
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
user.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "rit.h" 00024 #include "gpio.h" 00025 #include "user.h" 00026 #include "nexstar.h" 00027 #include "xbox360gamepad.h" 00028 #include "debug.h" 00029 00030 #define STICK_DIVISOR 4096.0 00031 00032 void handle_stick_left(void); 00033 void handle_stick_right(void); 00034 00035 typedef void (PROCESS_FUNC)(); 00036 00037 extern PROCESS_FUNC *process_callbacks[]; 00038 00039 USER_INPUT user_input; 00040 00041 /** user_get_button 00042 * 00043 * Used to get a "button press" from the user. This is basically 00044 * a read of the XBox360 gamepad interface via the USBEH system. 00045 * However, this function is provided to abstract that so that 00046 * other systems can "inject" button presses into the system. 00047 * The intention here is for a virtual console on a PC to 00048 * control the SOWB system via the (to be done) pccomms.c module. 00049 * This is just a conveinent way to get user IO into the SOWB. 00050 * 00051 * Note, this fnction calls all the module _process() functions 00052 * in it's loop (ie while no user IO is occuring). That gives the 00053 * SOWB modules a chance to get work done outside of time critical 00054 * interrupts. _process() functions should NEVER block and should 00055 * also be "fast" so as to avoid any user IO perseptive delay. 00056 * 00057 * Note, if arg peek is true, we return the next button press in 00058 * the buffer without actually removing that event from the buffer. 00059 * Allows us to look to see if an pending button press is within 00060 * the buffer awaiting being handled. 00061 * 00062 * @param bool peek 00063 * @return char The button pressed. 00064 */ 00065 char user_get_button(bool peek) { 00066 char c; 00067 00068 if (peek) { 00069 /* Make a call to all _process()'s just in case we find 00070 ourselves stuck in a tight loop waiting for an event. */ 00071 for (c = 0; process_callbacks[c] != NULL; c++) (process_callbacks[c])(); 00072 return xbox360gamepad_get_button_preview(); 00073 } 00074 00075 do { 00076 /* Call all module _process functions. */ 00077 for (c = 0; process_callbacks[c] != NULL; c++) { 00078 KICK_WATCHDOG; 00079 (process_callbacks[c])(); 00080 } 00081 00082 handle_stick_left(); 00083 handle_stick_right(); 00084 00085 /* Get a button press from the user or go round again. */ 00086 c = xbox360gamepad_get_button(); 00087 } 00088 while (c == 0); 00089 00090 return c; 00091 } 00092 00093 /* A flag to signify a timeout has occured. */ 00094 volatile int user_wait_ms_flag; 00095 00096 /** _user_wait_ms_cb 00097 * 00098 * Called by the RIT system to signal a timer timeout. 00099 * 00100 * @param int t_index The timer index value (handle). 00101 */ 00102 void _user_wait_ms_cb(int t_index) { 00103 user_wait_ms_flag = 0; 00104 } 00105 00106 /** user_wait_ms 00107 * 00108 * Used to "wait" for a specified time interval. 00109 * Note, while "waiting" for the timeout, it will 00110 * call all the modules _process() functions to 00111 * allow modules to perform non-time critical work 00112 * outside of interrupts. 00113 * 00114 * @param uint32_t ms The delay amount. 00115 */ 00116 void user_wait_ms(uint32_t ms) { 00117 rit_timer_set_counter(RIT_TIMER_CB_WAIT, ms); 00118 user_wait_ms_flag = 1; 00119 while (user_wait_ms_flag) 00120 for (int c = 0; process_callbacks[c] != NULL; c++) { 00121 KICK_WATCHDOG; 00122 (process_callbacks[c])(); 00123 } 00124 } 00125 00126 /** user_wait_ms 00127 * 00128 * Used to "wait" for a specified time interval. 00129 * Note, unlike the function above, NO _process() 00130 * functions are called. This really is a blocking 00131 * delay and should be used with care! 00132 * 00133 * @param uint32_t ms The delay amount. 00134 */ 00135 void user_wait_ms_blocking(uint32_t ms) { 00136 rit_timer_set_counter(RIT_TIMER_CB_WAIT, ms); 00137 user_wait_ms_flag = 1; 00138 while (user_wait_ms_flag) KICK_WATCHDOG; 00139 } 00140 00141 /** user_call_process 00142 */ 00143 void user_call_process(void) { 00144 for (int c = 0; process_callbacks[c] != NULL; c++) { 00145 KICK_WATCHDOG; 00146 (process_callbacks[c])(); 00147 } 00148 } 00149 00150 void handle_stick_left(void) { 00151 static XBOX360_STICK stick_left_previous; 00152 XBOX360_STICK *stick; 00153 int x, y; 00154 double rate; 00155 00156 stick = xbox360gamepad_get_stick_left(); 00157 if (stick->x/STICK_DIVISOR != stick_left_previous.x/STICK_DIVISOR || stick->y/STICK_DIVISOR != stick_left_previous.y/STICK_DIVISOR) { 00158 stick_left_previous.x = stick->x; 00159 stick_left_previous.y = stick->y; 00160 x = stick->x/STICK_DIVISOR; 00161 y = stick->y/STICK_DIVISOR; 00162 00163 if (x > 2 || x < -2) { 00164 rate = 6.0 * (double)((double)stick->x/STICK_DIVISOR / 16.0); 00165 _nexstar_set_azmith_rate_coarse(rate); 00166 } 00167 else { 00168 _nexstar_set_azmith_rate_coarse(0.0); 00169 } 00170 00171 if (y > 2 || y < -2) { 00172 rate = 6.0 * (double)((double)stick->y/STICK_DIVISOR / 16.0); 00173 _nexstar_set_elevation_rate_coarse(rate); 00174 } 00175 else { 00176 _nexstar_set_elevation_rate_coarse(0.0); 00177 } 00178 } 00179 } 00180 00181 void handle_stick_right(void) { 00182 static XBOX360_STICK stick_previous; 00183 XBOX360_STICK *stick; 00184 int x, y; 00185 double rate; 00186 00187 stick = xbox360gamepad_get_stick_right(); 00188 if (stick->x/STICK_DIVISOR != stick_previous.x/STICK_DIVISOR || stick->y/STICK_DIVISOR != stick_previous.y/STICK_DIVISOR) { 00189 stick_previous.x = stick->x; 00190 stick_previous.y = stick->y; 00191 x = stick->x/STICK_DIVISOR; 00192 y = stick->y/STICK_DIVISOR; 00193 00194 if (x > 2 || x < -2) { 00195 //debug_printf("New RIGHT stick position x = %d y = %d\r\n", stick->x/STICK_DIVISOR, stick->y/STICK_DIVISOR); 00196 rate = 0.4 * (double)((double)stick->x/STICK_DIVISOR / 16.0); 00197 _nexstar_set_azmith_rate_fine(rate); 00198 } 00199 else { 00200 _nexstar_set_azmith_rate_fine(0.0); 00201 } 00202 00203 if (y > 2 || y < -2) { 00204 rate = 0.4 * (double)((double)stick->y/STICK_DIVISOR / 16.0); 00205 _nexstar_set_elevation_rate_fine(rate); 00206 } 00207 else { 00208 _nexstar_set_elevation_rate_fine(0.0); 00209 } 00210 } 00211 } 00212 00213 00214
Generated on Tue Jul 12 2022 18:05:35 by
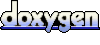