
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
usbeh_controller.h
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef USBEH_CONTROLLER_H 00024 #define USBEH_CONTROLLER_H 00025 00026 #include "usbeh.h" 00027 #include "usbeh_device.h" 00028 #include "usbeh_endpoint.h" 00029 00030 class USBEH_Controller { 00031 00032 public: 00033 00034 USBEH_HCCA hcca; 00035 USBEH_Endpoint endpoints[USBEH_MAX_ENDPOINTS_TOTAL]; 00036 USBEH_Endpoint endpointZero; 00037 USBEH_HCTD commonTail; 00038 USBEH_Setup setupZero; 00039 USBEH_Device devices[USBEH_MAX_DEVICES]; 00040 00041 USBEH_U32 frameNumber; 00042 USBEH_U08 callbacksPending; 00043 USBEH_U08 rootHubStatusChange; 00044 USBEH_U08 unused0; 00045 USBEH_U08 unused1; 00046 USBEH_U08 connectPending; 00047 USBEH_U08 connectCountdown; 00048 USBEH_U08 connectHub; 00049 USBEH_U08 connectPort; 00050 00051 USBEH_U08 SRAM[0]; 00052 00053 // Methods. 00054 void init(void); 00055 void process(void); 00056 int initHub(int device); 00057 int transfer(USBEH_Endpoint *endpoint, int token, USBEH_U08 *data, int length, int state); 00058 bool remove(USBEH_HCED *ed, volatile USBEH_HCED **queue); 00059 void release(USBEH_Endpoint *endpoint); 00060 int addEndpoint(int device, USBEH_endpointDescriptor *endpoint); 00061 int addEndpoint(int device, int endpoint, int attributes, int maxPacketSize, int interval); 00062 int addDevice(int hub, int port, bool isLowSpeed); 00063 int addDeviceCore(int hub, int port, bool isLowSpeed); 00064 int setConfigurationAndInterface(int device, int configuration, int interfaceNumber, USBEH_deviceDescriptor *desc); 00065 void processDoneQueue(USBEH_U32 tdList); 00066 void resetPort(int hub, int port); 00067 void connect(int hub, int port, bool lowSpeed); 00068 void disconnect(int hub, int port); 00069 void hubStatusChange(int hub, int port, USBEH_U32 status); 00070 void delayMS(int ms); 00071 void initHW(USBEH_HCCA *cca); 00072 void hubInterrupt(int device); 00073 static void hubInterruptCallback(int device, int endpoint, int status, USBEH_U08 *data, int length, void *userData); 00074 USBEH_HCTD * reverse(USBEH_HCTD *current); 00075 USBEH_Endpoint * getEndpoint(int device, int ep); 00076 USBEH_Endpoint * allocateEndpoint(int device, int endpointAddress, int type, int maxPacketSize); 00077 00078 }; 00079 00080 00081 // END #ifndef USBEH_CONTROLLER_H 00082 #endif
Generated on Tue Jul 12 2022 18:05:35 by
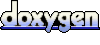