
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
ssp0.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "sowb.h" 00024 #include "debug.h" 00025 #include "ssp0.h" 00026 00027 bool ssp0_in_use; 00028 00029 bool SSP0_request(void) { 00030 if (!ssp0_in_use) { 00031 ssp0_in_use = true; 00032 return true; 00033 } 00034 return false; 00035 } 00036 00037 void SSP0_release(void) { 00038 ssp0_in_use = false; 00039 } 00040 00041 /* Declare ISR callbacks. */ 00042 extern int flash_read_ssp0_irq(void); 00043 extern int flash_write_ssp0_irq(void); 00044 00045 /* Function pointer type for the following table. */ 00046 typedef int (*SSP0_callback)(void); 00047 00048 /* Define an array of callbacks to make. */ 00049 const SSP0_callback ssp0_callbacks[] = { 00050 flash_read_ssp0_irq, 00051 flash_write_ssp0_irq, 00052 NULL 00053 }; 00054 00055 /** SSP0_IRQHandler 00056 */ 00057 extern "C" void SSP0_IRQHandler(void) __irq { 00058 for (int i = 0; ssp0_callbacks[i] != NULL; i++) { 00059 if ((ssp0_callbacks[i])() != 0) { 00060 break; 00061 } 00062 } 00063 } 00064 00065 /** SSP0_init 00066 */ 00067 void SSP0_init(void) { 00068 00069 DEBUG_INIT_START; 00070 00071 ssp0_in_use = false; 00072 00073 /* The flash device is connected to SSP1 via the Mbed pins. 00074 So this init is about configuring just the SSP1. */ 00075 00076 /* Enable the SSP1 peripheral. */ 00077 //LPC_SC->PCONP |= (1UL << 10); 00078 LPC_SC->PCONP |= (1UL << 21); 00079 00080 /* Select the clock required for SSP1. */ 00081 LPC_SC->PCLKSEL1 &= ~(3UL << 10); 00082 LPC_SC->PCLKSEL1 |= (3UL << 10); 00083 //LPC_SC->PCLKSEL0 &= ~(3UL << 20); 00084 //LPC_SC->PCLKSEL0 |= (3UL << 20); 00085 00086 /* Select the GPIO pins for the SSP0 functions. */ 00087 /* SCK0 */ 00088 LPC_PINCON->PINSEL0 &= ~(3UL << 30); 00089 LPC_PINCON->PINSEL0 |= (2UL << 30); 00090 /* MISO0 */ 00091 LPC_PINCON->PINSEL1 &= ~(3UL << 2); 00092 LPC_PINCON->PINSEL1 |= (2UL << 2); 00093 /* MISI0 */ 00094 LPC_PINCON->PINSEL1 &= ~(3UL << 4); 00095 LPC_PINCON->PINSEL1 |= (2UL << 4); 00096 00097 /* Select the GPIO pins for the SSP1 functions. */ 00098 /* SCK1 */ 00099 //LPC_PINCON->PINSEL0 &= ~(3UL << 14); 00100 //LPC_PINCON->PINSEL0 |= (2UL << 14); 00101 /* MISO1 */ 00102 //LPC_PINCON->PINSEL0 &= ~(3UL << 16); 00103 //LPC_PINCON->PINSEL0 |= (2UL << 16); 00104 /* MOSI1 */ 00105 //LPC_PINCON->PINSEL0 &= ~(3UL << 18); 00106 //LPC_PINCON->PINSEL0 |= (2UL << 18); 00107 00108 /* Note, we don't use SSEL1 in our design, we just use a standard GPIO 00109 because writing multi-byte data is simpler. */ 00110 00111 /* Setup the interrupt system. Note however, the SSP1 interrupt 00112 is only actually activated within the DMA ISR and is self disabling. */ 00113 NVIC_SetVector(SSP0_IRQn, (uint32_t)SSP0_IRQHandler); 00114 NVIC_EnableIRQ(SSP0_IRQn); 00115 00116 /* Setup the control registers for SSP1 */ 00117 LPC_SSP0->IMSC = 0; 00118 LPC_SSP0->CR0 = 0x7; 00119 LPC_SSP0->CPSR = FLASH_SSP_INIT_CPSR; 00120 LPC_SSP0->CR1 = 0x2; 00121 00122 DEBUG_INIT_END; 00123 } 00124
Generated on Tue Jul 12 2022 18:05:35 by
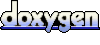