
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
utils.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 00024 #include "sowb.h" 00025 #include "ctype.h" 00026 #include "utils.h" 00027 #include "gps.h" 00028 #include "debug.h" 00029 00030 /** ascii2bin 00031 * 00032 * Converts an ascii char to binary nibble. 00033 * 00034 * @param char The character to convert [0-9][a-f][A-F] 00035 * @return char the bin value or -1 on invalid hex char. 00036 */ 00037 char ascii2bin(char c) { 00038 if (c >= '0' && c <= '9') return (c - '0') & 0xF; 00039 if (c >= 'A' && c <= 'F') return (c - 'A' + 10) & 0xF; 00040 if (c >= 'a' && c <= 'f') return (c - 'a' + 10) & 0xF; 00041 return (char)0xFF; 00042 } 00043 00044 /** hex2bin 00045 * 00046 * Converts a hex ascii string to binary int. 00047 * 00048 * Note, no error checking, assume string is valid hex chars [0-9][a-f][A-F] 00049 * 00050 * @param char *s The string to convert. 00051 * @param int len The length of the string to convert. 00052 * @return uint32_t the converted value. 00053 */ 00054 uint32_t hex2bin(char *s, int len) { 00055 int i; 00056 uint32_t rval; 00057 00058 for (rval = 0, i = 0; i < len; i++) rval = rval | (ascii2bin(*(s + i)) << ((len - i - 1) * 4)); 00059 00060 return rval; 00061 } 00062 00063 /** bin2ascii 00064 * 00065 * Convert a nibble to an ASCII character 00066 * 00067 * @param char c The nibble to convert 00068 * @return char The character representation of the nibble. 00069 */ 00070 char bin2ascii(char c) { 00071 c &= 0xF; 00072 if (c < 0xA) return c + '0'; 00073 return c + 'A' - 10; 00074 } 00075 00076 /** bin2hex 00077 * 00078 * Convert a binary to a hex string representation. 00079 * The caller should allocate a buffer for *s before 00080 * calling this function. The allocation should be 00081 * len + 1 in length to hold the string and the 00082 * terminating null character. 00083 * 00084 * @param uint32_t d The value to convert. 00085 * @param int len The string length. 00086 * @param char *s Where to put the string. 00087 * @return char * Returns *s passed in. 00088 */ 00089 // 238E,238E 00090 // O832,O832 00091 00092 char * bin2hex(uint32_t d, int len, char *s) { 00093 char c, i = 0; 00094 *(s + len) = '\0'; 00095 while (len) { 00096 c = (d >> (4 * (len - 1))) & 0xF; 00097 *(s + i) = bin2ascii(c); 00098 len--; i++; 00099 } 00100 return s; 00101 } 00102 00103 /** dec2bin 00104 * 00105 * Converts a decimal ascii string to binary int. 00106 * 00107 * Note, no error checking, assume string is valid hex chars [0-9] 00108 * 00109 * @param char *s The string to convert. 00110 * @param int len The length of the string to convert. 00111 * @return uint32_t the converted value. 00112 */ 00113 uint32_t dec2bin(char *s, int len) { 00114 int i, mul; 00115 uint32_t rval = 0; 00116 00117 for (mul = 1, i = len; i; i--, mul *= 10) rval += (ascii2bin(*(s + i - 1)) * mul); 00118 00119 return rval; 00120 } 00121 00122 /** strcsuml 00123 * 00124 * Return a two's compliment checksum char for th esupplied string. 00125 * 00126 * @param char * s The string to sum 00127 * @param int len The length of the string. 00128 * @return The two's compliment char. 00129 */ 00130 char strcsuml(char *s, int len) { 00131 char sum = 0; 00132 while (len) { 00133 sum += *(s +len - 1); 00134 } 00135 return (~sum) + 1; 00136 } 00137 00138 /** strcsum 00139 * 00140 * Return a two's compliment checksum char for the null terminated supplied string. 00141 * 00142 * @param char * s The string to sum 00143 * @return The two's compliment char. 00144 */ 00145 char strcsum(char *s) { 00146 return strcsuml(s, strlen(s)); 00147 } 00148 00149 /** strsuml 00150 * 00151 * Return the 8bit sum char for the supplied string. 00152 * 00153 * @param char * s The string to sum 00154 * @param int len The length of the string. 00155 * @return The sum 00156 */ 00157 char strsuml(char *s, int len) { 00158 char sum = 0; 00159 while (len) { 00160 sum += *(s +len - 1); 00161 } 00162 return sum; 00163 } 00164 00165 /** strsum 00166 * 00167 * Return the 8bit sum of all the characters of the supplied string. 00168 * 00169 * @param char * s The string to sum 00170 * @return The sum 00171 */ 00172 char strsum(char *s) { 00173 return strsuml(s, strlen(s)); 00174 } 00175 00176 /* Used for the date_AsString function. */ 00177 const char month_abv[][4] = { "Jan","Feb","Mar","Apr","May","Jun","Jul","Aug","Sep","Oct","Nov","Dec","Wtf" }; 00178 00179 /** date_AsString 00180 * 00181 * Used to get the current date and return a formatted string. 00182 * Note, the caller must have a 12byte buffer in place, pointed 00183 * to by s to accept the string. 