
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
th_xbox360gamepad.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #ifndef TH_XBOX360GAMEPAD_C 00024 #define TH_XBOX360GAMEPAD_C 00025 #endif 00026 00027 #include "mbed.h" 00028 #include "usbeh.h" 00029 #include "usbeh_endpoint.h" 00030 #include "usbeh_device.h" 00031 #include "usbeh_controller.h" 00032 #include "usbeh_api.h" 00033 #include "xbox360gamepad.h" 00034 #include "th_xbox360gamepad.h" 00035 00036 #include "main.h" 00037 #include "debug.h" 00038 00039 00040 const char *button_text[] = { "","LS","RS","XBOX","Unused","A","B","X","Y","DPAD UP","DPAD DOWN","DPAD LEFT","DPAG RIGHT","START","BACK","LEFT HAT","RIGHT HAT" }; 00041 00042 /* Define globals to hold Xbox360 stick data. */ 00043 XBOX360_STICK *stick; 00044 XBOX360_STICK stick_left_previous; 00045 XBOX360_STICK stick_right_previous; 00046 unsigned char trigger_left = 0, trigger_left_last = 0; 00047 unsigned char trigger_right = 0, trigger_right_last = 0; 00048 00049 void th_xbox360gamepad_init(void) { 00050 stick = xbox360gamepad_get_stick_left(); 00051 stick_left_previous.x = stick->x; 00052 stick_left_previous.y = stick->y; 00053 stick = xbox360gamepad_get_stick_right(); 00054 stick_right_previous.x = stick->x; 00055 stick_right_previous.y = stick->y; 00056 } 00057 00058 void th_xbox360gamepad(void) { 00059 unsigned char button; 00060 if ((button = xbox360gamepad_get_button()) != 0) { 00061 if (button > 0) { 00062 debug_printf("Button "); 00063 if (button > (BUTT_RIGHT_HAT_PRESS + 16)) { 00064 debug_printf("%s held\r\n", button_text[button - 32]); 00065 } 00066 else if (button > BUTT_RIGHT_HAT_PRESS) { 00067 debug_printf("%s released\r\n", button_text[button - 16]); 00068 } 00069 else { 00070 debug_printf("%s pressed\r\n", button_text[button]); 00071 switch (button) { 00072 case BUTT_A_PRESS: 00073 xbox360gamepad_led(LED_1_FLASH_THEN_ON); 00074 break; 00075 case BUTT_B_PRESS: 00076 xbox360gamepad_led(LED_2_FLASH_THEN_ON); 00077 break; 00078 case BUTT_X_PRESS: 00079 xbox360gamepad_led(LED_3_FLASH_THEN_ON); 00080 break; 00081 case BUTT_Y_PRESS: 00082 xbox360gamepad_led(LED_4_FLASH_THEN_ON); 00083 break; 00084 } 00085 } 00086 } 00087 } 00088 00089 if ((trigger_left = xbox360gamepad_get_trigger_left()) != trigger_left_last) { 00090 debug_printf("Left trigger: %d\r\n", trigger_left); 00091 trigger_left_last = trigger_left; 00092 } 00093 00094 if ((trigger_right = xbox360gamepad_get_trigger_right()) != trigger_right_last) { 00095 debug_printf("Right trigger: %d\r\n", trigger_right); 00096 trigger_right_last = trigger_right; 00097 } 00098 00099 unsigned char xbox360gamepad_get_trigger_right(void); 00100 00101 stick = xbox360gamepad_get_stick_left(); 00102 if (stick->x/STICK_DIVISOR != stick_left_previous.x/STICK_DIVISOR || stick->y/STICK_DIVISOR != stick_left_previous.y/STICK_DIVISOR) { 00103 stick_left_previous.x = stick->x; 00104 stick_left_previous.y = stick->y; 00105 // Don't bother printing for now, the sticks are too sensitive! 00106 int x = stick->x/STICK_DIVISOR, y = stick->y/STICK_DIVISOR; 00107 if (1 || (x > 10 || x < -10) || (y > 10 || y < -10) ) { 00108 debug_printf("New LEFT stick position x = %d y = %d\r\n", stick->x/STICK_DIVISOR, stick->y/STICK_DIVISOR); 00109 } 00110 } 00111 00112 stick = xbox360gamepad_get_stick_right(); 00113 if (stick->x/STICK_DIVISOR != stick_right_previous.x/STICK_DIVISOR || stick->y/STICK_DIVISOR != stick_right_previous.y/STICK_DIVISOR) { 00114 stick_right_previous.x = stick->x; 00115 stick_right_previous.y = stick->y; 00116 // Don't bother printing for now, the sticks are too sensitive! 00117 int x = stick->x/STICK_DIVISOR, y = stick->y/STICK_DIVISOR; 00118 if (1 || (x > 10 || x < -10) || (y > 10 || y < -10) ) { 00119 debug_printf("New RIGHT stick position x = %d y = %d\r\n", stick->x/STICK_DIVISOR, stick->y/STICK_DIVISOR); 00120 } 00121 } 00122 } 00123
Generated on Tue Jul 12 2022 18:05:35 by
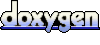