
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
predict_th.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "string.h" 00024 #include "sowb.h" 00025 #include "osd.h" 00026 #include "sgp4sdp4.h" 00027 #include "predict_th.h" 00028 #include "gps.h" 00029 #include "gpio.h" 00030 #include "satapi.h" 00031 #include "debug.h" 00032 00033 #ifdef PREDICT_TH_RUN 00034 00035 tle_t ISS_TLE; 00036 00037 00038 00039 int sgp4sdp4_th_init(void) { 00040 char buf[128]; 00041 GPS_TIME t; 00042 double jd_epoch, jd_utc, tsince, phase; 00043 vector_t vel = { 0, 0, 0 }; 00044 vector_t pos = { 0, 0, 0 }; 00045 vector_t obs_set; 00046 geodetic_t obs_geodetic; 00047 geodetic_t sat_geodetic; 00048 tle_t tle, localtle; 00049 char elements[3][80]; 00050 00051 /* Prepare to begin ISS */ 00052 strcpy(elements[0], "ISS (ZARYA)"); 00053 strcpy(elements[1], "1 25544U 98067A 10278.19511664 .00012217 00000-0 97221-4 0 147"); 00054 strcpy(elements[2], "2 25544 051.6473 027.7875 0007506 064.6316 006.5147 15.71651651680777"); 00055 00056 debug_printf("SGP4SDP4 TH starting 1\r\n"); 00057 00058 ClearFlag(ALL_FLAGS); 00059 Get_Next_Tle_Set(elements, &tle); 00060 memcpy(&localtle, &tle, sizeof(tle_t)); 00061 select_ephemeris(&tle); 00062 00063 gps_get_time(&t); 00064 00065 if (!t.is_valid) { 00066 debug_printf("SGP4SDP4 TH Abort, invalid time.\r\n"); 00067 return 0; 00068 } 00069 00070 jd_utc = gps_julian_date(&t); 00071 jd_epoch = Julian_Date_of_Epoch(tle.epoch); 00072 tsince = (jd_utc - jd_epoch) * xmnpda; 00073 00074 if (isFlagSet(DEEP_SPACE_EPHEM_FLAG)) { 00075 //debug_printf("Using SDP4\r\n"); 00076 SDP4(tsince, &tle, &pos, &vel, &phase); 00077 } 00078 else { 00079 //debug_printf("Using SGP4\r\n"); 00080 SGP4(tsince, &tle, &pos, &vel, &phase); 00081 } 00082 00083 Convert_Sat_State(&pos, &vel); 00084 //SgpMagnitude(&vel); // scalar magnitude, not brightness... 00085 //double velocity = vel.w; 00086 00087 GPS_LOCATION_AVERAGE loc; 00088 gps_get_location_average(&loc); 00089 if (loc.east_west == 'W') loc.longitude *= -1.; 00090 if (loc.north_south == 'S') loc.latitude *= -1.; 00091 00092 obs_geodetic.lat = loc.latitude * de2ra; // * 56.1920; 00093 obs_geodetic.lon = loc.longitude * de2ra; // * -3.0339; 00094 obs_geodetic.alt = loc.height / 1000.; 00095 00096 Calculate_Obs(jd_utc, &pos, &vel, &obs_geodetic, &obs_set); 00097 Calculate_LatLonAlt(jd_utc, &pos, &sat_geodetic); 00098 00099 double azimuth = Degrees(obs_set.x); 00100 double elevation = Degrees(obs_set.y); 00101 double range = obs_set.z; 00102 //double rangeRate = obs_set.w; 00103 //double height = sat_geodetic.alt; 00104 00105 //sprintf(buf, "JD UTC : %.5f JD SAT : %.5f DIF : %f\r\n", jd_utc, jd_epoch, jd_utc - jd_epoch); 00106 //debug_printf("%s", buf); 00107 00108 sprintf(buf, "ISS El:%.1f AZ:%.1f %dKm\r\n\n", elevation, azimuth, (int)range); 00109 osd_string_xy(0, 14, buf); 00110 debug_printf("%s", buf); 00111 return 1; 00112 00113 00114 } 00115 00116 #endif 00117
Generated on Tue Jul 12 2022 18:05:35 by
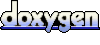