00184 * 00185 * @param GPS_TIME *t A pointer to the time data struct to "print as" 00186 * @param char *s A pointer to a buffer to hold the formatted string. 00187 */ 00188 void date_AsString(GPS_TIME *t, char *s) { 00189 int month = t->month - 1; if (month > 11 || month < 0) month = 12; /* Ensure in range. */ 00190 sprintf(s, "%4.4d/%s/%2.2d", t->year, month_abv[month], t->day); 00191 } 00192 00193 /** time_AsString 00194 * 00195 * Used to get the current time and return a formatted string. 00196 * Note, the caller must have a 12byte buffer in place, pointed 00197 * to by s to accept the string. 00198 * 00199 * @param GPS_TIME *t A pointer to the time data struct to "print as" 00200 * @param char *s A pointer to a buffer to hold the formatted string. 00201 */ 00202 void time_AsString(GPS_TIME *t, char *s) { 00203 sprintf(s, "%2.2d:%2.2d:%2.2d.%1.1d%1.1d", t->hour, t->minute, t->second, t->tenth, t->hundreth); 00204 } 00205 00206 /** double2dms 00207 * 00208 * Takes double and converts it to a printable display string of 00209 * degrees, minutes and seconds. 00210 * The caller is responsible for allocating the buffer *s before 00211 * calling this function. 00212 * 00213 * @param char *s A pointer to the buffer to print to. 00214 * @param double d The value to print. 00215 */ 00216 void double2dms(char *s, double d) { 00217 int degrees, minutes; 00218 double seconds, t; 00219 00220 degrees = (int)d; t = (d - (double)degrees) * 60.; 00221 minutes = (int)t; 00222 seconds = (t - (double)minutes) * 60.; 00223 00224 sprintf(s, "%03d\xb0%02d\x27%02d\x22", degrees, minutes, (int)seconds); 00225 } 00226 00227 /** printDouble 00228 * 00229 * Print a double to a string buffer with correct leading zero(s). 00230 * The caller is responsible for allocating the buffer *s before 00231 * calling this function. 00232 * 00233 * @param char *s A pointer to the buffer to print to. 00234 * @param double d The value to print. 00235 */ 00236 void printDouble(char *s, double d) { 00237 if (isnan(d)) sprintf(s, "---.----"); 00238 else if (d > 100.) sprintf(s, "%.4f", d); 00239 else if (d > 10.) sprintf(s, "0%.4f", d); 00240 else sprintf(s, "00%.4f", d); 00241 } 00242 00243 /** printDouble_3_1 00244 * 00245 * Print a double to a string buffer with correct leading zero(s). 00246 * The caller is responsible for allocating the buffer *s before 00247 * calling this function. 00248 * 00249 * @param char *s A pointer to the buffer to print to. 00250 * @param double d The value to print. 00251 */ 00252 char * printDouble_3_1(char *s, double d) { 00253 char temp[16]; 00254 if (isnan(d)) sprintf(temp, "---.-"); 00255 else if (d > 100.) sprintf(temp, "%.6f", d); 00256 else if (d > 10.) sprintf(temp, "0%.6f", d); 00257 else sprintf(temp, "00%.6f", d); 00258 memcpy(s, temp, 5); 00259 *(s+5) = '\0'; 00260 return s; 00261 } 00262 00263 /** printDouble_3_2 00264 * 00265 * Print a double to a string buffer with correct leading zero(s). 00266 * The caller is responsible for allocating the buffer *s before 00267 * calling this function. 00268 * 00269 * @param char *s A pointer to the buffer to print to. 00270 * @param double d The value to print. 00271 */ 00272 char * printDouble_3_2(char *s, double d) { 00273 char temp[16]; 00274 if (isnan(d)) sprintf(temp, "---.--"); 00275 else if (d > 100.) sprintf(temp, "%.6f", d); 00276 else if (d > 10.) sprintf(temp, "0%.6f", d); 00277 else sprintf(temp, "00%.6f", d); 00278 memcpy(s, temp, 6); 00279 *(s+6) = '\0'; 00280 return s; 00281 } 00282 00283 void printBuffer(char *s, int len) { 00284 #ifdef DEBUG_ON 00285 for (int i = 0; i < len / 0x10; i++) { 00286 debug_printf("%02X: ", i); 00287 for (int j = 0; j < 0x10; j++) { 00288 debug_printf("%02X ", s[(i * 0x10) + j]); 00289 if (j == 7) debug_printf(" "); 00290 } 00291 for (int j = 0; j < 0x10; j++) { 00292 if (isprint(s[(i * 0x10) + j])) { 00293 debug_printf("%c", s[(i * 0x10) + j]); 00294 } 00295 else { 00296 debug_printf("."); 00297 } 00298 if (j == 7) debug_printf(" "); 00299 } 00300 debug_printf("\r\n"); 00301 } 00302 #endif 00303 } 00304 00305 inline void disable_irqs(void) { 00306 NVIC_DisableIRQ(EINT3_IRQn); 00307 NVIC_DisableIRQ(RIT_IRQn); 00308 NVIC_DisableIRQ(UART0_IRQn); 00309 NVIC_DisableIRQ(UART1_IRQn); 00310 NVIC_DisableIRQ(UART2_IRQn); 00311 NVIC_DisableIRQ(USB_IRQn); 00312 } 00313 00314 inline void enable_irqs(void) { 00315 NVIC_EnableIRQ(USB_IRQn); 00316 NVIC_EnableIRQ(EINT3_IRQn); 00317 NVIC_EnableIRQ(RIT_IRQn); 00318 NVIC_EnableIRQ(UART0_IRQn); 00319 NVIC_EnableIRQ(UART1_IRQn); 00320 NVIC_EnableIRQ(UART2_IRQn); 00321 }
Generated on Tue Jul 12 2022 18:05:35 by
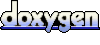