELEASE (BUTT_LS_PRESS + 16) 00051 #define BUTT_RS_RELEASE (BUTT_RS_PRESS + 16) 00052 #define BUTT_XBOX_RELEASE (BUTT_XBOX_PRESS + 16) 00053 #define BUTT_UNUSED_RELEASE (BUTT_UNUSED_PRESS + 16) 00054 #define BUTT_A_RELEASE (BUTT_A_PRESS + 16) 00055 #define BUTT_B_RELEASE (BUTT_B_PRESS + 16) 00056 #define BUTT_X_RELEASE (BUTT_X_PRESS + 16) 00057 #define BUTT_Y_RELEASE (BUTT_Y_PRESS + 16) 00058 #define BUTT_DPAD_UP_RELEASE (BUTT_DPAD_UP_PRESS + 16) 00059 #define BUTT_DPAD_DOWN_RELEASE (BUTT_DPAD_DOWN_PRESS + 16) 00060 #define BUTT_DPAD_LEFT_RELEASE (BUTT_DPAD_LEFT_PRESS + 16) 00061 #define BUTT_DPAD_RIGHT_RELEASE (BUTT_DPAD_RIGHT_PRESS + 16) 00062 #define BUTT_START_RELEASE (BUTT_START_PRESS + 16) 00063 #define BUTT_BACK_RELEASE (BUTT_BACK_PRESS + 16) 00064 #define BUTT_LEFT_HAT_RELEASE (BUTT_LEFT_HAT_PRESS + 16) 00065 #define BUTT_RIGHT_HAT_RELEASE (BUTT_RIGHT_HAT_PRESS + 16) 00066 00067 #define BUTT_LS_HOLD (BUTT_LS_PRESS + 32) 00068 #define BUTT_RS_HOLD (BUTT_RS_PRESS + 32) 00069 #define BUTT_XBOX_HOLD (BUTT_XBOX_PRESS + 32) 00070 #define BUTT_UNUSED_HOLD (BUTT_UNUSED_PRESS + 32) 00071 #define BUTT_A_HOLD (BUTT_A_PRESS + 32) 00072 #define BUTT_B_HOLD (BUTT_B_PRESS + 32) 00073 #define BUTT_X_HOLD (BUTT_X_PRESS + 32) 00074 #define BUTT_Y_HOLD (BUTT_Y_PRESS + 32) 00075 #define BUTT_DPAD_UP_HOLD (BUTT_DPAD_UP_PRESS + 32) 00076 #define BUTT_DPAD_DOWN_HOLD (BUTT_DPAD_DOWN_PRESS + 32) 00077 #define BUTT_DPAD_LEFT_HOLD (BUTT_DPAD_LEFT_PRESS + 32) 00078 #define BUTT_DPAD_RIGHT_HOLD (BUTT_DPAD_RIGHT_PRESS + 32) 00079 #define BUTT_START_HOLD (BUTT_START_PRESS + 32) 00080 #define BUTT_BACK_HOLD (BUTT_BACK_PRESS + 32) 00081 #define BUTT_LEFT_HAT_HOLD (BUTT_LEFT_HAT_PRESS + 32) 00082 #define BUTT_RIGHT_HAT_HOLD (BUTT_RIGHT_HAT_PRESS + 32) 00083 00084 #define LED_ALL_OFF 0x00 00085 #define LED_ALL_BLINKING 0x01 00086 #define LED_1_FLASH_THEN_ON 0x02 00087 #define LED_2_FLASH_THEN_ON 0x03 00088 #define LED_3_FLASH_THEN_ON 0x04 00089 #define LED_4_FLASH_THEN_ON 0x05 00090 #define LED_1_ON 0x06 00091 #define LED_2_ON 0x07 00092 #define LED_3_ON 0x08 00093 #define LED_4_ON 0x09 00094 #define LED_ROTATING 0x0A 00095 #define LED_BLINKING 0x0B 00096 #define LED_SLOW_BLINKING 0x0C 00097 #define LED_ALTERNATING 0x0D 00098 00099 typedef struct { 00100 uint16_t idVendor; 00101 uint16_t idProduct; 00102 const char* name; 00103 } XBOX360_DEVICE; 00104 00105 typedef struct { 00106 unsigned state; 00107 unsigned mask; 00108 unsigned count; 00109 USBEH_SOF_COUNTER pressHold; 00110 } XBOX360_BUTTON; 00111 00112 typedef struct { 00113 short x; 00114 short y; 00115 short x_previous; 00116 short y_previous; 00117 } XBOX360_STICK; 00118 00119 /* API functions. */ 00120 char xbox360gamepad_get_button(void); 00121 char xbox360gamepad_get_button_preview(void); 00122 unsigned char xbox360gamepad_get_trigger_left(void); 00123 unsigned char xbox360gamepad_get_trigger_right(void); 00124 void xbox360gamepad_led(int code); 00125 XBOX360_STICK * xbox360gamepad_get_stick_left(void); 00126 XBOX360_STICK * xbox360gamepad_get_stick_right(void); 00127 00128 int xbox360gamepad_init(void); 00129 void xbox360gamepad_process(void); 00130 00131 /* Button press and hold callback function. */ 00132 void xbox360gamepad_button_hold_callback(USBEH_SOF_COUNTER *q); 00133 00134 /* Onload callback function. */ 00135 int xbox360gamepad_onload_callback(int device, USBEH_deviceDescriptor *deviceDesc, USBEH_interfaceDescriptor **interfaceDesc); 00136 00137 void xbox360_chatpad_init(void); 00138 00139 #endif
Generated on Tue Jul 12 2022 18:05:35 by
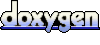